What does the if __name__ == "__main__" do in Python
In this article, you will learn about the If__name__==__main__ is a statement in python to define modules and the names of the modules. This statement plays a vital role in every program that will require creating a function or calling a particular function into the current program. In those cases, this statement is used to assign variables __name__ and __main__ to the file's name.
Every time before an execution of a program, the python interpreter will set a particular variable when it reads the file. In those variables "__name__" is also one variable. Every python file will be saved and recognized by an extension called ".py".
Python language has many modules, so every time we use a module, the python interpreter will set the variable __name__ as __main__. This will be the case only when the module is given in the main program. In other instances where the module is imported from another depending module, the __name__ variable will be assigned to the module's name.
A module is a group of functions that contain python definitions and statements. A module can access many functions, classes, and also variables. Having modules makes the program look more understandable and easy to write because there will be no use in rewriting the whole code, which might make the code look complex.
To make the module more understandable, we use this if __name__==__main__ statement.
Let us look at an example code to understand this concept clearly.
Example
# Python program for execution
# the main program directly
print ("always executes")
if __name__ == "__main__":
print ("executes only when invoked directly")
else:
print ("executes when imported only")
Output:

The code present in the first indentation level will get executed, but all the functions and class codes will not be executed.
As we can see in the above code, the if statement only allows the first indentation and will not execute the other statements. You can also test whether your scripts run directly or the scripts are being imported from other testing variables.
Let us look at another example code to see where we use this statement:
Example
# Suppose this is file.py.
print("before importing")
import math
print("before defining function_a")
def function_a():
print("Function a")
print("before defining function_b")
def function_b():
print("Function b {}".format(math.sqrt(110)))
print("Before __name__ guard")
if __name__ == '__main__':
function_a()
function_b()
print("After __name__ guard")
Output:
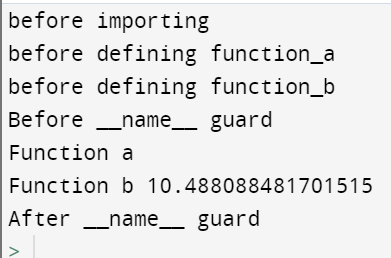
Need of if __name__==__main__
We have to call it out if we need to use a module by importing it from another one. Look at an example code.
Example
# Python program for execution
# function from directly
def MY_function():
print ("I am inside a function of a module")
# We can always test the function by calling it.
MY_function()
Output:

In this code, we called out a function to execute that part. But without calling the function, we can approach it by the following technique.
Technique:
# Python program for using
# main program for function call.
if __name__ == "__main__":
my_function()
import myscript
myscript.my_function()
Advantages of using if __name__==__main__
- For every python module, there will be a __name__ that is defined, and if this is the main program, then it is __main__, so this primary variable indicates that this program is being standalone by the user. This can also respond simultaneously to the appropriate sequence.
- Once you import this program into a module and then import it into the same program independently, the __name__ is set to be the module name you selected.
- All these python files can be used multiple times and also could be used as an independent program.
- Anytime a module is created, then that module will be a library. There is no need always to script it whenever it runs some units or other demos.
- Executing a code in python will set up a temporary variable and import the script.
Conclusion
In this article, we have covered a topic called if __name__==__main__ statement in python. So far, we have covered the definition of the if __name__==__main__ statement. Why does this statement work differently on different types of code? Application of this statement using example codes. Other ways of importing the module. The importance of this statement in the code and, finally, the advantages of this if __name__==__main__ statement.