Python Dictionary
Dictionary in Python is an unordered collection of data values, which is used to store data values like a map. Unlike different data types that hold just individual assets as a component, Dictionary holds the key: value pair. Key: Value is given in the Dictionary to make it more advanced and useful.
It is generally used when we have a large amount of data. It is a necessary to know the key to restoring its value. A dictionary key can be almost any Python data (except a list) but is usually preferred as a number or string. Dictionaries are defined in braces, with each item separated by key: value. In one Dictionary, we can have different types of keys and values. Word recommendations are advanced to recover values when the key is known. Python dictionary gives us many other interesting strategies assisting us while performing tasks.
Example
d={1:"abc",2:"xyz","pqr:10"}
Creating a dictionary in python
Creating a dictionary is simple: just putting data inside curly braces {} separated by commas. A data has a key and values; while the qualities can be of any data or information type and can review, keys should be of unchanging kind (string, number, or tuple with permanent components) and should be exceptional.
NOTE: Dictionary keys are case sensitive, a similar name yet various key occurrences will be dealt with particularly.
#creating an empty dictionary
d1=dict{}
This is how we can create an empty dictionary.
Example
info = {'name': 'Md Shibbu', 'age': 19, 'location': 'Noida'}
print(info)
print(type(info))
Output
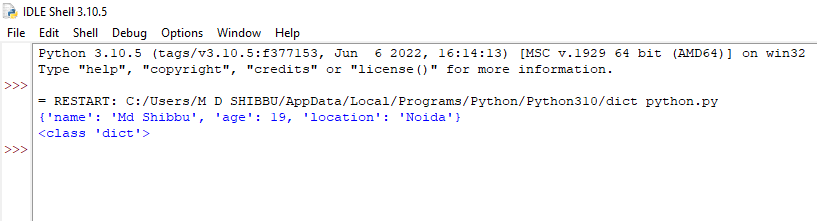
Example 2
k = ['rupees', 'pencils', 'copies', 'mugs'];
v = [13, 4, 39, 7];
items = dict(zip(k, v))
print(items)
Output
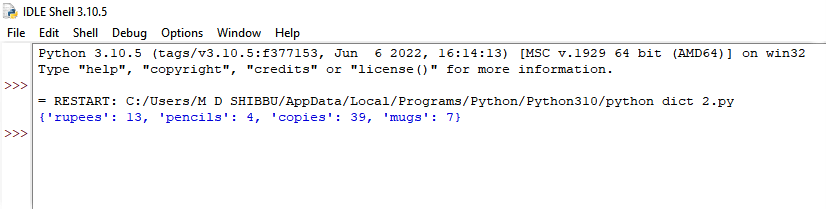
Addicting elements to a dictionary
In Python Dictionary, the extension of components should be possible in more than one way. Each value, in return, can be added to a Dictionary by identifying values alongside the key, for example, Dict[Key] = 'Value'. Settled key values can also be added to a current Dictionary. Refreshing a current value in a Dictionary should be possible using the implicit update() method.
Example
d = {'India':200,'Nepal':300,'Bhutan':400}
print("Country Name",d)
d.update({'India':500})
print("updated country name",d)
Output
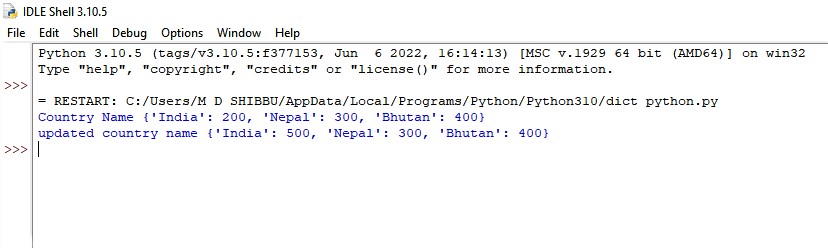
Example 2
d = {'Shyam':200,'Rahul':300,'Rohit':400}
print("Name",d)
d.update({'Rahul':600,'Rohit':900})
print("updated name",d)
Output
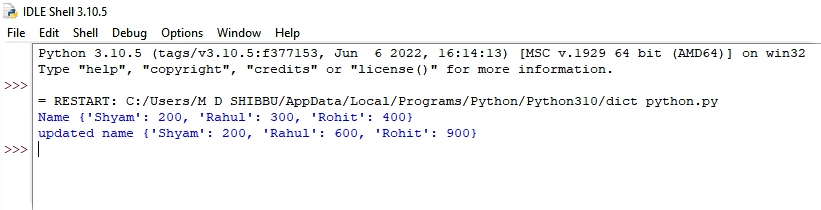
NOTE: If the key is already present, the current value gets refreshed. A new (Key: value) match is added to the Dictionary if the key is absent.
Removing an element from a dictionary in python
We know that Dictionary is mutable; we can eliminate a specific thing in a dictionary using the pop() method. This method eliminates a thing with the given key and returns the value. The pop item() method can eliminate and return a changeable (key, value) thing pair from the Dictionary. All things can be eliminated immediately by using the del() method. We can utilize the del()keyword to eliminate individual things or the whole Dictionary itself.
Example
bdict = {'a': 'anil', 'b': 'baibhav', 'c': 'crypto', 'd': 'daniel'}
print(bdict)
ae =bdict.pop('c')
print(bdict)
print('Removed element: ', ae)
Output
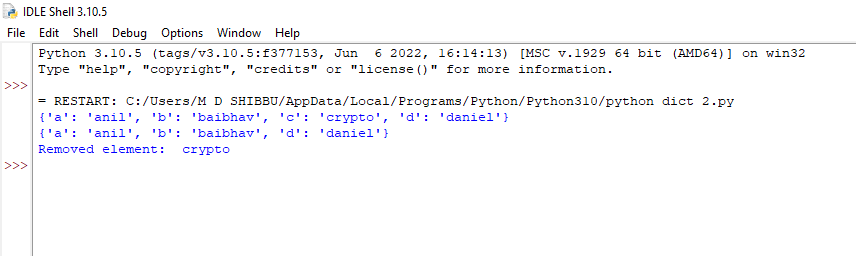
Example 2
bdict = {'a': 'Amir', 'b': 'Baibhav', 'c': 'crypto', 'd': 'daniel'}
print(bdict)
if "c" in bdict:
del bdict["c"]
print("Updated Dictionary :", bdict)
Output
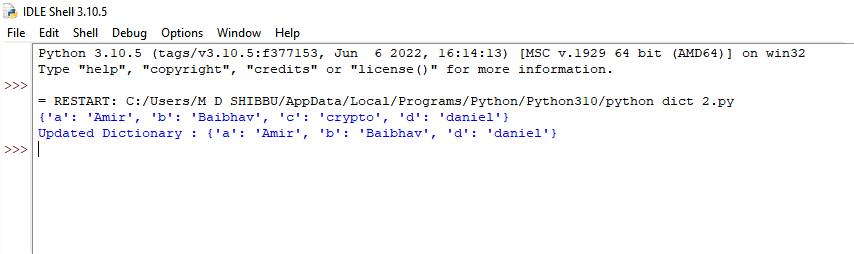
Methods in dictionary in python
In Python, as with strings and lists, a few implicit techniques can be appealed on word references. Now and again, the overview and word reference techniques share a similar name. In the conversation on object-oriented programming, you will see that it is completely ok for various kinds to have approached with a similar name.
clear()- Eliminate every element from the dictionary
copy()- Returns a duplicate value of the Dictionary
get() - Returns the value of determined key
items() - Returns an overview containing a tuple for each key-value pair
keys() - Returns an overview containing the Dictionary's keys
Pop() - Remove the item and stores it for future use
pop item() - Removes the last inserted key-value pair in dictionary
update() - Updates dictionary with determined key-value pairs
values() - Returns an overview of the values of the Dictionary
NOTE: The .things(), .keys(), and .values() methods really return something many refer to as a view object. A dictionary view object is like a window on the keys and values. For down-to-earth purposes, you can consider these techniques returning arrangements of the Dictionary's keys and values.
Conclusion
In Python,Lists and dictionaries are two of the most often utilized Python types. In Dictionary, data and word references are two of the most often utilized Python types. As you have seen, they have a few similarities yet differ in how their components are used. Data and word references will generally be fitting for various conditions.