Python Bubble Sort
Bubble sort is one of the techniques used to sort the elements in lists in a certain order, either in ascending or descending order. It is called Bubble sort because lower values get bubbled up to the front while the larger values sink into the last and stay there.
In this article, we will explain the Bubble sort algorithm and the mechanism with the program using Python.
Bubble Sort Algorithm
Bubble sort algorithm simply compares each pair of adjacent items and, if they are in the wrong order, swaps them, and this process continues until the end of the list till all the elements in the list are in order.
Example
Let’s understand it with an example to make things clear:
Assume we need the given list in ascending order: applying bubble sort, the mechanism would be like:
List1 = [7, 2, 8, 5, 4]
7 |
2 |
8 |
5 |
4 |
Firstly, the first two elements are checked. 7 is greater than 2, so they have to be swapped, which becomes:
2 |
7 |
8 |
5 |
4 |
Now, the second and third elements are compared. 7 and 8 are in the correct order. So, the next two elements are checked. 8 and 5 are not in the correct order, so swap.
2 |
7 |
5 |
8 |
4 |
Now, the last two elements are checked. Not in the order, so swap.
2 |
7 |
5 |
4 |
8 |
Here is the result after the first iteration. Now, the size for the next iterations will be lessened by 1 as the highest elements already got their spot. The highest element is bubbled up at the end. Now, again the same process is repeated.
The first two elements are checked. 2 and 7 are correct. So, the next two elements are checked. 7 and 5 are not in the right order, so swap.
2 |
5 |
7 |
4 |
8 |
The last check, 7 and 4, is compared and then swapped.
2 |
5 |
4 |
7 |
8 |
The second iteration is also done now. Again the size for the iteration is decreased by 1, and the third iteration is started.
2 |
5 |
4 |
7 |
8 |
The first two elements are checked. 2 and 5 are correct, so the next two elements are checked. 5 and 4 are not in order, so SWAP.
2 |
4 |
5 |
7 |
8 |
Now, in the last iteration, the first two elements are checked, and SORTING is done as they are in the correct order.
2 |
4 |
5 |
7 |
8 |
Here is the whole process all in the same picture:
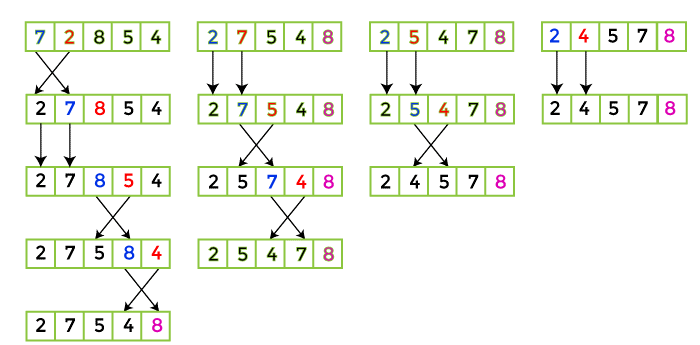
Algorithm
- From index 0, compare the elements with the succeeding element in the list.
- If the element you're working on is greater than the succeeding element, swap the two elements.
- Else, if both elements are in the correct order, then go to the next element of the succeeding element and the succeeding element and repeat step number 1.
The whole process is limited to sorting the elements into ascending order. We can do it in descending order too. The only change will be in the comparison. We check if the element we're working on is less than the next element, and if it is; we leave it and go to the next elements, while if they are not, we swap the elements. It bubbles up the smallest element. But, all the time, mostly ascending order is used and preferred.
Python Program to implement Bubble Sort
Here is the Python program to implement bubble sort on a list
def bubbleSort (list1): n = len (list1) for i in range(n-1): for j in range(0, n-i-1): if list1[j] > list1 [j + 1] : list1 [j], list1 [j + 1] = list1 [j + 1], list1 [j] list1 = [7, 2, 8, 5, 4] bubbleSort (list1) print ("Sorted list is:") for i in range (len (list1)): print ("% d" % list1 [i], end=" ")
Output:
Sorted list is: 2 4 5 7 8
Explanation:
In the above program, we wrote a function “bubbleSort” that takes the list as the parameter and in the function; we took the length of the list as the variable n. Now, we used nested loops. In the first loop, we traverse through the loop and in the second loop; we traverse through the loop till the last but one element. Now, we check if the elements are in the correct order and then swap if not.
Here is the mechanism table:
First, the list looks like [7, 2, 8, 5, 4]
i |
j |
j+1 |
if (list1[j] > list1 [j+1]) |
Swap, then list1: |
0 |
0 |
1 |
7 > 2 yes so swap |
[2, 7, 8, 5, 4] |
1 |
2 |
7 > 8 no |
[2, 7, 8, 5, 4] |
|
2 |
3 |
8 > 5 yes so swap |
[2, 7, 5, 8, 4] |
|
3 |
4 |
8 > 4 yes so swap |
[2, 7, 5, 4, 8] |
|
|
||||
1 |
0 |
1 |
2 > 7 no |
[2, 7, 5, 4, 8] |
1 |
2 |
7 > 5 so swap |
[2, 5, 7, 4, 8] |
|
2 |
3 |
7 > 4 so swap |
[2, 5, 4, 7, 8] |
|
|
||||
2 |
0 |
1 |
2 > 5 no |
[2, 5, 4, 7, 8] |
1 |
2 |
5 > 4 so swap |
[2, 4, 5, 7, 8] |
|
|
||||
3 |
0 |
1 |
2 > 4 no |
[2, 4, 5, 7, 8] |
|
|
|
|
Advantages
Bubble sort is really the simplest algorithm out of all the algorithms used for sorting. Also, this mechanism has a lot of advantages, which are given below:
- It is simple to understand, write and takes less number of lines.
- All the data is stored in one place and there is little memory overhead. Moreover, after the procedure is done, the data in the memory will be ready to be processed.
Disadvantages
Disadvantages include the time. It needs to check every pair of adjacent items for the size of elements in the array for several number of times, which takes more time. As the number of elements in the list increases, the amount of time required to implement bubble sort on the list increases exponentially. Ten times the size of array makes the time required hype up to almost 100 times.