Python Packages
A package is a collection of Python modules that share a similar namespace and are generated by putting all of the modules in a single directory with certain special files like _init .py.
For a folder containing several modules like.py files to be recognized as a package in a directory structure, a special file called _init .py must also be stored in the folder, even if the file _init .py is empty. A library, on the other hand, can contain one or more packages and sub-packages.
Structure of a Package
Because python packages are simply collections of modules with a common namespace, this namespace is formed by creating a directory that contains all relevant modules. One of the things one should keep in mind is that - Packages are all directories that include multiple.py modules. A _init .py file, even if empty, must be present in a folder containing Python files for the folder to be recognized as a package.
Here is a figure explaining the same;
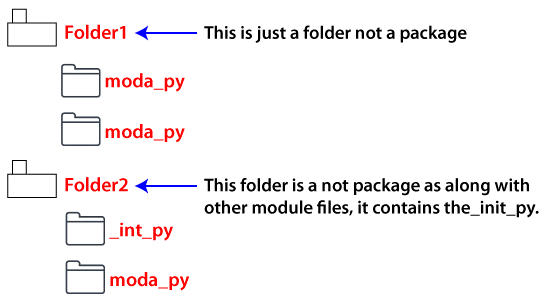
A folder's _init .py file shows that it is an importable Python package; otherwise, a folder is not regarded as a Python Package.
Procedure for Creating Packages
To create a package, you must follow a certain procedure, as discussed below.
1. Make a Decision Regarding the Package's Basic Structure
It means you should know what your package's name will be (i.e., a directory/folder with that name will be created) and what modules and subfolders (to act as sub-packages) will be included.
For instance, let’s create a package named asPackageNewwith structure as shown below;
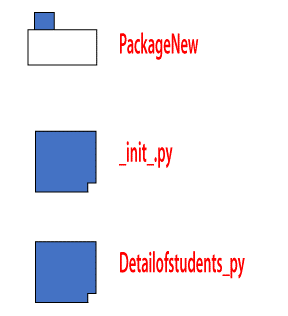
2. Create a Directory Structure with Folders Labelled with Package and Subpackage Names.
As shown in the preceding example, we established a folder called PackageNew. We construct files/modules, i.e..py, files and subfolders with their own.py files inside this folder.
3. In the Package and SubpackageFolders, Create _init .pyFiles.
Remember that Python will not check for submodules inside that directory if the _init .py file is missing; thus, efforts to import the module will fail.
4. Connect it to Your Python Installation.
Once your package directory is ready, you can associate it with Python by adding it to the current Python distribution's site-packages folder.
In Python, you can only import a library and package if it is tied to its site-packages folder.
- To check the path of the python site-packages folder, type the following two commands one after the other on the python prompt and try to locate the path of the site-packages folder:
import sys
print(sys.path)
PYTHONPATH determines the directories that the Python interpreter will look at when importing modules, and the sys.path attribute provides vital information about PYTHONPATH. - Once you've determined the location of the site-packages folder, the simplest method is to copy your own package folder and paste it there. (But one should remember that this method is not considered the usual practice.)
We simply opened the site-packages folder on our Windows installation and pasted the entire PackageNew folder.
5. Your package folder has now become a python library, allowing you to import its modules and utilize its functions after moving it into the site-packages folder of your current Python installation.
Note: The site-packages directory is the default target directory for all installed Python packages.
- The Python interpreter recognizes the folder as a package using __init.py. It also defines which modules should have their resources imported.
Installing a Python Package
There are some packages that are in the standard library; the Python Package Index, sometimes known as PyPI, is the official repository for locating and downloading third-party packages. To install packages from PyPI, use the pip package installer:
$ pip install <name of the package>
Pip is a Python package manager that can install Python packages from various sources, including PyPI.
The conda command can be used to install Python packages if you installed Python with Anaconda or MinicondaTo install a Python package or module, type the following code in Anaconda Prompt.
$ conda install <name of the module>
Conda is simple to use, but it lacks the versatility of pip. If conda fails to install a package, you can always use pip.
Importing Modules
The import Statement:
The import statement makes the contents of the module available to the caller. As shown below, the import statement can be written in many forms.
The simplest form:
import<module_name>
A single import statement can contain many comma-separated modules:
import<module_name>[,<module_name>...]
Example:
Let's pretend we haven't installed any packages yet, do not worry cause Python comes with a vast library of pre-installed packages named the Python Standard Library. It contains capabilities for various tasks, including text processing and math. Let's have a look at the second option:
import math
- It should be noted that this does not give the caller immediate access to the module's contents. Each module has its own private symbol table, which serves as the global symbol table for all objects in the module. As previously stated, a module defines its own namespace.
- The import <modulename> statement merely adds <modulename> to the caller's symbol table. The private symbol table of the module stores the objects declared in the module.
- Objects in the module are only accessible from the caller when prefixed with <module name> via dot notation.
Assume you've generated a file called module1.py that contains the following code:
array1 = [150, 250,350, 450]
string1 = "Python packages are fun to read and experiment with."
def function1(argument):
print(f'argument = {argument}')
class cls1:
pass
In module1.py, several objects are defined like:
- array1 à that is an array.
- function1() à that is a function.
- cls1 à that is a class.
- string1 à that is a string.
Here in the code below, after the following import statement, module1 is placed into the local symbol table. Thus, module1 has meaning in the caller’s local context:
>>>importmodule1
>>>module1
<module 'module1' from 'C:\\Users\\kitty\\Documents\\Python\\doc\\module1.py'>
String1 and function1, on the other hand, are stored in the module's private symbol table and have no local value.
>>>string1
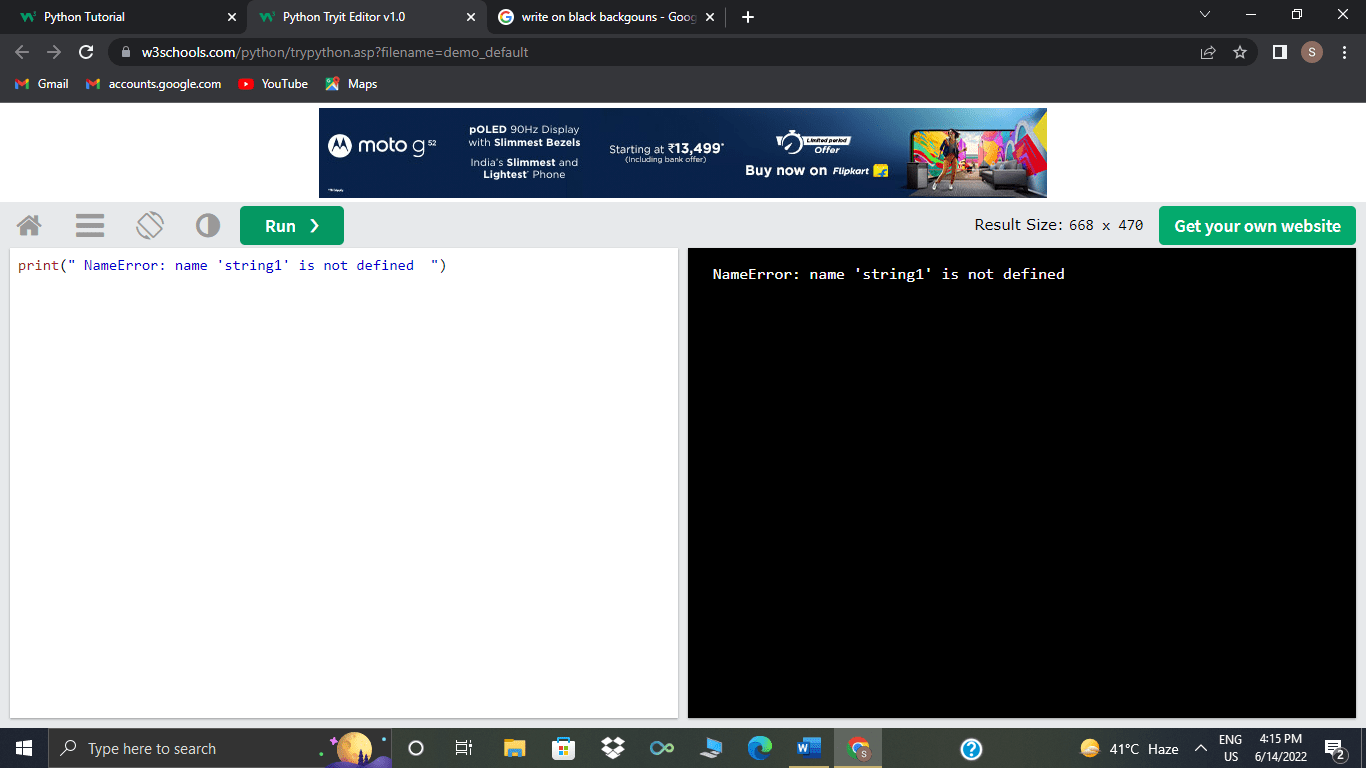
>>>function1('python')
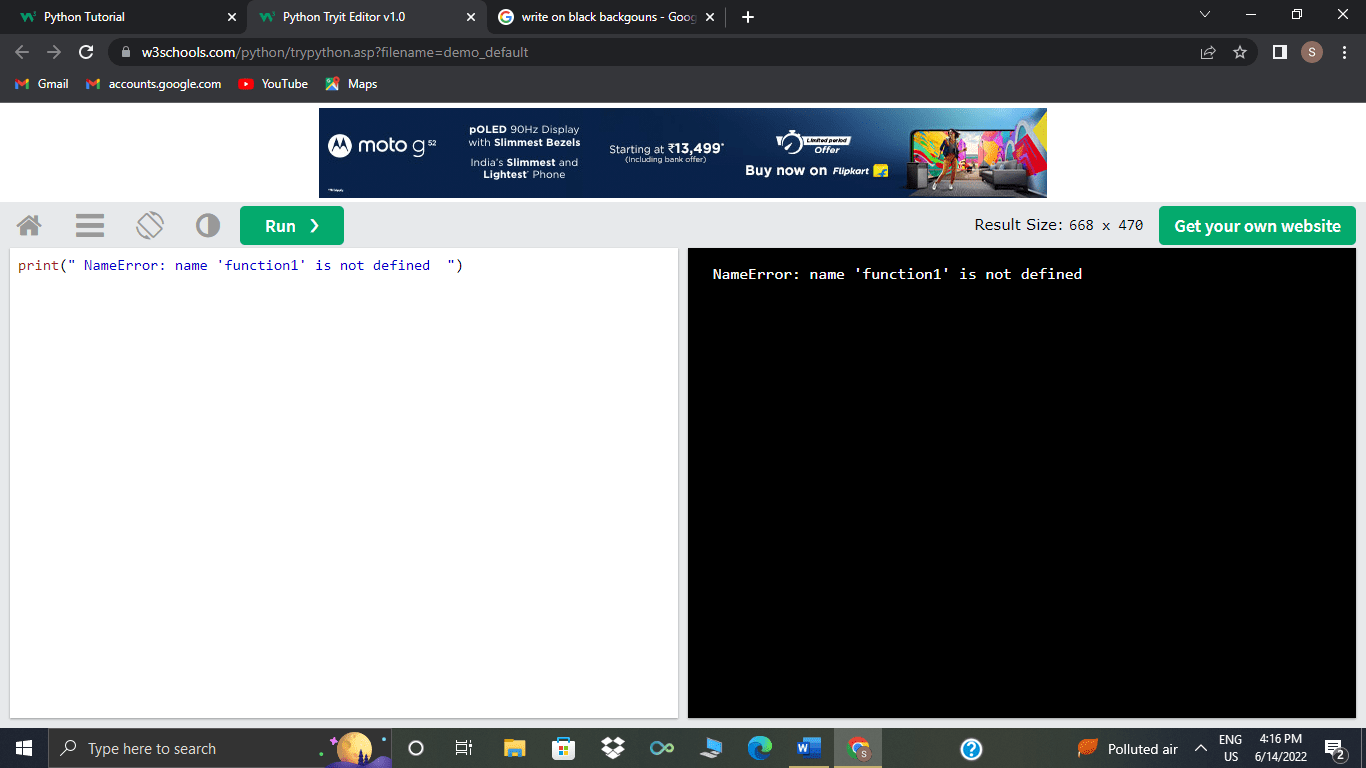
As you can see, therefore, the names of objects declared in the module must be prefixed by module1 to be accessed in the local context, as shown below;
>>>module1.string1
‘Python packages are fun to read and experiment with.’
>>>module1.function1('python')
argument = python
from <module_name> import <name(s)>
Individual objects from the module can be imported directly into the caller's symbol table using a different form of the import statement:
from <module_name> import <name(s)>
Following the execution of the above statement, the caller's environment can refer to <name(s)> without the <module name> prefix.
For example: if you want to use only the log function from the math module, then you can code as given below,
>>> from math import log
from <module_name> import <name> as <alternate_name>
Individual objects can also be imported, but they must be entered into the local symbol table with alternate names.
from <module_name> import <name> as <alternate_name>[, <name> as <alternate_name> …]
This allows names to be entered directly into the local symbol table but tries to avoid conflicts with previously used names.
Example:
>>>from math import fabs as fs
>>>math.fs(x)
import <module_name> as <alternate_name>
You can also import a module as a whole under a different name.
import <module_name> as <alternate_name>
Example:
>>>import math as mh
>>>mh.fabs(x)
Note: Another way is to import all of the resources from a module into your namespace:
>>> from math import *
Module Reloading
If you alter a module's script while programming in interactive mode, the modifications will not be imported, even if you issue another import statement. In this situation, you'll want to utilize the importlib library's reload() function:
>>>import importlib
>>>importlib.reload(<some module name>)
Using the dir() Function
The dir() method returns a list of the function names (or variable names) in a module.
All modules, even those you write yourself, can use the dir() function.
Example:
import math
x = dir(math)
print(x)
Output:
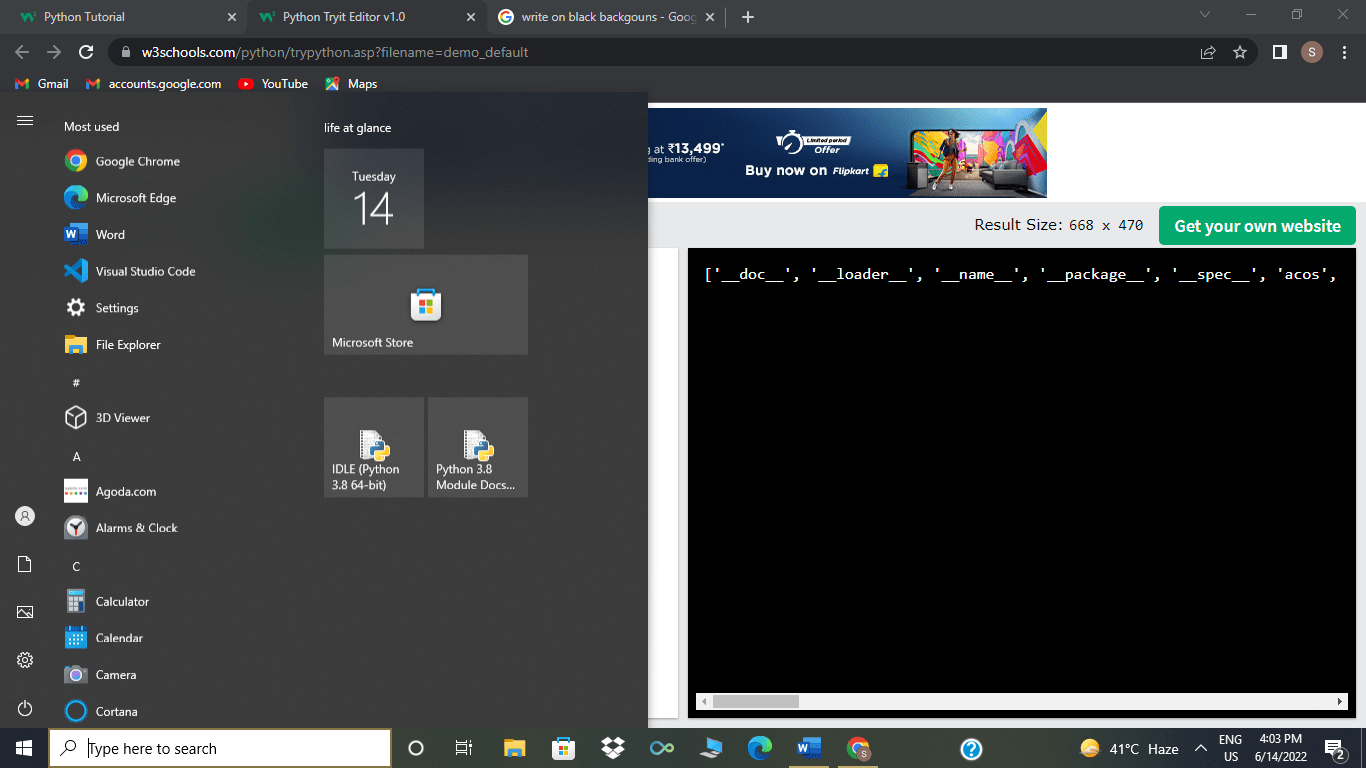
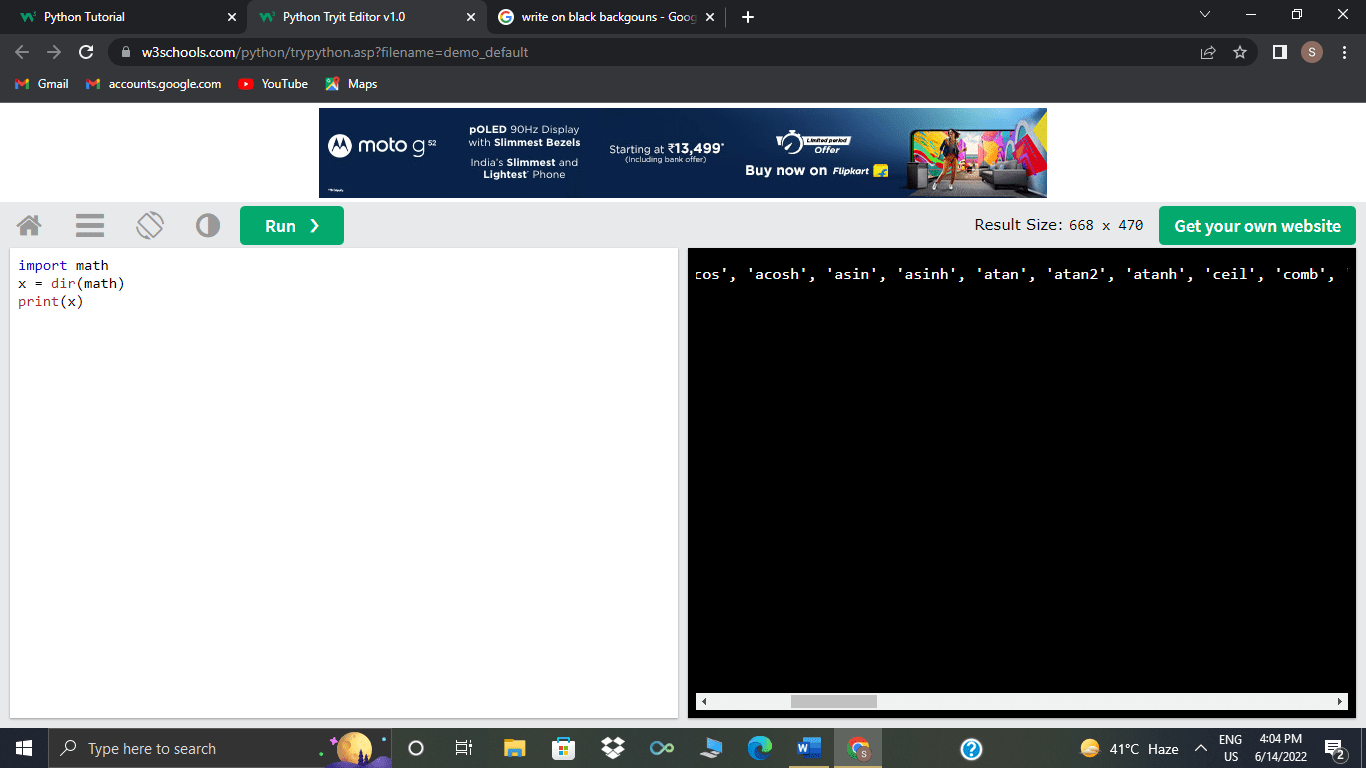
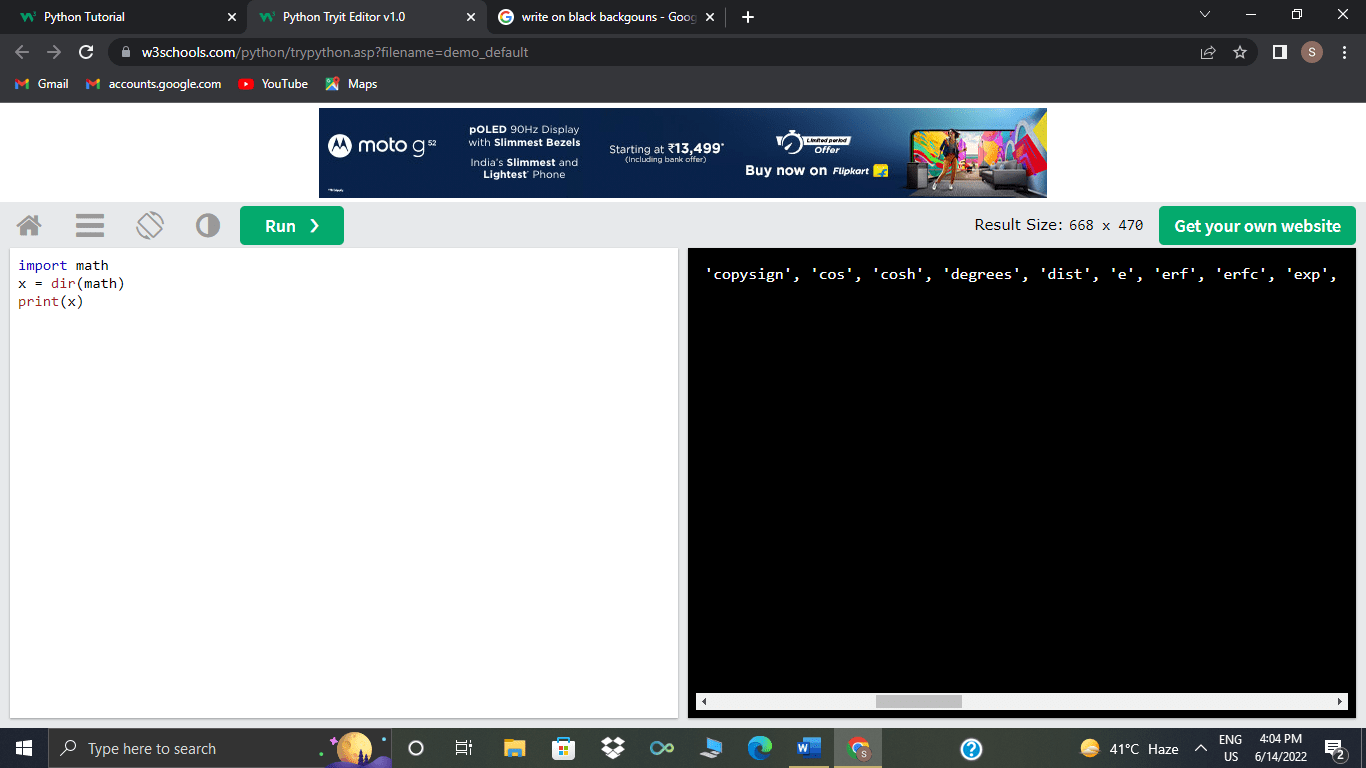
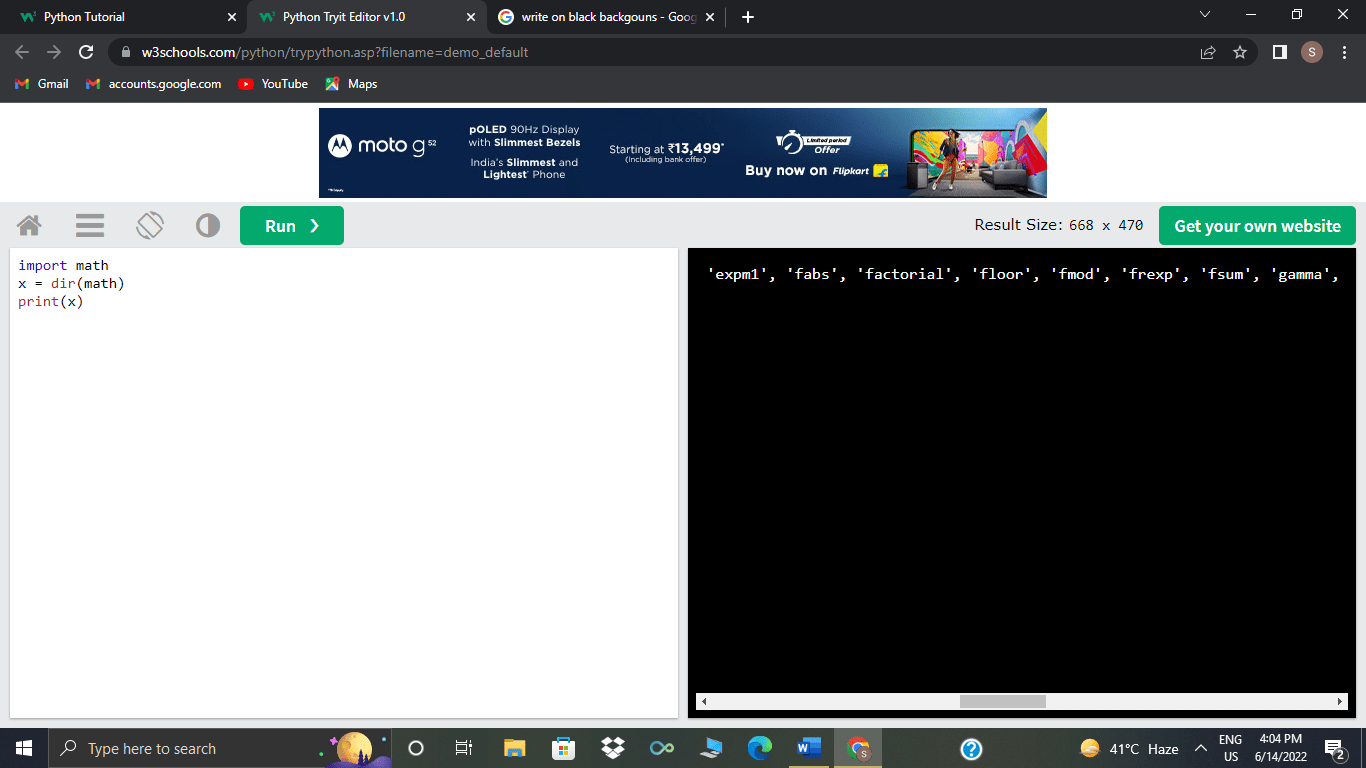
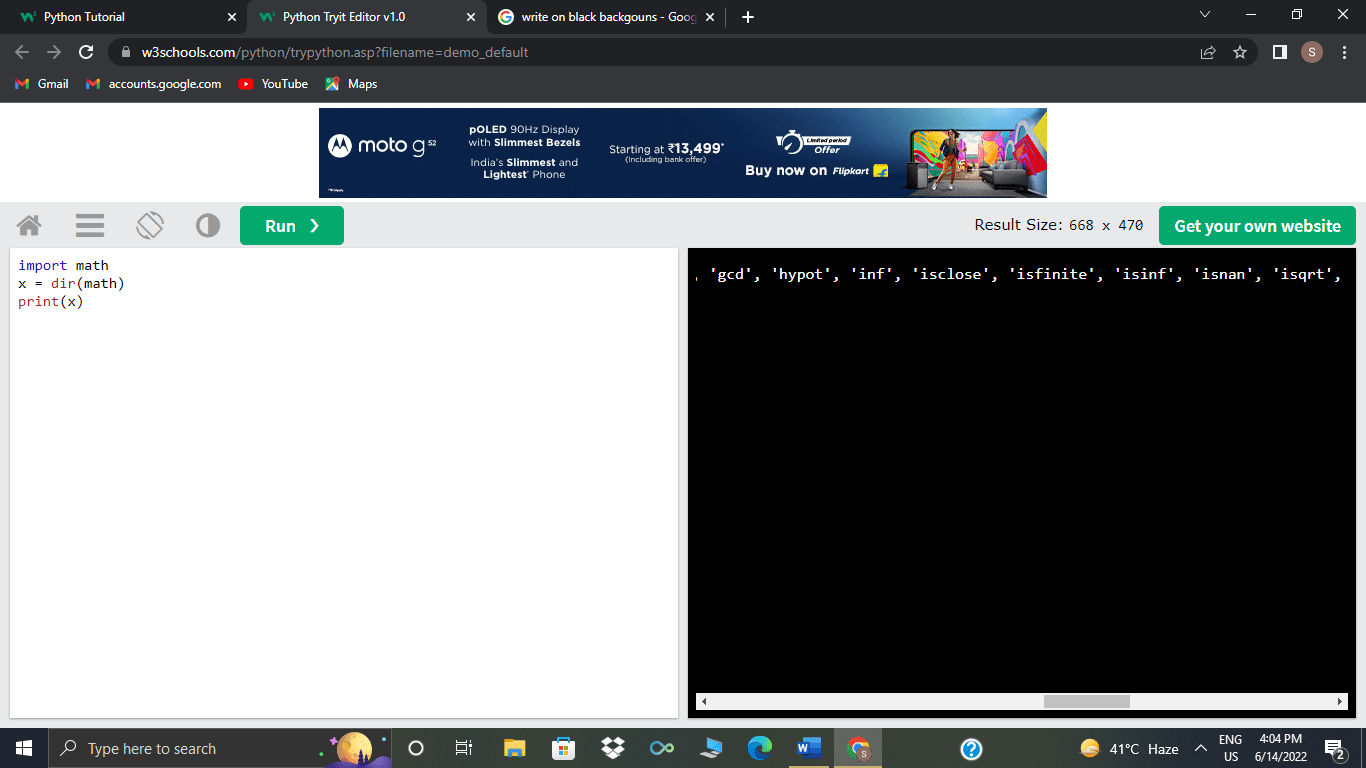
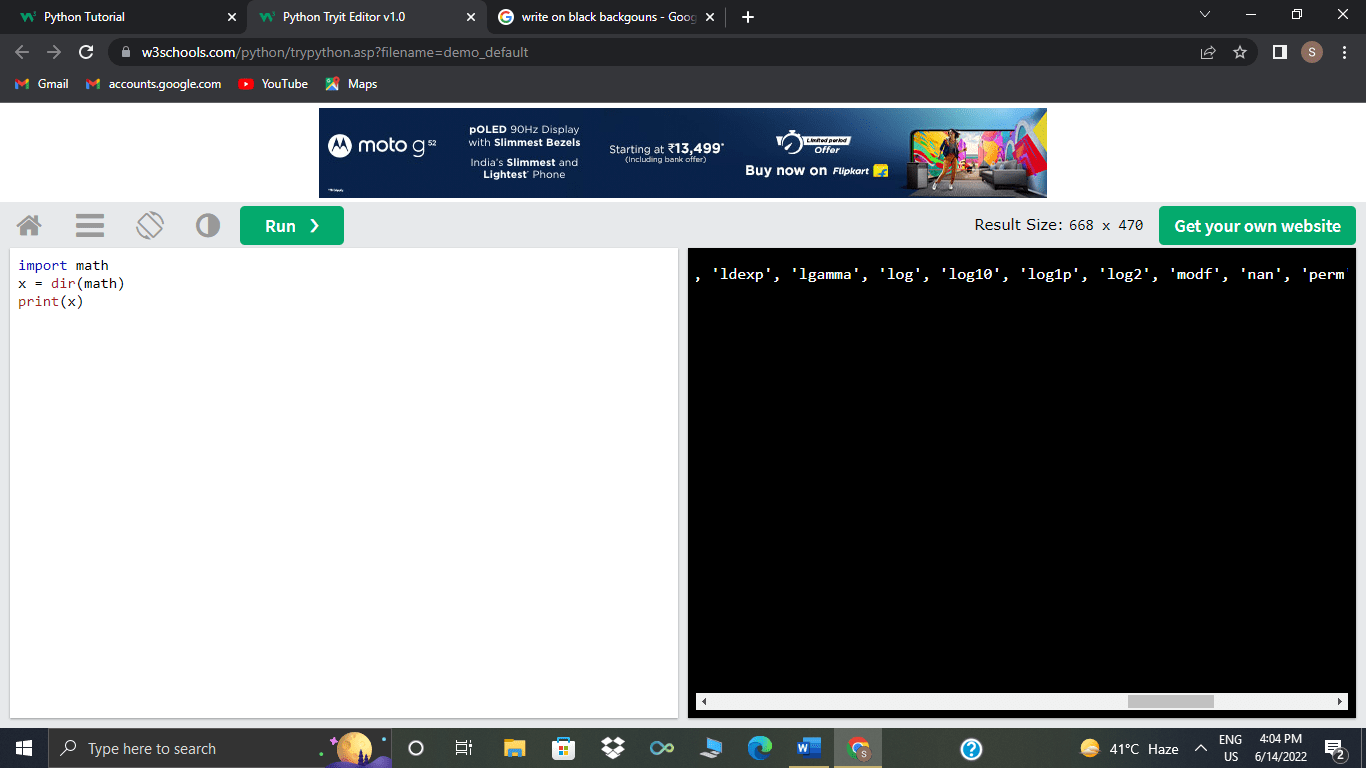
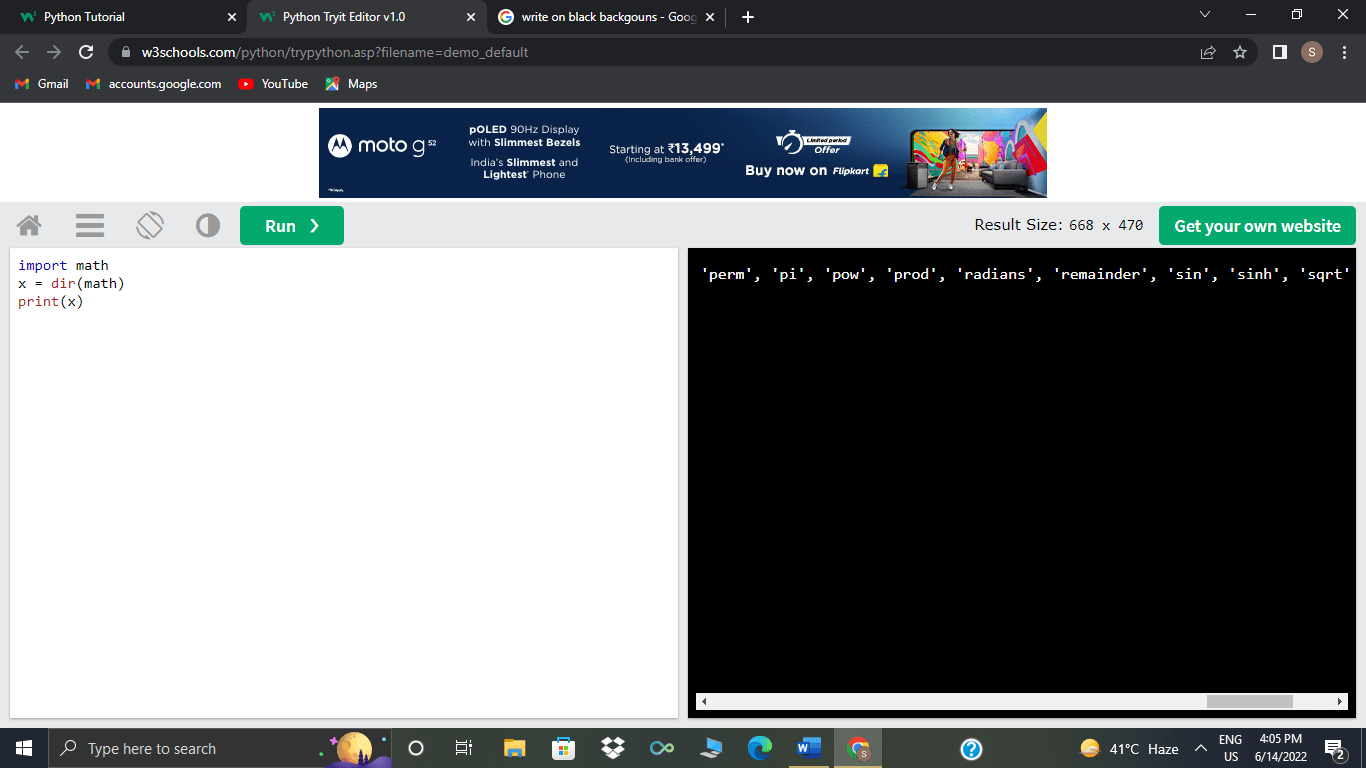
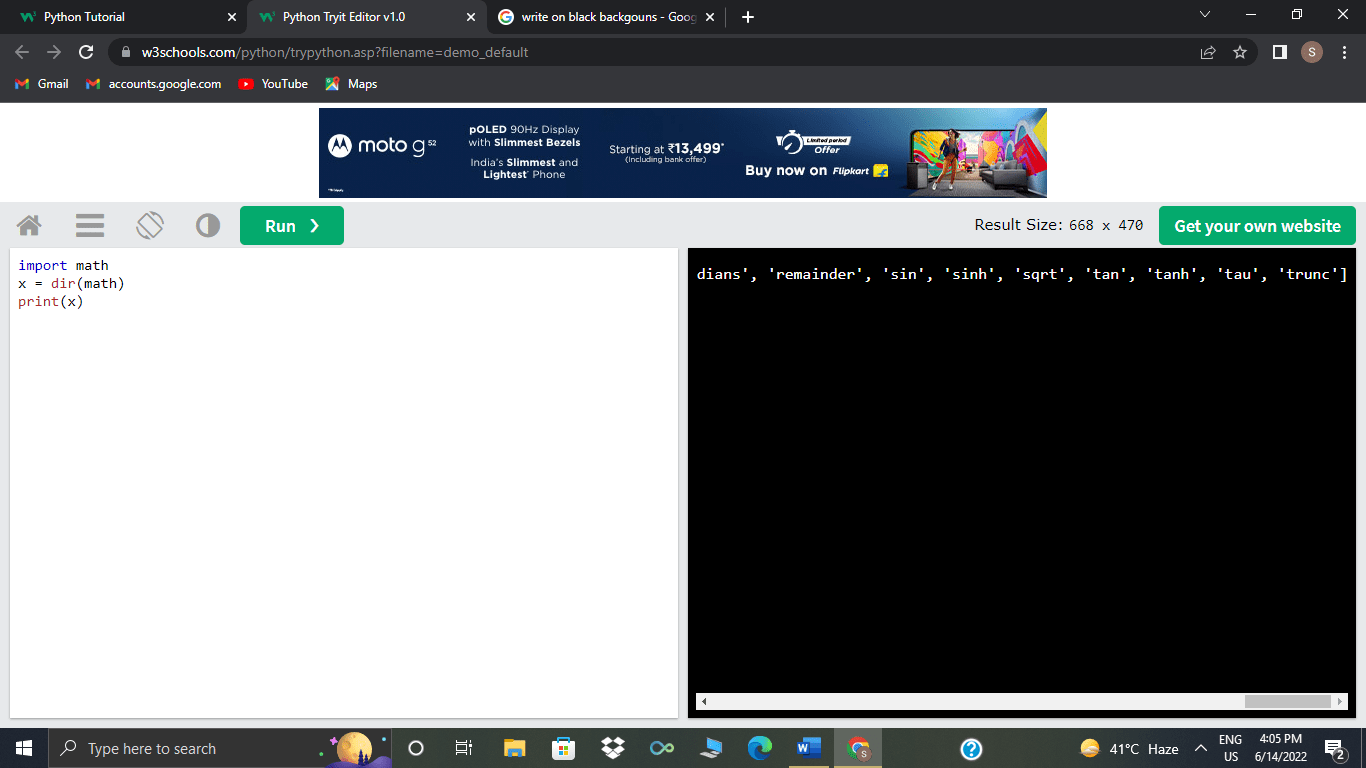