Character Set in Python
A collection of legal characters that will recognize by a programming language known as a Character set.
Taking python programming language as an instance. The set of characters supported by the python language is called the python character set. Therefore, the Python character set is a genuine collection of characters that the Python language can recognize.
Python is compatible with all ASCII and Unicode characters. Python character set includes the following:
- Digits: Python programming language supports all the digits starting from 0 to 9. Supports all digits (0 to 9)
- Alphabets: Python programming supports all alphabets, including small and capital alphabets. Supports all small alphabets (a to z) and all Capital alphabets (A to Z).
- White spaces: Python programming language is completely depended on indentation where it is a tab space with 4 white spaces. Python recognizes white spaces like tab spaces (4 white spaces). Blank space, newline character and carriage return.
- ASCII values: Python recognizes all the ASCII characters. Thus, python character set also includes ASCII values.
- UNICODE characters: Almost all characters are now available across platforms, programmes, and devices due to Unicode, an international character encoding standard that assigns a unique number to each character across different programming languages. Python also has support for UNICODE characters.
Since Python supports the Unicode standard, and it also supports ASCII and Unicode.
You can better understand how the python character set works by understanding the python tokens.
What is a Token
The smallest distinct unit in a Python programming language is called a token. Tokens are used to create all statements and instructions in a program.
We have various tokens in Python that are:
- Keywords
- Identifiers
- Python Literals
- Operators
- Punctuator
These python tokens are built on top of a python character set.
Keywords: In a computer language, keywords have a particular significance or meaning. They are utilized for their unique qualities. They cannot be utilized for any arbitrary reason, including as names for functions or variables.
In Python, we have 33 keywords. That include:
False, await, else, impart, pass, None, break, except, in, raise, True, class, finally, is, return, and, continue, for, lambda, try, as, def, from, nonlocal, while, assert, del, global, not, with, async, elif, if, or, yield.
Example
# For is keyword
for p in range(1, 9):
# Printing the value of p after each iteration of for loop
print(p)
# Checking if the value of p is less than 5
# Here we have used if, continue, else, and break keywords in this program
if p < 5:
continue
# If p is greater than 5, then break the loop
else:
break
Output
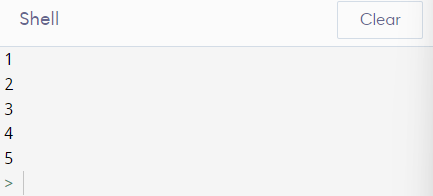
Identifiers: The names assigned to any variable, function, class, list, method, etc. for their identification are known as identifiers. Python has certain guidelines for naming identifiers and is a case-sensitive language.
You have certain rules for naming an identifier in the python programming language.
As Python is a case-sensitive programming language. It even treats small and capital letters as different identifiers. "Key" and "key" are two different identifiers in python programming.
Every identifier should:
- Start with an alphabet. It might either be a small letter (a – z) or a capital letter (A – Z), or it can start with an underscore (_). But it should start with any other character other than these.
- Digits can also be a part of an identifier but digits can not start an identifier name. That identifier should not begin with a digit.
- Except for alphabets, underscore, digits using any other character or white spaces are not allowed in an identifier name.
- It should not be a keyword.
Example
# Declaring Abc, _abc and abc1 as identifiers in the program
Abc = 1
_abc = 2
Abc1 = 3
# Printing the values using the identifiers
print (Abc)
print (_abc)
print (Abc1)
Output
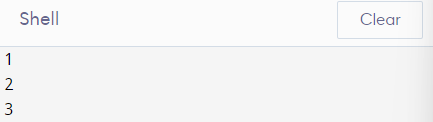
Using the identifier names 9abc, ab@c, and 0@bc is not allowed in Python as you have to follow the rules for declaring an identifier. Above mentioned identifiers do not follow the set of rules, so we cannot use them for naming an identifier.
Python Literals: Literals are the constant values that are fixed. The source code uses literal in Python.
In a python programming language, you have support for a different type of literal, which include:
- String literals
- Character literals
- Numeric literals
- Boolean literals
- Special literals
- Collection of literals
String Literals: String literal is defined as a text which is enclosed within single quotes (‘ ’), Double quotes (“ ”) or triple quotes. The such text represents the python string literals. We use triple quotes for multi-line strings.
Example
# Program for string literals
p = ‘Hai’
q = “hello”
r = ‘‘‘this is
multi line
string’’’
# Printing the values
print (p)
print (q)
print (r)
Output
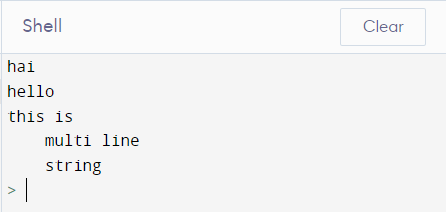
Character literals: A character literal is a single character enclosed between single quotes or Double quotes.
Example
# Program for Character literals
p = ‘a’
q = “b”
# Printing character literals
print (p)
print (q)
Output
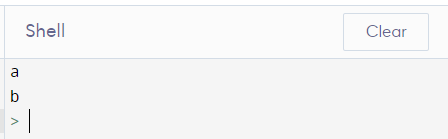
Numeric literals: Literals in the form of numbers are known as Python numeric literals. Python is compatible with the following numeric literals:
- Integer literals
- Float literals
- Complex literals
Example
# Program for numeric literals in Python
p = 1 # Integer literal
q = -2
r = 0.1 # Float literal
# Printing the values
print (p)
print (q)
print (r)
Output
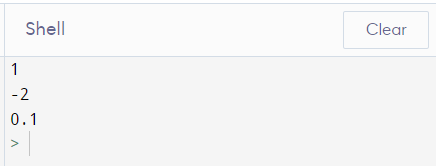
Boolean literals: In Python, Boolean literals are True or False values. Where T and F are capital letters, you should not use small letters as Python is case sensitive language.
Example
# Program for Python Boolean literals
p = True
q = False
# Printing values
print (p)
print (q)
Output
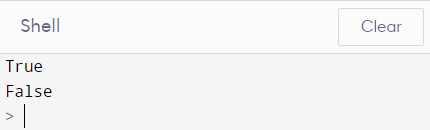
Special literals: None is said to be special literal in a python programming language. We use None when you have to denote nothing that is no value, or the absence of value.
Example
# Program for special literal in Python
p = None
# Printing the value
print (p)
Output
None
Collection of literals: In Python, you have a collection of literals, which is also known as literals collections that are:
1) List
Python uses lists to store the sequential order of different kinds of data. Although Python has six data types that may hold sequences, the list is the most popular and dependable form. Because Python lists are changeable types, we can change their elements after they've been generated.
2) Tuple
A tuple is an assortment of immutable, ordered objects. Since both tuples and lists are sequences, they are similar. Tuples and lists, however, vary because we cannot alter tuples, although we can modify lists after creating them. Additionally, we build tuples using parentheses, whereas we make lists using square brackets.
3) Dictionary
The information is kept in key-value pair format using a Python dictionary. Python's dictionary data type can mimic real-world data arrangements where a given value exists for a particular key. The mutable data structure is what it is. The element's Keys and values are used to define the dictionary.
4) Set
A Python set is a grouping of unsorted elements. The set's elements must all be distinct and unchangeable, and the sets must eliminate duplicates. Sets can be changed after they are created because they are changeable.
Example
# Program for literals collection
l = [1, 2, 3] # List
t = (1, 2, 3) # Tuple
d = {1: ‘a’, 2: ‘b’, 3: ‘c’} #Dictionary
s = {1, 2, 3} #Set
# Printing the values
print (l)
print (t)
print (d)
print (s)
Output
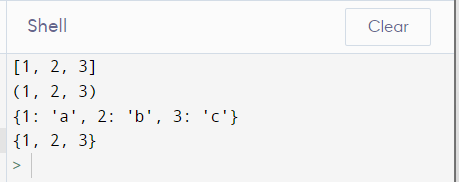
Operators: Python Operators are symbols used to perform operations on operands that may be values or identifiers. Operators are used to performing operations on operands.
In the python character set, we have special characters used as operators.
In Python, we have different types of operators that are:
- Arithmetic Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Identity Operators
- Membership Operators
Example
# Program for python operators
p = 10
q = 20
# Arithmetic Operator in Python
print (p + q)
# Comparison Operator in Python
print (p == q)
o = True
u = False
# Logical Operator in Python
print (o or u)
# Bitwise Operator in Python
print (p | q)
# Assignment Operator in Python
p += q
print (p)
# Identity Operator in Python
print (p is q)
l = [10, 20, 30, 40, 50]
# Membership Operator in Python
if (q in l):
Print ("q is present in the list l")
Output
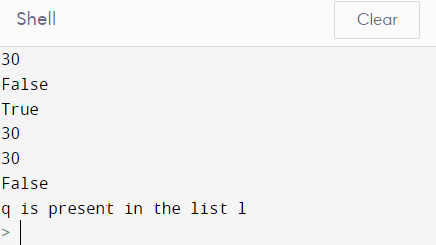
Punctuators: Punctuators are the symbols that are used in Python to form the structures, Organize statements and expressions.
Some of the punctuators in a python programming language are:
[], {}, (), @, -+, +=, *=, //=, **== and there are even many other punctuators in python programming language which you can use to organize the statements.