How to Compare two Lists in Python?
How to Compare two Lists in Python
The list is a data structure in Python that can hold values of different data types. The values are enclosed in square brackets [ ]. Lists are mutable which means their values can be changed. An example of a list is-
list1 = [23,’Apple’,3.5,’Mangoes’]
The values present in a list can be accessed through indexing. For instance, list1[1] will return the value ‘Apple’.
The difference between lists and arrays is that lists can hold values of multiple data types whereas arrays hold values of a similar data type.
In this article, we will discuss how to compare two lists in python using the following methods-
- Sort()
- Collections counter
- Using equals operator.
In all the programs, we are using the approach of defining the functions, since they reduce the code size and help us to use the desired functionality for different input parameters.
In each program, the definition of the function contains sort(), collections counter(), and use of equals operator, and then we have passed two lists at a time as an input parameter.
Let us have a look at the first method where we will compare the elements of a list using sort().
In sort() method, we will take lists as input parameters and then apply the sort() method which will arrange the elements in ascending order and then compare its elements
The following program illustrates the same-
#creating a function def comp_list(l1,l2): l1.sort() #using sort() l2.sort() if(l1==l2): print("Both lists are equal") else: print("Both lists are not equal") l1=[1,2,3,4,5] l2=[2,4,5,1,3] comp_list(l1, l2) #calling the function l3=[11,12,13,14,15] l4=[15,11,13,12,14] comp_list(l3, l4) #calling the function l5=[20,21,22,23,24] l6=[27,28,29,30,31] comp_list(l5,l6) #calling the function
INPUT-
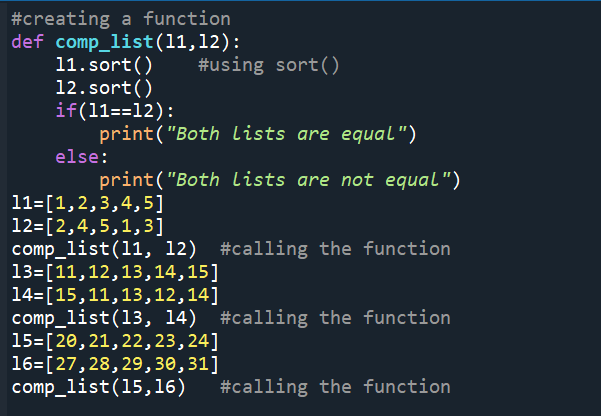
OUTPUT-
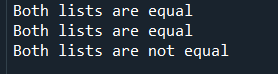
We can observe the following things-
- First, the two lists are passed l1 and l2 in the function comp_list(), and then the results are checked on the execution of our code.
- Similarly, we specified the elements in the lists l3,l4,l5, and l6 and then passed l3 and l4 in the function and then l5 and l6.
- In the output, we can observe that the elements of l1 and l2, l3 and l4 are equal whereas elements of l5 and l6 are not equal.
In the second method, we will implement it using collections.counter()
The counter() method returns the count of objects(iterables) present in the list. To use it for comparison we will import collections and then use this method.
The following program illustrates the same-
import collections #creating a function def comp_list(l1,l2): if(collections.Counter(l1)==collections.Counter(l2)): print("Both lists are equal") else: print("Both lists are not equal") l1=[1,2,3,4,5] l2=[2,4,5,1,3] comp_list(l1, l2) #calling the function l3=[11,12,13,14,15] l4=[15,11,13,12,14] comp_list(l3, l4) #calling the function l5=[20,21,22,23,24] l6=[27,28,29,30,31] comp_list(l5,l6) #calling the function
INPUT-

OUTPUT-

We can observe the following things-
- First, the two lists are passed l1 and l2 in the function comp_list(), and then the results are checked on the execution of our code.
- Similarly, we specified the elements in the lists l3,l4,l5, and l6 and then passed l3 and l4 in the function and then l5 and l6.
- In the output, we can observe that the elements of l1 and l2, l3 and l4 are equal whereas elements of l5 and l6 are not equal.
The last method which we will discuss is the comparison of the elements of the list using the equals operator.
The == operator is used to test the equality of two objects. It returns the result as True or False.
The following program illustrates the same-
#creating a function def comp_list(l1,l2): if(l1==l2): print("Both lists are equal") else: print("Both lists are not equal") l1=[1,2,3,4,5] l2=[2,4,5,1,3] comp_list(l1, l2) #calling the function l3=[11,12,13,14,15] l4=[15,11,13,12,14] comp_list(l3, l4) #calling the function l5=[20,21,22,23,24] l6=[20,21,22,23,24] comp_list(l5,l6) #calling the function
INPUT-

OUTPUT-
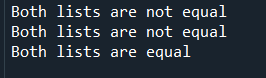
We can observe the following things-
- First, the two lists are passed l1 and l2 in the function comp_list(), and then the results are checked on the execution of our code.
- Similarly, we specified the elements in the lists l3,l4,l5, and l6 and then passed l3 and l4 in the function and then l5 and l6.
- In the output, we can observe that the elements of l1 and l2, l3 and l4 are not equal whereas elements of l5 and l6 are equal.
So, in this article, we discussed the various methods of comparing the elements of a list in Python.