Python And Operator
Python's and operator takes two operands that can be either object, Boolean expressions, or both. And operator creates more complex expressions using those operands. Conditions are the common name for the operands in and expression. And expression yields a true result if both requirements are satisfied. Otherwise, a false result is returned:
Code:
print(True and True )
print(False and False )
print(True and False )
print(False and True )
Output:

- These illustrations demonstrate that and expression only return True if both operands are true. And operator is a binary operator since it requires two operands to create an expression.
The few illustrations above demonstrate and operator's truth table.
operand1 | operand2 | operand1 and operand2 |
True | True | True |
True | False | False |
False | False | False |
False | True | False |
This table lists the truth value of a Boolean expression with operand1 and operand2 as examples. The truth values of the operands of the expression influence its result. If both are true, it will be true. It will be untrue if not. The general reasoning behind the and operator is as follows. In Python, this operator is capable of more, though.
Using Python and Operator with Boolean Expressions
To create compound Boolean expressions, combinations of variables and values that result in a Boolean value, you'll often utilize logical operators. Boolean expressions thus return True or False.
Common examples of this kind of expression include comparisons and equality tests:
print(6 == 3 + 3)
print(7 > 5 )
print(4 < 3 )
print(22 != 78 )
print([4, 9] == [4, 9] )
print("Ban" == "Tank" )
Output:
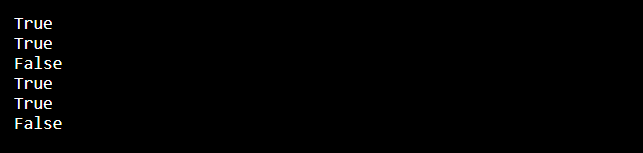
These are all Boolean expressions since they all have a True or False return value. And keyword can be used to combine them to construct compound expressions that test two or more sub expressions simultaneously:
Code:
print(6 > 3 and 6 == 3 + 3)
print(7 < 2 and 7 == 7)
print(4 == 4 and 9 != 9)
print(3 < 2 and 6 != 6)
Output:

In this case, combining two True expressions yields another True expression. All other combinations give False results. These examples show that the syntax for building compound Boolean expressions using and operator is as follows:
expression1 and expression2
If both expressions 1 and 2 evaluate as True, the compound expression is True. If at least one subexpression evaluates to False, the result is False.
When creating a compound expression, there is no restriction on how many and operators you can employ. This indicates that you can combine more than two subexpressions in a single expression by using multiple and operators:
Code:
print(7 > 3 and 7 == 3 + 4 and 8 != 3)
print(8 < 3 and 0 == 3 and 5 != 4)
Output:

Once more, you get True if the evaluation of all the sub expressions is True. If not, you receive False. It would be best if you remembered that Python evaluates the expressions progressively from left to right, especially as the expressions grow longer.
Short-Circuiting the Evaluation
- The logical operators 'and' and 'or' in Python employ a technique known as short-circuit evaluation, also referred to as lazy evaluation. The operand on the right is only evaluated by Python when it is necessary, to put it another way.
- Python determines the final result after evaluating the left operand of the and expression. If it is false, the entire expression is false. In this instance, it is not necessary to evaluate the operand on the right. Python is already aware of the outcome.
- The entire expression is inherently false if the left operand is false. Evaluating the final operand would be a waste of CPU time. Python avoids this by stopping the evaluation midway through.
- • The right operand is only evaluated by and operator, in contrast, if the first operand is true. The truth value of the right operand, in this instance, determines the outcome. The entire expression is true if it is true. Otherwise, the statement is untrue.
- Check out the examples below to see how the short-circuiting feature works:
Code:
def true_function():
print("Running true_function()")
return True
def false_function():
print("Running false_function()")
return False
print(true_function() and false_function())
print(false_function() and true_function())
print(false_function() and false_function())
print(true_function() and true_function())
Output:
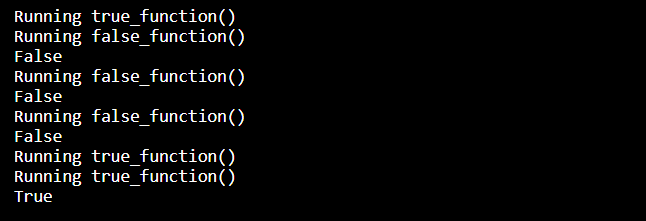
- And operator behaves lazily in the first expression as predicted. The second function is not evaluated because the outcome of the first function's evaluation is false. Although the first function returns False in the second statement, the bitwise AND operator (&) eagerly performs both functions. Take note that the outcome is False in both situations.
Using Python and Operator with Common Objects
Use and operator to combine two Python objects into a single expression. In that case, Python's internal bool() function is used to determine the operands' truth values. You consequently receive a particular object rather than a Boolean value. Only when an operand explicitly evaluates to True or False do you receive True or False:
Code:
print(3 and 4)
print(6 and 0.0)
print([] and 5)
print(0 and {})
print(False and "")
Output:

In these instances, if the and expression evaluates to False, it returns the operand on the left. If not, the operand on the right is returned. And operator determines an object's truth value using internal Python rules to produce these results.
- Here is a summary of how the and operator behaves when applied to ordinary Python objects instead of Boolean expressions. Note that Python determines the outcome by using the truth value of each object:
object1 | object2 | object1 and object2 |
False | False | object1 |
False | True | object1 |
True | True | object2 |
True | False | object2 |
Generally, if an and expression's operands are objects rather than Boolean expressions, the operator yields the object on the left if the expression evaluates to False. The item on the right is still returned even if it evaluates to False.
Mixing Boolean Expressions and Objects
Additionally, you can use an and expression to combine Boolean statements with typical Python objects. The and expression continues to return the left operand if that condition is true; otherwise, it returns the right operand. Depending on which component of the expression yields that result, the returned value could either be True, False or an ordinary object:
Code:
print(6 < 7 and 6)
print( 6 and 6 < 7)
print( 4 < 6 and [])
print([] and 4 < 6)
print(7 > 11 and {})
print({} and 7 > 11)
print(6 > 9 and 5)
print(5 and 6 > 9)
Output:
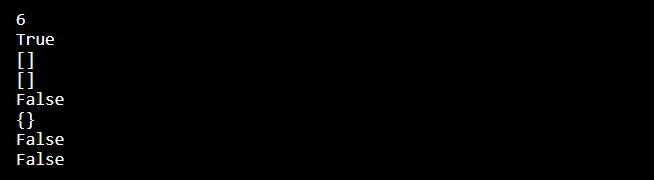
These examples combine the use of common objects and Boolean expressions. You can see that for each set of examples; you can either get a non-Boolean object or a Boolean value, either True or False. Which component of the expression yields the final result will determine the outcome.
Here is a table summarizing how and operator behaves when Boolean expressions and specific Python objects are used together:
expression | object | expression and object |
True | True | object |
True | False | object |
False | False | False |
False | True | False |
- Python calculates the result by evaluating the Boolean expression on the left and obtaining its Boolean value (True or False). The truth value of the object on the right is then determined by Python using its internal rules.
Combining Logical Operators in Python
- Python also provides or operator and the not operator as additional logical operators.
- They can be combined with and operator to form more sophisticated compound expressions.
- The precedence of each logical operator must be considered when creating accurate and understandable expressions using numerous logical operators. To put it another way, you must consider the order in which Python executes them.
Compared to other operators, the logical operators in Python have low precedence. To achieve a consistent and readable outcome, it's advisable to employ a pair of parentheses (()) occasionally:
Code:
print(6 or 3 and 7 > 1)
print((6 or 4) and 7 > 1)
Output:

- In these instances, a compound phrase is created by combining the 'or' and 'and' operators. Or operator takes advantage of short-circuit evaluation, just like and operator. Or operator, in contrast to and, terminates when it encounters a valid operand. This is seen in the first illustration. Or sub expression returns 6 immediately without assessing the rest of the expression because 6 is true.
- Or sub expression, however, operates as a single true operand when enclosed in a pair of parentheses, and 7> 1 is also evaluated. The conclusion is True.
Using Python and Operator in Boolean Contexts
And operator, like all of Python's Boolean operators, is particularly helpful in Boolean settings. Most Boolean operators' real-world usage cases can be found in Boolean settings.
Boolean Contexts are defined by Two Primary Structures in Python:
- If statements: Let you perform conditional execution and take different courses of action based on the result of some initial conditions.
- While statement: Allow you to do repetitive operations while a certain condition is true and perform conditional iteration.
What you might refer to as control flow statements include these two forms. They aid in determining the course of your program’s execution.
In both if statements and while loops, you can create compound Boolean expressions using Python's and operator.
- while Loops
The second structure that allows and expressions to be used to control how a program is executed is the while loop. The while statement header's 'and' operator enables you to test several assumptions and continue the loop's code block until all assumptions are satisfied.
Code:
from time import sleep
from random import randint
def control_pressure():
pressure = measure_pressure()
while True:
if pressure <= 500:
break
while pressure > 500 and pressure <= 700:
run_standard_safeties()
pressure = measure_pressure()
while pressure > 700:
run_critical_safeties()
pressure = measure_pressure()
print("Wow! The system is safe...")
def measure_pressure():
pressure = randint(490, 800)
print(f"psi={pressure}", end="; ")
return pressure
def run_standard_safeties():
print("Running standard safeties...")
sleep(0.2)
def run_critical_safeties():
print("Running critical safeties...")
sleep(0.7)
if __name__ == "__main__":
control_pressure()
Output:
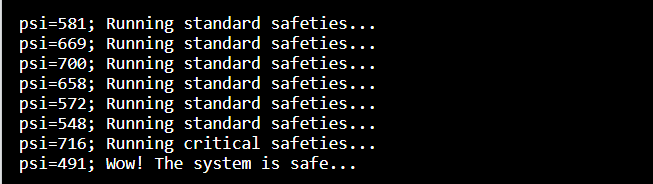
On line 8 of control pressure(), you add an endless while loop. The conditional statement exits the loop, and the program is completed if the system is stable and the pressure is less than 500 psi.
Line 12 has a first nested while loop that executes the regular safety procedures while maintaining a system pressure of between 500 and 700 psi. The loop receives a new pressure measurement after each iteration to retest the situation. The second loop on line 16 executes the crucial safety measures if the pressure exceeds 700 psi.
- If Statements
- Boolean expressions are frequently referred to as conditions since they frequently suggest the necessity of fulfilling a specific criterion.
- They are quite helpful when used with conditional statements. Such a sentence starts with the Python keyword if and goes on to include a condition.
- Conditional statements may also contain elif and else clauses.
The logic of conditionals in English grammar is followed by conditional statements in Python. If code block runs if the condition is met. If not, the execution moves on to another code block:
Code:
x = -11
if x < 0:
print("x is negative")
elif x > 0:
print("x is positive")
else:
print("x is equal to 0")
Output:

- The statement "x< 0" is true because it contains a negative value. The if code block executes, and the screen displays the message "x is negative." However, if you alter the value of a to a positive number, the elif block will activate, and Python will print that it is positive. Lastly, if you set a to zero, the else code block executes. Try different things and see what happens.
- Let's imagine that before running a certain piece of code, you want to confirm that two criteria are satisfied—that is, they are both true.
Code:
age = int(input("enter your age: " ))
if age >= 0 and age <= 9:
print("You are a child!")
elif age > 9 and age <= 18:
print("You are an adolescent!")
elif age > 18 and age <= 65:
print("You are an adult!")
elif age > 65:
print("Golden ages!")
Output:
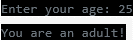
- Here, input() is used to obtain the user's age, and int is used to convert it to an integer value (). It is used to determine whether age is higher than or equal to 0. The same clause determines if the applicant's age is nine or less. You construct a composite Boolean expression to accomplish this.
- To establish the life stage related to the user's age, the three elif clauses examine other intervals.
The script follows one of two paths depending on the age you specify at the command line. In this example, if you enter 25 as your age, the phrase "You are an adult!" appears on your screen.