How to reverse a string in python
How to reverse a string in python
A Brief About Strings-
- The String is a data type in Python that has a sequence of characters. This series of characters are represented in single, double or triple quotes. Python has a built-in string class called ‘str’.
For example-
Name=’Darcy’ Nationality=” British” Message= “Hello World”
- The characters of strings can be accessed using the indexes. The expression [ ] in brackets is called an index.
For example-
message = “TENET”
The characters of this string can be accessed as-
message [0] = T message [1] = E message [2] = N message [3] = E message [4] = T
- They are immutable which means they cannot be changed. A new string is created when we try to change or modify the existing string variable. Using id(), we can get the memory addresses of objects.
- The feature of slicing present in strings can help us to fetch the characters of our choice. The syntax of slice operation is s[start:end].
Start specifies the beginning index of the substring.
End specifies the last character index of the substring.
a='CORSICA' OUTPUT
print(a[1:5]) ORSI
print(a[1:]) ORSICA
print(a[:6]) CORSIC
- Regular Expressions are an influential tool for different kinds of manipulation of our strings. They can be accessed using the re module.
Regular expressions help us to match or find strings in another strings.
Some of its examples are-
- match() function- This function matches the pattern with a string. It gives the desired output only when the match is found at the beginning of string.
Syntax –
re.match(pattern,string,flags=0)
- search() function- This function matches the pattern with a string. The match of a pattern can occur anywhere in the string.
Syntax –
re.search(pattern,string,flags=0)
- sub() function- It is used to search a pattern and then it is replaced by another pattern.
Syntax –
re.sub(pattern,repl,string)
- findall() function- It searches a string and then returns a list of matches of the pattern in the string.
Syntax –
re.findall(pattern,input_str,flags=0)
Let’s understand our objective here,
If we have a string named ‘CORSICA’, we are supposed to obtain the reverse of this string in our output.
Input String – ‘CORSICA’
Output String – ‘ACISROC’
The different approaches that we can use to implement this are-
1. Using the for loop and concatenating the letters in reverse format and storing them in a variable.
2. Using the reverse() function.
3. Using the slicing operation
METHOD I
In python, for-loop helps us to pick each element from the string and perform the expected functionality on it according to the conditions.
So, let's have a glance on what's the step-by-step approach we'll use here-
- Take your input string.
- Now with the help of for loop, it will take each character from the string and append it in the variable and it will be appended in the reverse format.
- Now we will print the variable in which we have stored this value.
def reverse(string): revstr='' for i in string: revstr = i + revstr return revstr string = "CORSICA" print("Original string: ",string) print("Reverse string: ",reverse(string))

METHOD II
Functions in Python helps in the redundancy of our code and make it easy to comprehend.
Python is popular and well-accepted because of its versatile nature. The reverse() function helps us to obtain the reverse of the string.
Following are the steps-
- We will use the reverse() function inside the join function since join takes each iterable and converts it into a string.
- Then we call the function to display the desired result.
def reverse(str): string=' ‘. join(reversed(str)) return string my_string='CORSICA' print("Original string: ",my_string) print("Reversed string: ",reverse(my_string))
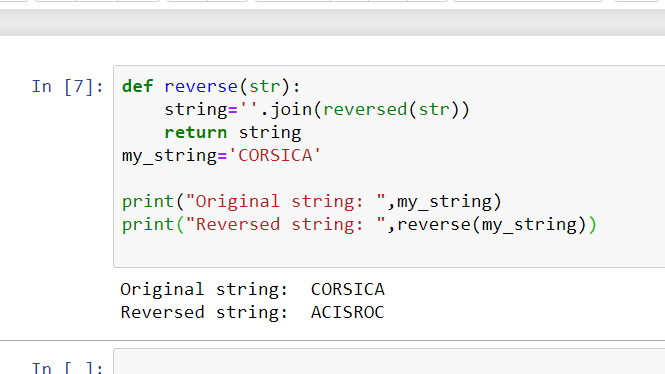
METHOD III
Slicing is a great technique when we want to play with the letters of our string. So here we have three parameters i.e., start, stop and step.
Let’s understand the procedure here-
The reverse of string can be obtained by initializing
Start as -1(since we have to start with the last character of the string)
Step as -1(Traversing in the backward direction)
And leaving the end as blank.
Again, we can call our function to display the required results.
def reverse(string): string=string[-1::-1] return string my_string='CORSICA' print("Original string: ",my_string) print("Reversed string: ",reverse(my_string))
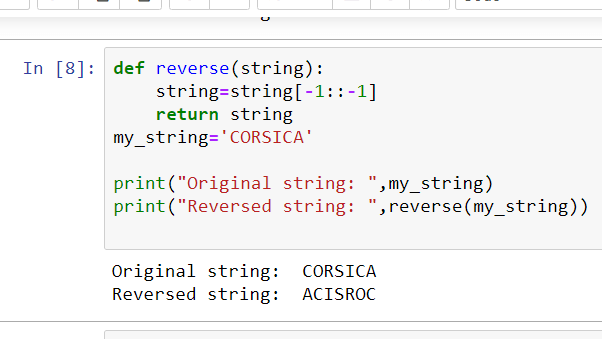