How to develop a game in python
Fun always makes a task interesting and easy to execute. Learning in the same theoretical way at some point reaches the boring spot. In this article, using some Python knowledge, let us build a game – remember the game we played as children on our little Nokia cell phones?
Let us now build the “Hungry snake game”.
Python is a very user-friendly language. Its library provides many inbuilt functions and modules to make every complex task easy and approachable for beginners. Then, does it help in creating games? Of course, yes!
There is an inbuilt library in Python called the pygame library. It is a set of modules. Just as the name suggests, it is used to develop games. It provides great help to the designers with suitable graphics, animations, and even sounds.
Pete Shinners developed this cross-platform set of modules.
It is no big deal to learn about the module. It would be best if you had a basic understanding of Python language and its concepts. Let’s start by installing the module:
Installation
To use the module, we need to install it first, and for that, all you need to do is to type a command in the cmd terminal; follow the below steps:
1. Open cmd from the start menu
2. Type the command:
pip install pygame
3. Open the anaconda terminal prompt and type the same command if you are using anaconda.
Creating a simple pygame window:
We cannot play the game in the output terminal like executing a program. We need to create a separate window using pygame to play the game.
Program:
import pygame
pygame. init ()
scr = pygame. display. set_mode ((500, 600))
flag = False
while flag != True:
for event in pygame. event. get ():
if event. type == pygame. QUIT:
flag = True
pygame. display. flip ()
Output: A 500 * 600 (Width * Height) pygame empty window will be created.
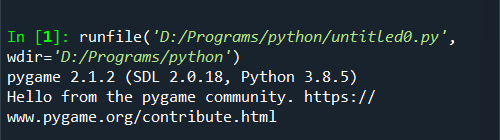
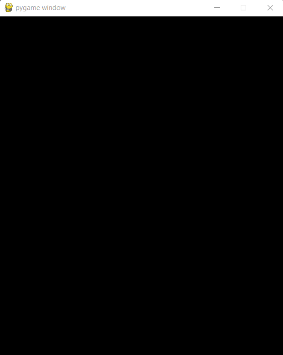
Understanding:
- pygame. init (): init () stands for initializing. It is the same as in the concept of classes and objects in Python. Here, it is used to initialize the modules in pygame.
- pygame. display. set_mode ((width, height)): It gives dimensions to the pygame window to be created.
- pygame. event. get (): It is used to get or obtain the event/ action performed and do the tasks accordingly, like when the user hits the close button.
- pygame. QUIT: When the user clicks the close button of the pygame window, this function closes the window by quitting the program execution.
- flip (): This function applies any update to the whole program/ game.
These are the functions we used from pygame to create a window. All we did in the program is:
We imported the pygame; we gave the dimensions to the window. We took a flag variable and initialized it to False. Now, we ran a loop with the user's events/ actions, which we get by the get () function. When the user clicks close, the flag is set to True, and the loop is broken; the window will be closed.
Graphics/ designs:
In the module, many pre-defined functions help us create designs and draw shapes on the screen.
First, let us go through the functions and their syntaxes:
The module used to draw geometrical shapes is the draw module:
We can use different constructors to draw these shapes with different arguments. In the below program, we are going to use:
1. To draw a rectangle:
pygame. draw. rect (surface, color, dimensions, fill)
- For color, we use the color codes.
- If we give fill == 0: fill the rectangle with a color
fill > 0: for line thickness
fill < 0: nothing will be drawn
2. To draw a line:
pygame. draw. line(surface, color, initial_pos, final_pos, width)
- width represents the thickness of the line
- If width >= 1 : line thickness is grown
- Default width = 1
- If width < 1, nothing will be drawn
3. To draw multiple lines continuously:
pygame. draw. lines (surface, color, closed? width)
- This function is used to draw multiple lines with the given coordinates
- Closed?: if it is given True, an additional line segment will be drawn to close the drawn lines into a closed figure.
4. To draw an ellipse:
pygame. draw. ellipse (surface, color, dimensions, fill)
- Dimensions of the rectangle should be given by which the ellipse will be bounded.
- If we give fill == 0: fills the ellipse with a color
fill > 0: for line thickness
fill < 0: nothing will be drawn
5. To draw a polygon:
pygame. draw. polygon (surface, color, points, fill)
6. To draw an arc:
pygame. draw. arc (surface, color, intial_angle, stop_angle, width)
7. To draw a circle:
pygame. draw. circle (surface, color, center_point, radius)
Example:
Here is a sample program using the above functions:
import pygame
from math import pi
pygame. init ()
size = [500, 600]
window = pygame.display.set_mode(size)
flag = False
seconds = pygame. time. Clock()
while not flag:
seconds. tick(10)
for event in pygame. event. get():
if event. type == pygame. QUIT:
flag = True
window. fill ((255, 255, 255))
pygame. draw. line (window, (255, 255, 0), [0, 0], [50,40], 5)
pygame. draw. lines (window, (0, 255, 0), False, [[0, 70], [40, 70], [150, 80], [220, 30]], 5)
pygame. draw. rect (window, (0, 255, 0), [65, 17, 54, 22], 3)
pygame. draw. rect (window, (0, 255, 0), [190, 15, 58, 30])
pygame. draw. ellipse (window, (255, 0, 0), [245, 15, 30, 40], 2)
pygame. draw. ellipse (window, (255, 0, 0), [350, 10, 30, 20])
pygame. draw. polygon (window, (0, 0, 0), [[300, 100], [0, 200], [200, 200]], 5)
pygame. draw. circle (window, (0, 255, 255), [70, 250], 40)
pygame. draw. arc (window, (0, 255, 0), [220, 85, 150, 125], 0, pi / 2, 2)
pygame. display. flip ()
pygame. quit ()
Understanding:
We imported pygame and pi from the math module. We used pi to specify the angle while drawing a circle. We created a window. We used:
- pygame. time. Clock. tick (): time is a module used to keep track of time, and clock is an object. The tick function helps to compute the number of milliseconds passed after the previous call. We passed an argument – 10. The function will delay or slow the program than 10 frames per second, thus controlling the speed.
After that, we used the fill function to give the color code of white to fill the window with white color. We used the functions from the draw module to draw different shapes, from lines to circles.
Output:
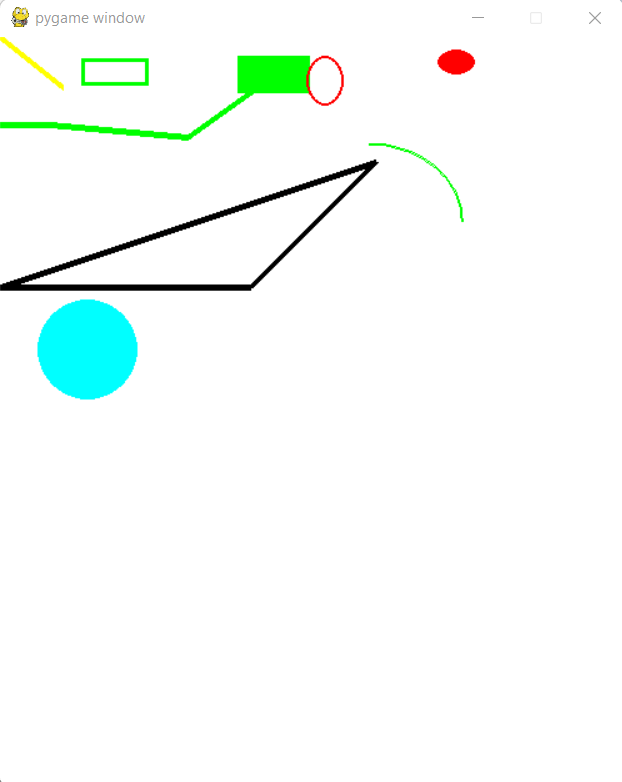
Program to develop Snake Game
Now, it is time to jump into the concept, program for the snake game:
import random
import pygame
import Tkinter as tk
from Tkinter import messagebox
class cube (object):
rows = 20
w = 500
def __init__ (self, start, x_dir = 1, y_dir = 0, color = (255, 0, 0)):
self. pos = start
self. x_dir = 1
self. y_dir = 0
self. color = color
def movement (self, x_dir, y_dir):
self. x_dir = x_dir
self. y_dir = y_dir
self. pos = (self. pos[0] + self. x_dir, self. pos[1] + self. y_dir)
def draw (self, surface, flag = False):
dis = self. w // self. rows
i = self. pos [0]
j = self. pos [1]
pygame. draw. rect (surface, self. color, (i * dis + 1, j * dis + 1, dis - 2, dis - 2))
if flag:
centre = dis // 2
radius = 3
cirmid = (i * dis + centre - radius, j * dis + 8)
cirmid2 = (i * dis + dis - radius * 2, j * dis + 8)
pygame. draw. circle (surface, (0, 0, 0), cirmid, radius)
pygame. draw. circle (surface, (0, 0, 0), cirmid2, radius)
class snake (object):
body = []
turns = {}
def __init__ (self, color, pos):
self. color = color
self. head = cube (pos)
self. body. append (self. head)
self. x_dir = 0
self. y_dir = 1
def movement (self):
for event in pygame. event. get ():
if event. type == pygame. QUIT:
pygame. quit ()
clicks = pygame. key. get_pressed ()
for click in clicks:
if clicks [pygame. K_LEFT]:
self. x_dir = -1
self. y_dir = 0
self. turns [self. head. pos [:]] = [self. x_dir, self. y_dir]
elif clicks [pygame. K_RIGHT]:
self. x_dir = 1
self. y_dir = 0
self. turns [self. head. pos [:]] = [self. x_dir, self. y_dir]
elif clicks [pygame. K_UP]:
self. x_dir = 0
self. y_dir = -1
self. turns [self. head. pos [:]] = [self. x_dir, self. y_dir]
elif clicks [pygame. K_DOWN]:
self. x_dir = 0
self. y_dir = 1
self. turns [self. head. pos [:]] = [self. x_dir, self. y_dir]
# When snake hits the boundary wall
for i, j in enumerate (self. body):
position = j. pos [:]
if position in self. turns:
turn = self. turns [position]
j. movement (turn [0], turn [1])
if i == len (self. body) - 1:
self. turns. pop (position)
else:
if j. x_dir == -1 and j. pos [0] <= 0:
j. pos = (j. rows - 1, j. pos [1])
elif j. x_dir == 1 and j. pos [0] >= j. rows - 1:
j. pos = (0, j. pos [1])
elif j. y_dir == 1 and j. pos [1] >= j. rows - 1:
j. pos = (j. pos [0], 0)
elif j. y_dir == -1 and j. pos [1] <= 0:
j. pos = (j. pos [0], j. rows - 1)
else:
j. movement(j. x_dir, j. y_dir)
def reset (self, pos):
self. head = cube (pos)
self. body = []
self. body. append (self. head)
self. turns = {}
self. x_dir = 0
self. y_dir = 1
#To add a cube after eating
def pluscube (self):
snaketail = self. body [-1]
x, y= snaketail. x_dir, snaketail. y_dir
if x == 1 and y== 0:
self. body. append (cube ((snaketail. pos [0] - 1, snaketail. pos [1])))
elif x == -1 and y == 0:
self. body. append (cube ((snaketail. pos [0] + 1, snaketail. pos [1])))
elif x == 0 and y== 1:
self. body. append (cube ((snaketail. pos [0], snaketail. pos [1] - 1)))
elif x == 0 and y== -1:
self. body. append (cube ((snaketail. pos [0], snaketail. pos [1] + 1)))
self. body [-1]. x_dir = x
self. body [-1]. y_dir = y
def draw (self, surface):
for i, j in enumerate (self. body):
if i == 0:
j. draw (surface, True)
else:
j. draw (surface)
def Grid (w, rows, surface):
sizebetween = w // rows
x = 0
y = 0
for i in range (rows):
x = x + sizebetween
y = y + sizebetween
# draw grid line
pygame. draw. line (surface, (255, 255, 255), (x, 0), (x, w))
pygame. draw. line (surface, (255, 255, 255), (0, y), (w, y))
def Redraw (surface):
global rows, width, s, snack
surface. fill ((0, 0, 0))
s. draw (surface)
snack. draw (surface)
Grid (width, rows, surface)
pygame. display. update ()
def randsnack (rows, item):
positions = item. body
while True:
x = random. randrange (rows)
y = random. randrange (rows)
if len (list (filter (lambda z: z. pos == (x, y), positions))) > 0:
continue
else:
break
return (x, y)
def screen_message (subject, content):
root = tk. Tk ()
root. Attributes ("-topmost", True)
root. withdraw ()
messagebox. showinfo (subject, content)
try:
root. destroy ()
except:
pass
def main ():
global width, rows, s, snack
width = 500
rows = 20
win = pygame. display. set_mode ((width, width))
s = snake ((255, 0, 0), (10, 10))
snack = cube (randsnack (rows, s), color = (0, 255, 0))
flag = True
clock = pygame. time. Clock ()
while flag:
pygame. time. delay (50)
clock. tick (10)
s. movement ()
if s. body[0]. pos == snack. pos:
s. pluscube ()
snack = cube (randsnack (rows, s), color = (0, 255, 0))
for x in range (len (s. body)):
if s. body [x]. pos in list (map (lambda z: z. pos, s. body [x + 1 :])):
print ('Your score: \n', len (s. body))
screen_message ('Game over! \n', 'Play again. . . \n')
s. reset ((10, 10))
break
Redraw (win)
main ()
Output:
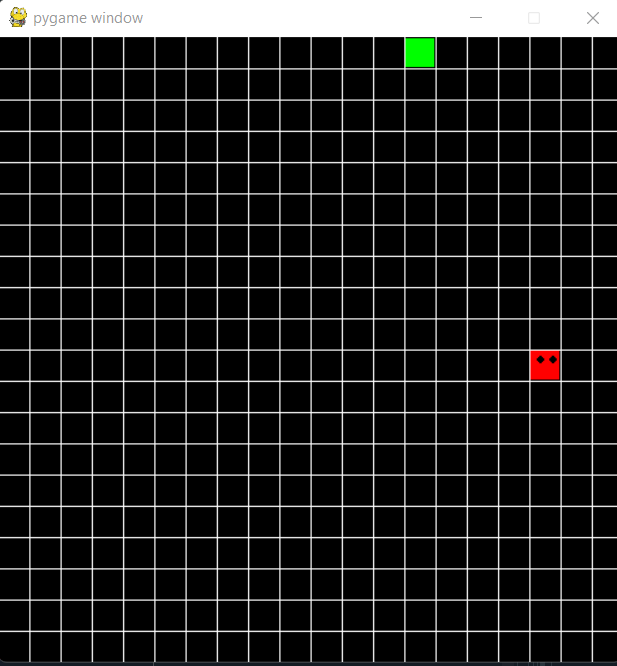
If the snake runs into its body, the game will be over, and the above message box will be displayed. We used Tkinter to make the dialog box, and all the other functions are from pygame.
You can refer to the official website to know more about pygame and its functions.