Python elif
Python elif
The elif statement is used to check multiple conditions and execute the specific block of statements depending upon the true condition among them.
Syntax
if expression1: statement elif expression2: statement elif expression3: statement else: statement
The elif statement can be optional such as else. When the if expression is true, the statement of expression 1 will be printed otherwise control moves to elif expression, if that elif condition evaluates false then control transfers to next elif. It will check all elif expression until it gets a true expression.
Note: Python does not provide a switch or case statement as other programming languages
Flow diagram
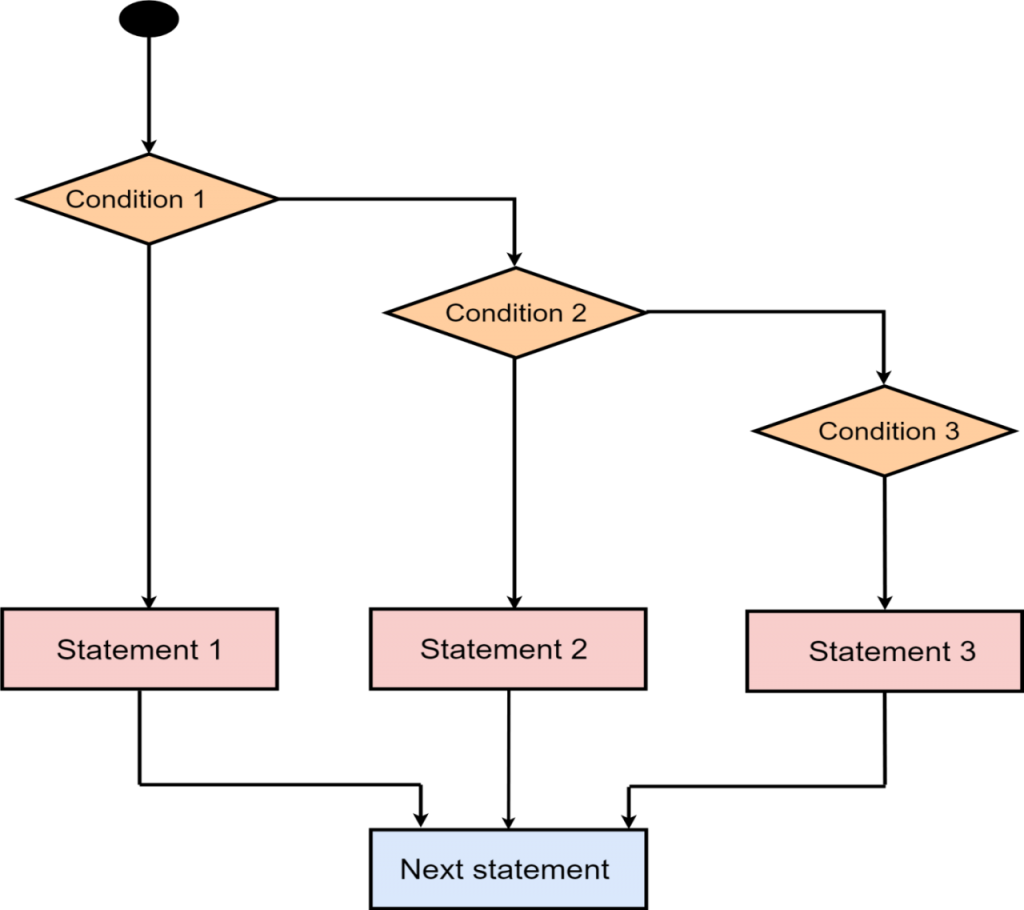
In the above flow diagram, Python evaluates each expression (i.e., the condition) one by one and if a true condition is found the statement block under that expression will be executed. If the false condition is detected, then statement block under else condition will be executed. In the following example, we have applied if, series of elif, and else to get the type of a variable.
1. Write a program to check the grade of the student
marks = int(input("Enter the marks "))
if marks >85 and marks <= 100:
print("Congrats ! you scored grade A ")
elif marks >60 and marks <= 85:
print("You scored grade B + ")
elif marks >40 and marks <= 60:
print("You scored grade B ")
elif marks >30 and marks <= 40:
print("You scored grade C ")
else:
print("You are fail ")
Output:
Enter the marks 60 You scored grade B
2. Write a program to check whether its morning, noon, or night.
time= int(input("Enter the time"))
if time >= 6 and time <12:
print("Good Morning")
elif time == 12:
print("Good Noon")
elif time >12 and time <= 17:
print("Good Afternoon")
elif time >17 and time <= 20:
print("Good Evening")
elif (time >20 and time <=24) or (time >= 0 and time <=6):
print("Good Night")
else:
print("Invalid time!")
Output:
Enter the time12 Good Noon
3. Write a program of the simple calculator
a=int(input("Enter the first number")) b=int(input("Enter the second number")) print("1. Add") print("2. Sub") print("3. Mul") print("4. Div") choice=input("Enter your choice") if(choice=='1'): print("The addition is:",a+b) elif(choice=='2'): print("The subtracion is:",a-b) elif(choice=='3'): print("The multiplication is:",a*b) elif(choice=='4'): print("The divide is:",a/b) else: print("Enter the valid choice")
Output:
Enter the first number:30 Enter the second number:20 1. Add 2. Sub 3. Mul 4. Div Enter your choice:3 The multiplication is: 600
4. Write a program to check the type of triangle.
a=int(input("Enter the first angle:"))
b=int(input("Enter the second angle:"))
c=int(input("Enter the third angle:"))
if a<b+c or="" b<a+c="" c<a+b:="" print("the="" triangle="" does="" not="" exist")="" elif="" a="=b" and="" #an="" equilateral="" has="" three="" angles="" of="" 60°,="" equal="" sides.="" print("equilateral="" triangle")="" b="=c" c="=a:" #="" an="" isosceles="" two="" that="" are="" equal,="" print("isosceles="" else:="" obtuse="" one="" only="" angle.="" print("obtuse="" <="" pre=""></b+c>
Output:
Enter the first angle: 60 Enter the second angle:60 Enter the third angle:45 Isosceles triangle