Python Variable Scope with Local & Non-local Examples
This article will examine Python's global, local and non-local variables and show you how to use them to write code without problems.
Let's quickly review what a variable in Python is and how variable scopes work before we go into the how and why of using global and non-local variables in your functions.
What is a Variable in Python?
A label or name provided to a specific area in memory is known as a variable. The value you want your software to save to use later is stored in this place. The beautiful thing about Python is that you can define any variable type without specifying it. You may assign the desired value to a new variable in Python using the assignment operator (=, a single equals sign).
The variable name appears to the left of the assignment operator, and its value appears to the right. The assignment will be assessed if the right-hand side of the equation is an arithmetic operation.
There are some rules in Python for creating variables are given below:
- Only letters (uppercase or lowercase), numerals, and the underscore character are permitted.
- A variable cannot begin with a numeric value.
- A variable may not be a keyword in Python.
Let’s take an example to understand variables in python given below:
Example of valid variable names in python
string_variable = "Hello World!"
int_variable = 56
float_variable = 5.63
Example of invalid variable names in python
string variable = "Hello World!"
56int = 56
float-variable = 5.63
Variable Scope in Python
Variables in Python provide the storage areas for data values. When a variable is assigned to an instance, it is mapped to that instance since they are reference, or pointers, to an object in memory.
Python is not "statically typed," in contrast to languages like C/C++/JAVA. Variables must not have their types or initial values declared before use. When a variable is initially given a value, it is considered to have been formed.
Variables defined inside functions and classes (instance variables) are often local by default, but variables defined outside these structures are typically global.
Its scope refers to the area of a program where a variable is reachable. The LEGB rule is based on the four main categories of variable scope. Local -> Enclosing -> Global -> Built-in is referred to as LEGB.
The scope of a variable in Python refers to the area where we may locate it and, if necessary, access it.
Local Scope in Python
When a variable is defined inside a function, its scope is LIMITED to the function. This implies that neither its value nor access outside the function is possible. It exists as long as the function is running and is available from the time it is defined until the end of the function (Source).
The variables defined in the function are variables with a local scope. The function body contains a definition for these variables.
Let’s understand Local scope in Python by taking the examples given below.
Example 1 of Local Scope in Python
# defining a function name as fun
def fun():
x = "Hi World!"
print("printing value of x from inside of function fun()", x)
# calling the function
fun()
print("printing value of x from outside of function fun()", x)
Output

The above example shows a variable x inside the function fun(). When we call the print function inside the fun(), it prints the value of x, but when it is called outside the fun function, we get an error “NameError: name 'x' is not defined”. Hence x is a local variable of function fun. We cannot access x from outside of the function body.
Example 2 of Local Scope in Python
#defining a function name as fun1
def fun1():
x = "Hi World!"
#defining a function name as fun2
def fun2():
y = "Hello"
print("printing value of y from inside of function fun2()", y)
fun2()
print("printing value of x from inside of function fun1()", x)
print("printing value of y from inside of function fun1()", y)
#calling the function
fun1()
print("printing value of x from outside of function fun1()", x)
print("printing value of y from outside of function fun1()", y)
Output
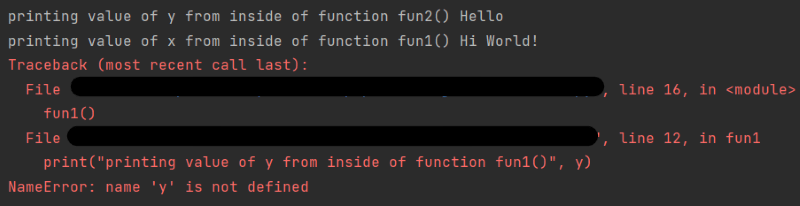
In the above example, we have 2 nested functions, fun1() and fun2(). In fun1() function we have a local variable x and inside fun1() function we have fun2() function. fun2() function has a local variable y.
When we call the fun2() function, it prints the value of y, and when we call the fun1() function, it prints the value of x and tries to print the value of y also. But y is not a local variable to fun1(). The scope of y is inside the fun2() function, so when we try to print it outside the fun2() function, we get an error “NameError: name 'y' is not defined.”
Also, variable x has scope inside the fun1(), so we cannot print x outside the fun1() function.
Global Scope in Python
Possibly the simplest scope to comprehend is this one. A variable becomes a global variable whenever defined outside any function, and its scope is the entire program. This implies that it can be applied to any function.
A global scope variable can be read from anywhere in the program. We can access these variables both inside and outside of the code. We declare a variable as global when we intend to use it across the rest of the program.
Let’s understand the Global scope in Python by taking the example below.
Example 1 of Global Scope in Python
#defining a function name as fun1
def fun1():
print("printing value of x from inside of function fun1()", x)
#defining a function name as fun2
def fun2():
x = 5
print("printing value of x from inside of function fun2()", x)
#defining a function name as fun3
def fun3():
x += 11
print("printing value of x from inside of function fun3()", x)
x = 9
#calling the function fun1
fun1()
print("printing value of x from outside of functions", x)
#calling the function fun2
fun2()
print("printing value of x from outside of functions", x)
#calling the function fun3
fun3()
print("printing value of x from outside of functions", x)
Output
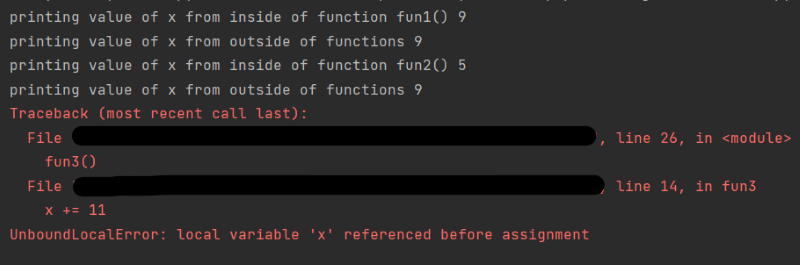
In the above example we have 3 functions fun1(), fun2(), and fun3(). fun1() function does not have any local variable. When we try to print the value of the global variable, it prints the value of x. Hence global x has scope inside function also.
Inside the function fun2(), we try to reassign the value of x and print it. So fun2() prints the new value of x. But when we print the value of x outside the function fun2(), it does not change. Hence x inside fun2() is not the global x. It is a different local variable x.
Inside the function fun3(), we try to modify the value of x using the += operator to get an error “UnboundLocalError: local variable 'x' referenced before assignment”. Hence the value of a global variable we cannot change directly.
We must utilize the global keyword to make the program mentioned above function. We need to utilize the global keyword in a function when making assignments or to change them. For printing and accessing, global is not necessary. Why? Due to the assignment to x inside of fun(), Python "assumes" that we want a local variable, which is why the initial print command returns this error. It is considered local if a variable is modified or created inside a function without being defined as a global variable.
Global keyword in Python
The global keyword in Python enables you to change a variable outside the current scope. It is used to construct a global variable and make local adjustments to the variable.
Let’s understand the rules for the global keywords in python as given below:
Rules for Global Keywords in Python
- A variable is, by default, considered global when defined outside a function. The usage of global keywords is not required.
- A variable is local when it is created inside a function.
- The global keyword reads and writes global variables inside a function.
- The global keyword has no impact when used outside of a function.
The following example demonstrates how to use the global keyword to instruct Python that we want to use the global variable:
Example of Global Keyword in the Python:
def fun1():
global x
x = 5
print("printing value of x from inside of function fun1()", x)
def fun2():
global x
x += 11
print("printing value of x from inside of function fun2()", x)
x = 9
print("printing value of x before calling fun1() function", x)
fun1()
print("printing value of x after calling fun1() function and before calling fun2() function", x)
fun2()
print("printing value of x after calling fun2() function", x)
Output

In the above example, we have 2 functions, fun1() and fun2().
Inside the function fun1(), we try to reassign the value of x and print it. So first, we will use the global keyword in fun1(), then assign the new value of x. It prints the new value of x. It also changed when we printed the value of x outside the function fun1(). Hence x inside fun1() is the global x.
Inside the function fun2(), we try to modify the value of x using the += operator. It prints the modified value of x. First, we use the global keyword then we print the value of x inside the function. Outside of the function fun2, when we print the value of x, we see that the value of global x is modified.
Non-local keyword
In Python, nested functions are handled with the nonlocal keyword. This keyword functions similarly to global, but in the case of nested functions, it declares a variable to point to the variable of the outer enclosing function rather than global.
In nested functions with an undefined local scope, nonlocal variables are used. This indicates that the variable cannot be both local and global in scope.
To make nonlocal variables, we employ nonlocal keywords.
NOTE: Changes introduced to a nonlocal variable's value appear in the local variable.
Let's look at a Python usage example for a nonlocal variable.
Example:
def fun1():
x = 5
print("printing value of x from inside of function fun1() before calling fun2", x)
def fun2():
nonlocal x
x += 11
print("printing value of x from inside of function fun2()", x)
fun2()
print("printing value of x from inside of function fun1() after calling fun2", x)
x = 9
fun1()
print("printing value of x after calling fun1() function", x)
Output

The above example shows a global variable x and a function fun1(). Function fun1() also has a local variable named x and another function fun2(). In function fun1(), we print the value of x and get the value of local x. In function fun2(), we try to change the value of x, which is local to fun1(). So we used the nonlocal keyword and modified the value of x.
LEGB Rule in Python
A Python interpreter will follow the LEGB (Local -> Enclosing -> Global -> Built-in) logic when running your application.
Assume you're calling print(x) inside of inner(), a function that is nested inside of outer (). Python will then check if "x" was locally declared within inner (). If not, the outer() variable will be applied. The enclosing function is this. If it is also not specified there, the Python interpreter will move up a level to the global scope. Only the built-in scope, which has exclusive variables for Python itself, is present above that.
Conclusion
You understand the LEGB rule, the Python variables' scope, and the proper usage of the global and nonlocal keywords. I routinely use these terms.