Python List Size
Introduction
The list data type in Python is an ordered, flexible collection. A list may also contain duplicate entries. To get the size of any object, use the len() function in Python. The steps to finding a list's length in Python are covered in this article in the following order:
- List in Python
- How to find the Length of a List in Python?
List in Python
Python uses lists to implement the sequence of different sorts of data. There are 6 data types in Python that can store sequences, but a list is the most popular and dependable kind.
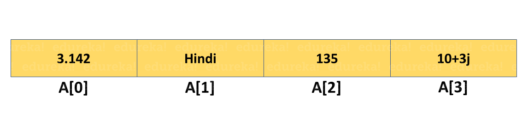
A list is characterized as a grouping of items or values of various categories. The list's items are surrounded in square brackets [] and separated by commas (,).
It is described as follows:
list_1 = ['java', 'python', 2022];
list_2 = [1, 2, 3, 4, 5];
list_3 = ["a", "b", "c", "d"];
How can I Determine a Python List's Length?
The most popular and fundamental techniques for determining a list's length in Python are as follows:
1. How to Use the len() Function to Determine a List's Length:
To determine the total number of elements in a tuple, list, array, dictionary, etc., use the built-in function len(). The list's length is returned by the len() method, which accepts a list as an input.
One of the most popular and practical ways to get a list's length in Python is to use the len() function. Today's programmers all use this method because it is the most traditional.
Syntax:
len (list)
The following line of code demonstrates how to use the len() method to get a list's length:
Code #1:
# Use Python application to show how len() function works
x = []
x.append("Python")
x.append("Java")
x.append("C")
x.append("C++")
print ("Using len() function, the size of the list is: ", len(x))
Output:
Using len() function, the size of the list is: 4
Code #2:
# Using Python application to
# show how len() function works
size = len ([10, 20, 30, 40, 60, 50, 80])
print ("The length of the list is: ", size)
Output:
The length of the list is: 7
2. How to Use the length_hint() Function to Determine a List's Length
A less popular method for determining the size of a list and other iterables is length_hint().
You must import length_hint() from the operator module in order to use it because it is defined there.
The length_hint() method's syntax is length hint (list_Name).
You can see how to utilize the length_hint() method from operator import length_hint in the example below:
Code:
# Python code to show list length
# using a len() and length_hint technique
# from operator import length_hint
# Put the value of the list
list_1 = [ 4, 64, 7, 3, 6, 4, 1, 8, 30, 6, 31, 5, 35]
# Print out list_1
print ("The list is : " + str(list_1))
# Using len(), Finding the size of the list
list_size = len(list_1)
# Using length_hint(), finding size of list
list_length_hint = length_hint(list_1)
# Print out the length of the list
print ("List size calculated using len() is : " + str(list_size))
print ("List size calculated using length_hint() is : " + str(list_length_hint))
Output:
The list is: [4, 64, 7, 3, 6, 4, 1, 8, 30, 6, 31, 5, 35]
List size calculated using len() is : 13
List size calculated using length_hint() is : 13
3. How to Use the length Naive Method to Determine a List's Length
The most popular technique for determining a list's length in Python is the len() method. However, there is another straightforward way that gives the list's length.
To determine the count using the Naive technique, one simply runs a loop while increasing the number up to the last member of the list. In the absence of other effective strategies, this is the most straightforward tactic that can be applied.
The following line of code demonstrates how to use the Naive method to get a list's length:
Code:
# Python code to show list length using a naive technique
# Put the value of the list
list_1 = [ 4, 64, 7, 3, 6, 4, 1, 8, 30, 6, 31, 5, 35]
# Print out list_1
print ("The list is : " + str(list_1))
# Using a loop, find the length of the list
# Initializing count
count = 0
for j in list_1:
# Increment the count
count = count + 1
# Print out the length of the list
print ("The list size calculated using naive methods is : " + str(count))
Output:
The list is : [4, 64, 7, 3, 6, 4, 1, 8, 30, 6, 31, 5, 35]
The list size calculated using naive methods is : 13
4. How to Use the length __len__() Method to Determine a List's Length
Another built-in way available in Python to get the size of the list is the __len__() method.
Although the __len__() and len() methods have a similar appearance, they differ just a little bit.
The __len__() method, which returns the counts of elements in the sequence, or the list, is called internally when the len() function is used. Additionally, as demonstrated in the example below, we can call the __len__() function directly.
Code:
# List length is determined by a Python program
# using the __len__() function.
# List initialization to determine the length
My_List = ['India', 'USA', 'UK', 'Russia', 56, 343, 76, 78, 23];
# Print out My_List
print ("The list is : " + str(My_List))
# Get the size of the list
len = My_List.__len__()
print ("Using _len_() function, the size of the list is: ", len)
Output:
The list is : ['India', 'USA', 'UK', 'Russia', 56, 343, 76, 78, 23]
Using _len_() function, the size of the list is: 9
Performance Analysis – len() vs length_hint() vs Naive vs _len_() Method
It is always vital to have a good justification for selecting one alternative over another when making decisions between alternatives. In order to provide a better option to utilize, this section performs a time study of how long it takes to run each one.
Code:
# Python function to display list length Performance Evaluation
from operator import length_hint
import time
# Putting the value of the list
demo_list = [ 1, 4, 5, 7, 8, 2, 9, 34, 67]
# Printing demo_list
print ("The list is : " + str(demo_list))
# Using a naive, find the size of the list
# Initializing count
startTime_naive = time.time()
count = 0
for j in demo_list:
# increment the count
count = count + 1
endTime_naive = str(time.time() - startTime_naive)
# Using a len(), find the size of the list
startTime_len = time.time()
list__len = len(demo_list)
endTime_len = str(time.time() - startTime_len)
# using length_hint(), finding the length of the list
startTime_hint = time.time()
list_length_hint = length_hint(demo_list)
endTime_hint = str(time.time() - startTime_hint)
# using _len_(), finding the length of the list
startTime__len_ = time.time()
list_length__len_ = demo_list.__len__()
endTime__len_ = str(time.time() - startTime__len_)
# Print out the Times of each
print ("Using naive method, time taken is : " + endTime_naive)
print ("Using len() function, time taken is : " + endTime_len)
print ("Using length_hint() function, time taken is : " + endTime_hint)
print ("Using _len_() function, time taken is : " + endTime__len_)
Output:
The list is : [1, 4, 5, 7, 8, 2, 9, 34, 67]
Using naive method, time taken is : 0.0
Using len() function, time taken is : 0.0
Using length_hint() function, time taken is : 0.0
Using _len_() function, time taken is : 0.0
Best Method for Determining a Python List's Length
The Python built-in len() method is regarded by programmers as the best technique for determining the size of the list out of all the techniques previously discussed.
Because the list is an object and has memory space available for storing the size, the len() function needs O(1) time to determine the list's size.
Conclusion
As a result, we now understand the various methods for determining a Python list's length. As well as understand which method is best. The OS and a few of its parameters have a significant impact on how long it takes. You might receive different results from two runs in a row; in some cases, naïve takes the shortest amount of time out of the three.