How to Take Input in Python
How To Take Input In Python
Python has various in-built functions that help in code redundancy. Let's discuss the usage of some functions-
- ascii() – it returns a readable representation of objects.
- format() – it inserts the specified value in the placeholder.
- globals() – it returns a dictionary of variables present in the global namespace.
- help() – it displays the documentation of modules.
- id() – it tells about the data type of variable.
- map() – it returns a map object of results after applying the function.
- filter() – it returns the filtered objects after testing whether items are acceptable or not.
- pow() – this function returns of value x raised to the power of y.
- sorted() – this function returns a sorted list of objects.
- input() – this function asks the user to input the value.
Here we will talk about the ways by which we can take input in python.
The two approaches we can use are-
- Using a prompt in the input function.
- Using the input function input().
In this program, we ask the user to input the age and then we will display it.
a=input(“Enter age: ”) print(“The age is {}”.format(a))
Once you have written the code, go to ‘File’ and click on ‘Save As’. Save your file as ‘input.py’.
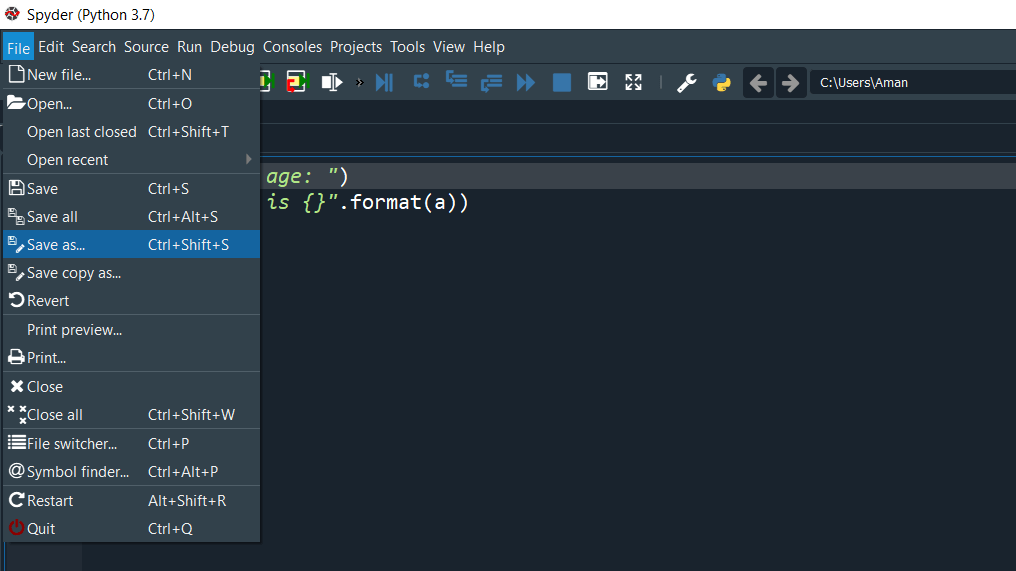
When we give input in the output window, the input function takes that as a string (it doesn't matter whether you give an integer or float value). If we want to use an integer value for computation in our code, we must typecast it. Here we can check the data type of our variable (in python we refer to them as objects) using print(type(a)).
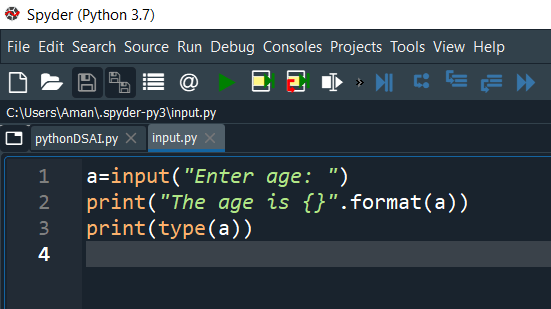
On executing our program, we can see that it belongs to the class 'string'.

To obtain our value in the integer type, we will typecast it as-
a=int(input(“Enter age: ”))
In this program, we have used placeholders { } in the print function. It holds the values that we want to display. The sequence of the same can be provided in the format function.
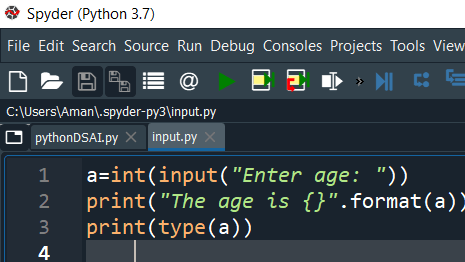
Now on running our program, we can see this time the class type is ‘integer’.
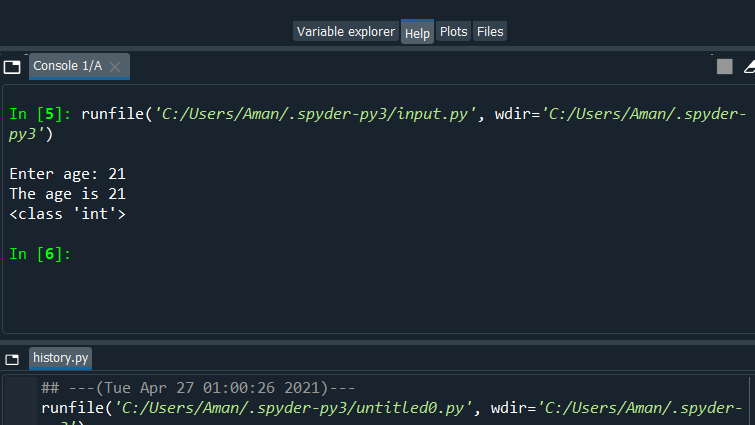
In the next approach, we will directly call the input function and ask the user to provide the value.

On executing this program, we will not get a message on the output window, Instead, we will see the blinking cursor on the output window so here we’ll input the value.
Here also we can observe the type is a string.

We can again typecast it and get our value in the integer type.
Input_age=int(input())
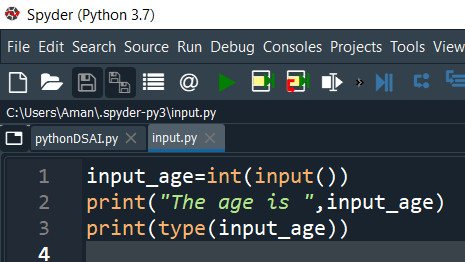
After typecasting we can observe that we can get the value in the 'integer' type.
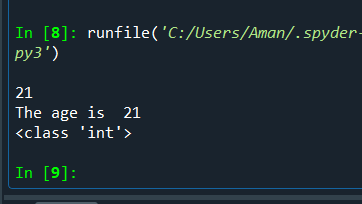
Let’s have a look at few more examples where input() is used.
p=int(input("Enter the principal: ")) r=int(input("Enter the rate: ")) t=int(input("Enter the time: ")) si=p*r*t/100 print("The value for simple interest is {}".format(si))
- Python program to calculate the volume of a cylinder.
pi=3.14 r=int(input("Enter the value of radius: ")) h=int(input("Enter the value of height: ")) vol=pi*pow(r,2)*h print("Volume of cylinder is {}".format(vol))
- Python program to perform addition, subtraction, division, and multiplication.
num1=float(input("Enter number1: ")) num2=float(input("Enter number2: ")) add=num1+num2 subt=num1-num2 mult=num1*num2 div=num1/num2 print("Addition of two float numbers is {}".format(add)) print("Subtraction of two float numbers is {}".format(subt)) print("Multiplication of two float numbers is {}".format(mult)) print("Division of two float numbers is {}".format(div))
- Python program to calculate the area of a circle.
pi=3.14 r=int(input("Enter the value of radius: ")) area=pi*r*r print("Area of circle is {}".format(area))
- Python program to find the greatest of three numbers.
a=int(input("Enter the value of 1st number: ")) b=int(input("Enter the value of 2nd number: ")) c=int(input("Enter the value of 3rd number: ")) if(a>b and a>c): print("{} is greatest".format(a)) elif(b>a and b>c): print("{} is greatest".format(b)) else: print("{} is greatest".format(c))
- Python program to understand type conversion.
x=input("Enter the first number: ") y=input("Enter the second number: ") print(x+y) #result is addition of two strings x=int(input("Enter the first number: ")) y=int(input("Enter the second number: ")) print(x+y) #result is addition of two numbers
- Python program to calculate distance between two points.
x1=int(input("Enter the x-coordinate of first point: ")) y1=int(input("Enter the y-coordinate of first point: ")) x2=int(input("Enter the x-coordinate of second point: ")) y2=int(input("Enter the x-coordinate of second point: ")) dist=((x2-x1)**2+(y2-y1)**2)**0.5 print("The calculated distance is {}".format(dist))