How to set font for Text in Python
As a tuple with the font family as the first component, a size in point as the second, and possibly a string with one or more of the style modifiers bold, italic, underlined, and overstrike as the third component.
Example
("Helvetica", "16") for a 16-point Helvetica regular.
("Times", "24", "bold italic") for a 24-point Times bold italic.
font object fonts
By importing the tkFont module and utilising its Font class function Object() { [native code] }, you may produce a "font object"
Here is a list of available options:
- Family is the name of the font family as a string.
- Size is an integer representing the font height in points. Use -n to produce a font that is n pixels high.
- "Bold" denotes boldface, whereas "Normal" denotes standard weight.
- "Roman" for unslanted and "italic" for italic is called slant.
- Underline 0 for regular text, and 1 for underlined text.
- overstrike 0 for regular text, 1 for overstruck text
X Window fonts
You can utilize any of the X font names if your operating system is Windows Operating System.
For instance, the developer prefers the fixed-width font "-*-lucidatypewriter-medium-r-*-*-140-*-*-*-*" for usage on screens. Have used the xfontsel application to aid with font selection.
font formats in X11
These bitmap font formats are supported in the X Window System.
- Binary distribution format is called(.bdf).
- The Portable compiled format is called as or (.pcf).
- The server's normal format is called(.snf).
As well as outline fonts upon certain systems.f3b (The exclusive outline font style from Sun Microsystems)
- .pfa (ASCII font for Adobe Type 1 PostScript)
- .pfb (Binary for Adobe Type 1 PostScript Font)
- .spd (Format for the Bitstream Speedo outline typeface)
Although the. p cf format has supplanted the. SNF format is now regarded as outdated; many local systems still utilize the. snf format.
font utilities
The programmes dxfc, bdftopcf, and bdftosnf can convert between some of these formats, but there aren't any converters for all possible combinations of font file formats.
Getbdf can get a typeface in. bdf format from the X server. This program enables conversion between any two formats, even if it does so indirectly through the X server, because there exist converters for this format to the other formats.
bdfresize allows you to scale a .bdf font logically. The output is a.bdf file similarly, but before using it, you must adjust the FONT line in the output file to modify the font name to match the new size.
An.snf file's contents can be printed using the shows command.
pdf format with mftobdf. may may convert to metafont fonts.
Any font may be read from a font server and produced in.bdf format using the fstobdf programme.
The xfd utility, for instance xfd -fn 10x20, shows a table of every character in a font.
All of the typefaces that the X server is aware of are listed by the xlsfonts function. This will yield a large list of 1000–2000 typefaces on many systems. If you use it, you should usually filter the output using a pager, such as less, more, or pg, or else with a pattern search tool, such as agrep, creep, ceegrep, grep, fgrep, grep, or strep.
Tkinter font wrapper
The Font class is available for generating and utilizing specified fonts in the Tkinter.font module.
The various font weights and slants are as follows:
tkinter.font.NORMAL
tkinter.font.BOLD
tkinter.font.ITALIC
tkinter.font.ROMAN
class tkinter.font.Font(root=None, font=None, name=None, exists=False, **options
The Font class represents identified fonts. Instead of identifying a font by its properties with each occurrence, Tk uses named fonts to create and identify fonts as a single object. Font instances are identified by their family, size, and style setting and assigned distinct identifiers.
Arguments:
font:-A tuple of font specifiers (family, size, options)
name:-distinct font name
exists:-If true, self points to a specified font.
actual(option=None, displayof=None)
The font's characteristics should be returned.
get(option)
The font's property may be retrieved.
config(**options)
Change the attributes of the font.
copy()
New instances of the current font should be returned.
measure(text, displayof=None)
Return the amount of space formatted within the supplied font that the text would take up on the chosen display. The default display is the main program window if none is provided.
metrics(*options, **kw)
Return font-specific data. Options consist of :
ascent : The length a font character can occupy from the baseline to its highest point.
descent : The smallest position a font character can occupy between the baseline and the top of the character
linespace: Minimum vertical distance between any two font characters is required to prevent vertical line overlap.
Fixed : 0 unless the typeface has a fixed width of 1.
tkinter.font.families(root=None, displayof=None)
Bring back the many font families.
tkinter.font.names(root=None)
Return the names of the defined fonts.
tkinter.font.nametofont(name, root=None)
For a tk-specified font, return a Font representation.
How can I change the text's font in Tkinter?
Tkinter is a GUI module offered by Python (Graphical User Interface). This tutorial will demonstrate how to change the text font in Tkinter.
Method 1: using the .configure() function with a tuple.
Approach for the above method:
- Bring the tkinter module in.
- Establish a GUI window.
- Make a text widget for us.
- Make a tuple with the font's specs in it. However, the order should be kept as follows while producing this tuple: (font family, font size in pixel, font weight). Font family, Font weight, and Font Size should all be given as strings and integers, respectively.
- Utilize the.configure() function to parse the specs into the Text widget.
The application of the abovementioned approach is shown below.
Example
# Bring up the Tkinter module
import tkinter
# Building the GUI window
root = tkinter.Tk()
root.title("python")
root.geometry("350x260")
# Our text widget being created.
sample_text = tkinter.Text( root, height = 15)
sample_text.pack()
# Building a tuple with
# the font's features
Font_tuple = ("Times new romen", 25, "bold")
# categorized the requirements into the
# utilising the .configure() function, a text widget.
sample_text.configure(font = Font_tuple)
root.mainloop()
Output
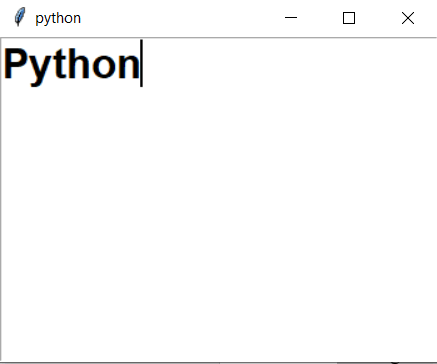
Method 2: The Font object of tkinter.typeface is used to change the font.
Approach for the above method:
- Tkinter module import.
- import the Tkinter font.
- Making the GUI window
- Establish our text widget.
- Using the tkinter.font module, create a Font-type object. In order to create this object, the function Object() { [native code] } asks for the appropriate font requirements (font family, font size in pixel, and font weight). The text widget needs this specific object in order to choose its font.
- The Text widget may be parsed using the.configure() function.
Example
# Module import
import tkinter
import tkinter.font
# The GUI window's creation.
root = tkinter.Tk()
root.title("javatpoint")
root.geometry("742x350")
# Our text widget being created.
sample_text=tkinter.Text( root, height = 15)
sample_text.pack()
# Generate a Font object using Tkinter.
Desired_font = tkinter.font.Font( family = "Times new romen",
size = 16,
weight = "bold")
# The Font object was parsed
# by use of the .configure() function, to the Text widget.
sample_text.configure(font = Desired_font)
root.mainloop()
Output
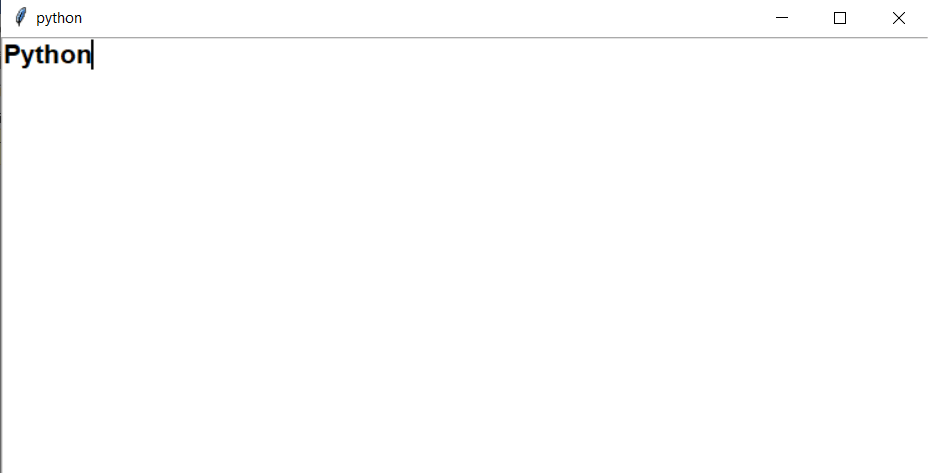