_name_ in Python
Introduction:
The code at level 0 indentation is to be performed when the command to run a Python program is supplied to the interpreter because Python does not have a main() function like the C language. Before that, though, it will define a few unique variables. One such unique variable is __name__. If the source file is launched as the main program, the interpreter changes the value of the name variable to " main ". If this file is imported from another module, name will be set to the name of the module.
What is _name_ in Python?
_name__ is a built-in variable that evaluates the name of the current module.
Note: By combining it with an if statement, as demonstrated below, it may be used to determine if the current script is being executed independently or being imported into another script.
Code:
# Program1.py
print ("Program1 __name__ = %s" %__name__)
if __name__ == "__main__":
print ("Program1 is being run directly")
else:
print ("Program1 is being imported")
Output:
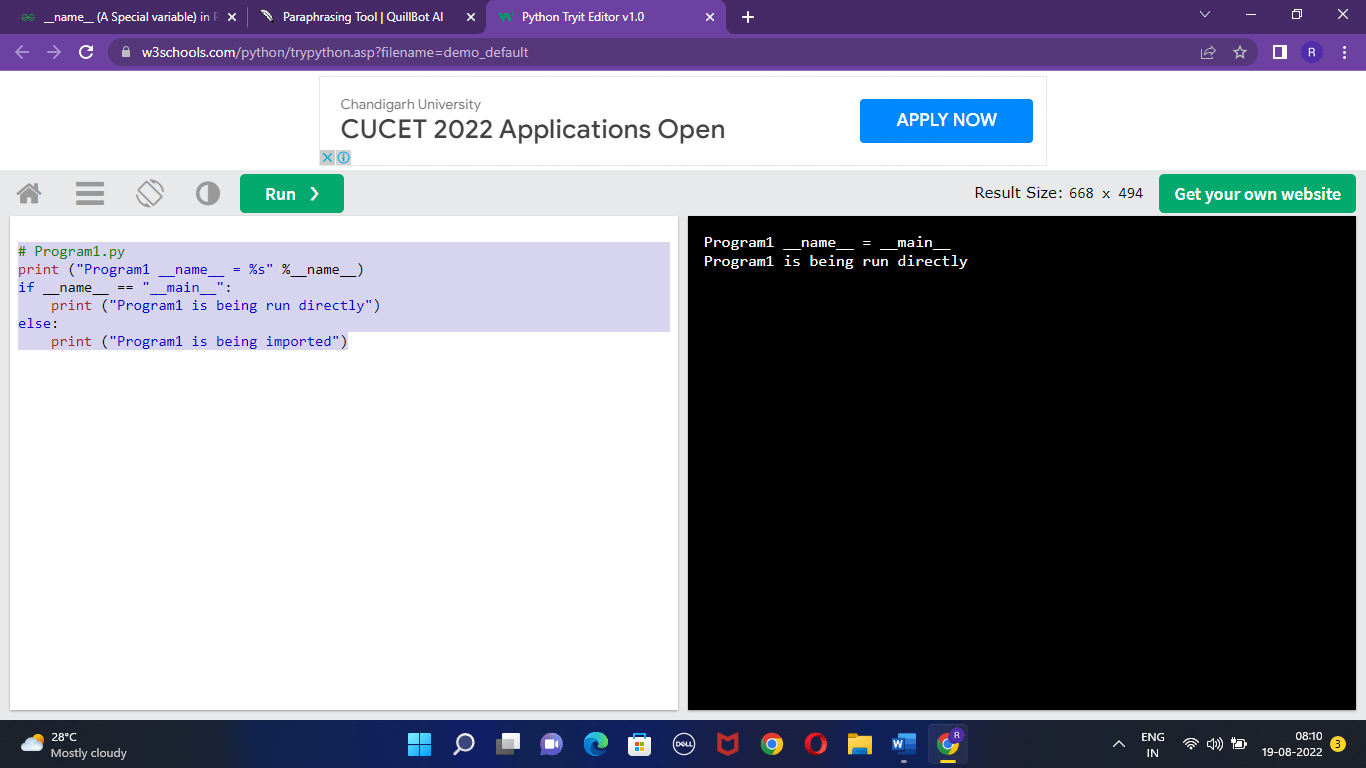
Why is the Variable "_name_" Used?
One of the unique Python variables is _name . Depending on how we run the contained script, it derives its value.
There are occasions when you write a script that has functionalities that might be applied to other scripts as well. That script can be imported into another script in Python as a module.
You can choose whether to execute the script using this unique variable. Or perhaps you wish to import the script's defined functions.
What Types of Values may the Variable _name_ Hold?
The __name__ variable takes on the value __main__ when your script is executed. When you import the enclosed script, the name of the script will be shown.
Understanding This through an Example:
Make a script in Python called Script1.py, and add the following code to it:
Code:
#Script1.py
# A function is defined
def Valuestored():
print('Value of __name__:', __name__)
Valuestored()
Output:
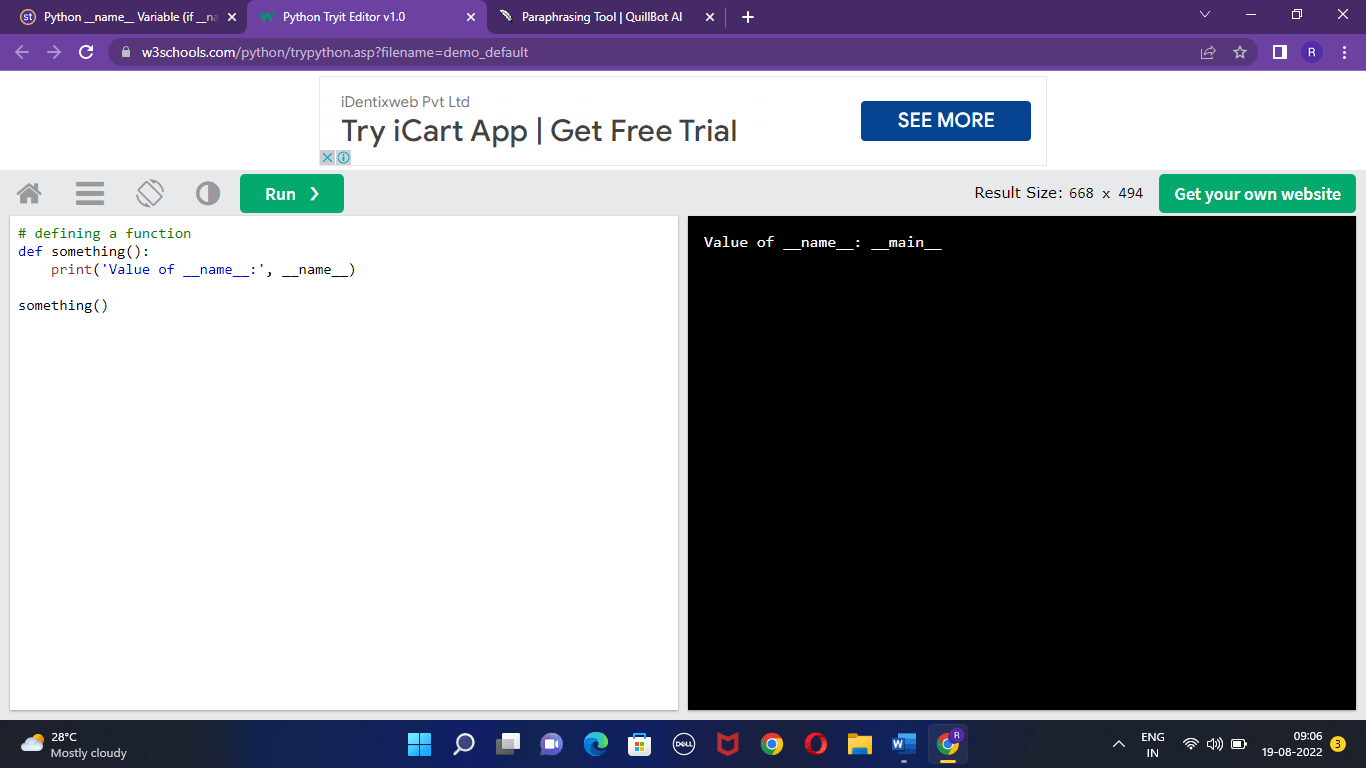
The value for the __name__ variable was set to __main__ when we ran the Script1.py script.
Let's now build another Python script called Script2.py to which you can import the previous one.
Code:
#Script2.py
# importingScript1.py
import Script1
Script1.Valuestored()
Output:
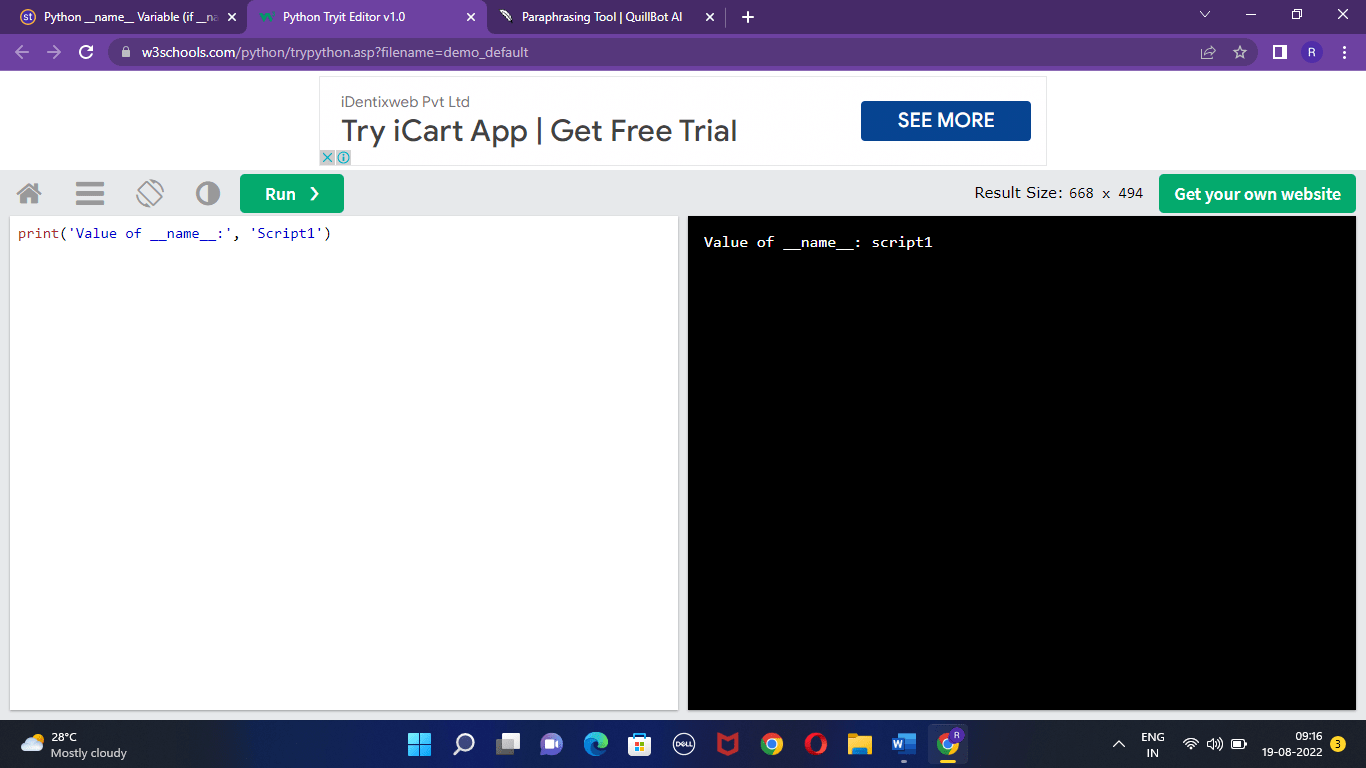
The Script1.py module's name variable's value was printed, therefore you can see from the output of the code above that the variable's value is Script1.
If __name__ == __main__ is Used:
If you want to restrict the execution of certain Python code to only when the script is run directly, use the if statement with the condition name == main .
As an example;
Code:
# importingScripy1.py
import Script1
if __name__ == __main__:
Script1.Valuestored()
Here, it is possible to tell whether the current module or script is running independently or not using the string main. The name variable has two underscores on either side of the name to indicate to the Python interpreter that it is a reserved or special term.
_name_ in Python: Example using Code:
It has already been mentioned that when we run a code file, the value of the name variable changes to main since the code is executed directly rather than being imported into another file.
Code:
#Code1.py
# A function is defined
def Value():
print('Function is in Code1.')
if __name__=="__main__":
Value()
print('Called from Code1.')
else:
print('Seems like Code1 is imported in some other file.')
Output:
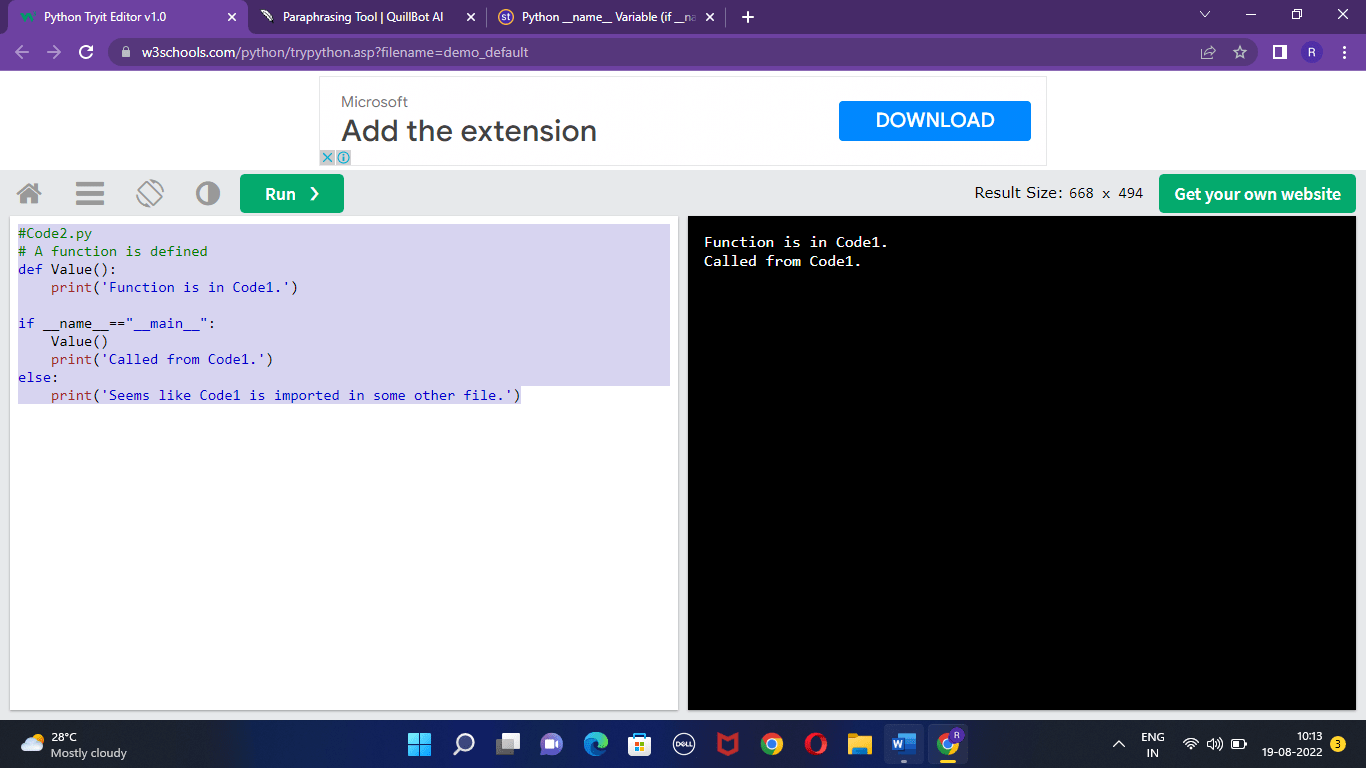
Let's now create a new Python script file called Code2.py, import Code1.py into it, and attempt to invoke the function Values() that is defined in Code1.
Code:
import Code1
if __name__=='__main__':
Code1.Value()
print(' This is Called from Code2.')
Output:
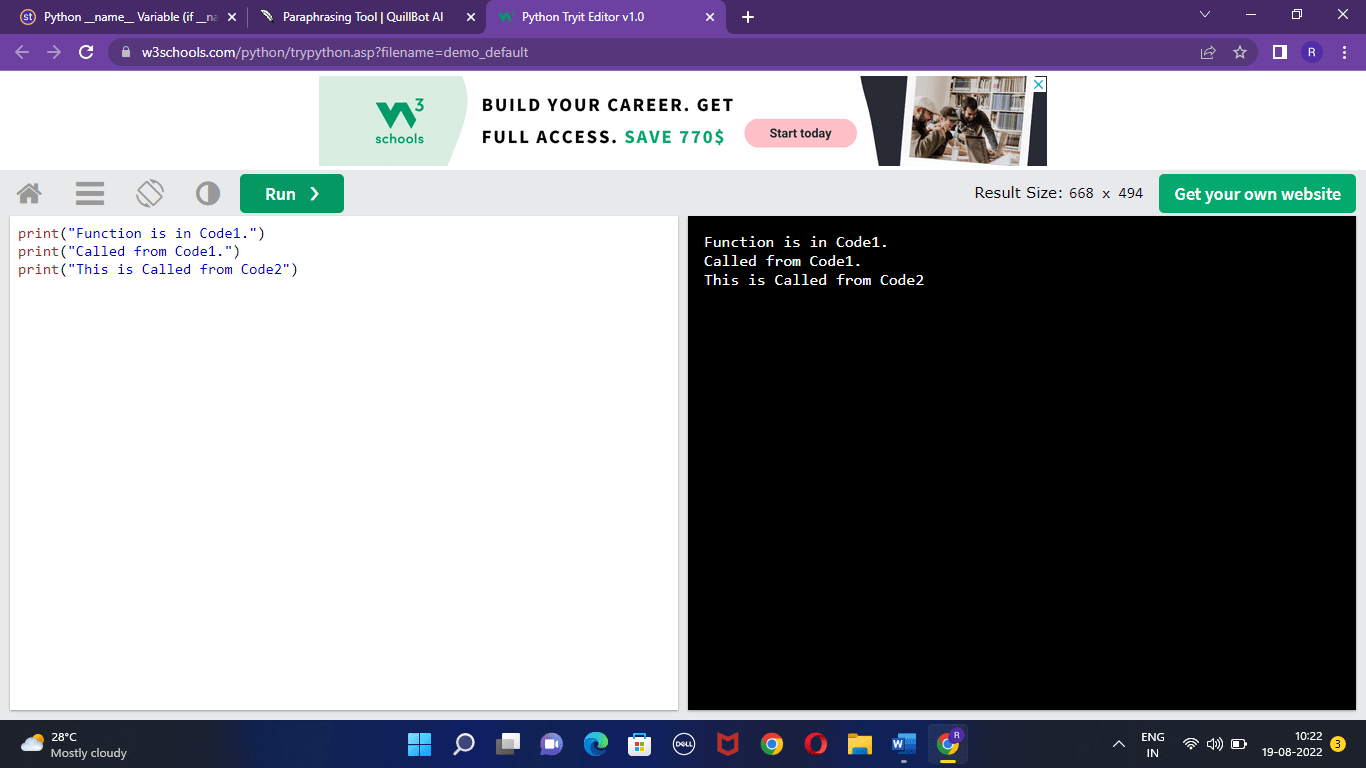
When the import statement for Code1 was run inside Code2, the name variable had the value Code1 (the name of the module), but since Code2 was the first script to be run, it now has the value main.
Conclusion
- The scope of the code being run is stored as a string in the Python special variable __name__.
- In most programming languages, the main method or function is often utilised as the point at which any program is performed. But what about Python? The first line, which represents the program's indentation level 0, is often where a Python program (script) starts to run.
- The creation of a named variable occurs before a Python program is run, though. This variable can be utilized in Python in place of the main method.
- When you execute the script directly in Python, it assigns the '__main__' variable to the __name__ variable, and if you import the script as a module, it assigns the module name.