How to Convert String to List In Python?
How to Convert String to List In Python?
We all are familiar with what strings and lists are, let us have a quick revision on them-
Strings are a sequence of characters that are denoted using inverted commas ''. They are immutable which means they cannot be changed once declared. The distinction between the two strings can be understood using id().
Examples of a string are-
a=’Bill Gates’ x=’Moscow’
The list is a data structure in Python that can hold values of different data types. The values are enclosed in square brackets [ ]. Lists are mutable which means their values can be changed. An example of a list is-
list1 = [23,’Apple’,3.5,’Mangoes’]
In this article, we will discuss various ways of converting a string to a list in Python.
The following list contains the methods we will be elucidating in detail-
- Using the split function.
- Using list ().
- Converting float numbers into a list of lists.
- Using json module
- Using ast module
Using split function
The split function in Python is used to convert a string into a list. The parameters of this function are separator and maxsplit.
The separator tells us about the delimiter or the part at which the string should be split. By default, white space is considered a separator.
The maxsplit parameter tells us about the maximum number in which our string should be splitted.
Following program illustrates the working of split function-
sname="Tutorials and Examples" #declaring the string print(sname) #printing the string print(type(sname)) #printing the type of string print("The string after converting to list {}".format(sname.split())) #using split function
Input-
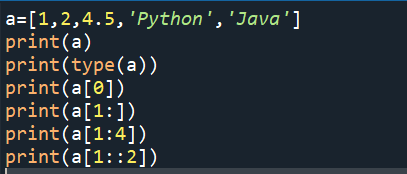
Output-
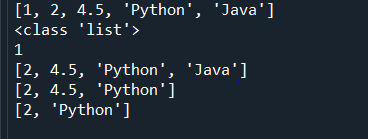
Once the program is executed we can see that the given string is converted into a list whose data type can be checked using the type function.
2. Using list()
The list() takes the specified string as a sequence and converts it into a list.
Following program illustrates the working of list()-
sname="Tutorials and Examples" #declaring the string print(sname) #printing the string print(type(sname)) #printing the type of string print("The string after converting to list of characters {}".format(list(sname)))
Input-
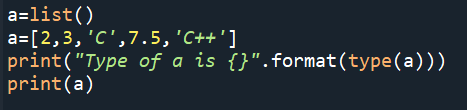
Output-

In the output we can observe after using list(), the string is converted to a list and each element in the list is the character of our string.
In the next example, we will pass float integers as a string and then apply list().
Let us have a look at the program-
sname="1.2 2.3 3.4 4.5 5.6" #declaring the string print(sname) #printing the string print(type(sname)) #printing the type of string print("The string after converting to list of float numbers {}".format(list(sname)))
Input-

Output-

In the output, we can see a list is returned whose each element is a number and a special character (.).
3. Converting float numbers into a list of lists.
In this example we will discuss how we can return a list of lists, we will use the split function and map() here.
We have seen how the split function works in the previous example, here will see how the map() adds its functionality to every element of our list and makes our program more meaningful.
It takes function and sequence as parameters.
The function that we have provided here is ‘list’ and the sequence is ‘slist’ which is the list obtained from the split function.
Following program illustrates how we can convert the string of float numbers into a list of lists.
sname="1.2 2.3 3.4 4.5 5.6" #declaring the string print(sname) #printing the string print(type(sname)) #printing the type of string slist=sname.split() print(slist) listc=list(map(list,slist)) print(listc)
Input-

Output-

In the output, we can observe a list that has lists of numbers.
4. Using json module
There is a module in Python called 'json' which can be used to work with the json data (JSON stands for JavaScript Object Notation). It takes a valid string and converts it into a dictionary. Here we will be using json.loads() to convert our string to a list.
The important thing that we must take care of is that our input should be numeric.
Following program illustrates how we can convert the string to lists by importing the json module.
import json sname="[1,2,3,4,5]" #declaring the string print(sname) #printing the string print(type(sname)) #printing the type of string slist=json.loads(sname) print(slist) print(type(slist))
Input-
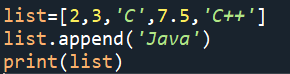
Output-

In the output, we can observe it returns the type of sname as a string and then after applying json.loads() it converts our input string to a list which can be verified using the type function.
4. Using ast module
AST stands for abstract syntax trees. This module is used to check what the current grammar looks like concerning our program.
Here ast.literal_eval() takes a string as an input and converts it to a list.
Following program illustrates how we can convert the string to lists by importing the ast module.
import ast sname="[1,2,3,4,5]" #declaring the string print(sname) #printing the string print(type(sname)) #printing the type of string slist=ast.literal_eval(sname) print(slist) print(type(slist))
Input-

Output-

In the output, we can observe it returns the type of sname as a string, and then after applying ast.literal_eval() it converts our input string to a list which can be verified using the type function.
So, in this article, we discussed the various ways of converting a string to a list in Python.