Python List
The list is one of the most versatile, mutable data-structures in Python. It can store heterogeneous data or different types of data. The list contains comma-separated values (item) within the square brackets. Creating a list in Python is simple as follows:
list1 = [‘Abhay’,’Arjun’,’Himanshu’,’Anubhav’] list2 = [1,2,3,4,5,6] list3 = [‘Himanshu’,20, 54.6,’Abhay’, ’a’, ’b’]
Indexing and Slicing in List
Indexing in List:
Indexing of list can be done by using [] operator.
list =[ 'P', 'Y' , 'T' , 'H' , 'O' ,N’]

The values in the list can be accessed by its index position. The index starts from 0 to n-1. The first element is stored in the 0th index, and the second element is stored in the first index and so on.
The rightmost index position is -1, and its adjacent left index is -2 and so on. For example:
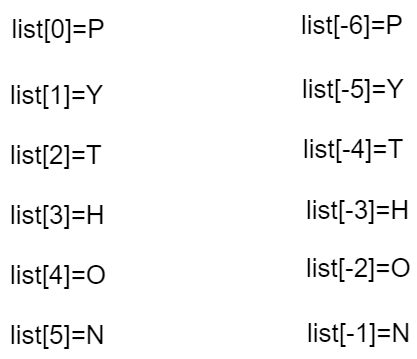
Slicing in List
Python provides a facility to get a sub-list of the list. Sometimes we require only some data from a given list then slicing makes it happen. The syntax is:
list_variable[start: end: step]
start
refers to the starting position of the list element.stop
refers to the index of the element where we should stop just before to finishing of our slice.step
allows you to take each nth-element within a start:stop range.
Let's look at this concept using below example:
Example-1:
list = [10,20,30,40,50,60,70,80,90] print(list[2:6])
Output:
[30, 40, 50, 60]
In the above example, the start is equal to 2, so slicing starts from the second position. The value at index 2 is 30.
The stop indicates the last element of the list, and its value is 60. Hence it will stop just before to the stop index.
The steps permit to take each nth-element within a start: stop range.
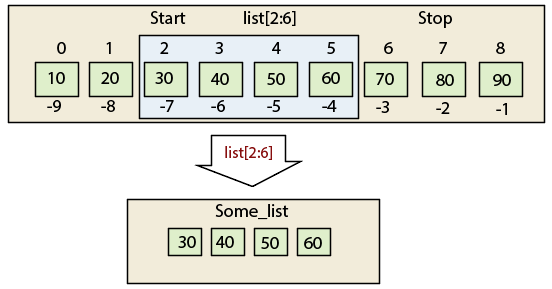
We can skip the start index and then it will starts from 0 by default. For example:
list = [10,20,30,40,50,60,70,80,90] print(list[:4])
Output
[10, 20, 30, 40]
So, list[:4] is equivalent to the list[0:4]. It is a shortcut to extract first nth element
We can skip the stop index also. It will return last n elements of the given list. For example:
list = [10,20,30,40,50,60,70,80,90] print(list[4:])
Output
[50, 60, 70, 80, 90]
In the above example, we skip the stop index that’s mean it takes elements from the start position to end of the list.
Example-2:
list = [10,20,30,40,50,60,70,80,90] print(list[::]) #By default step-size is 1. There is no need to specify it print(list[::2]) # Here step-size is two print(list[1::3]) print(list[2:7:2])
Output:
[10, 20, 30, 40, 50, 60, 70, 80, 90] [10, 30, 50, 70, 90] [20, 50, 80] [30, 50, 70]
In the above program, we can omit start and stop the argument and use only step argument. By providing step, we can skip some elements.
Accessing elements of list using negative indexing
list = [10,20,30,40,50,60,70,80,90] print(list[-3:]) #The index position is count from backward. start is -3 and it will proceed to last index of list print(list[1:-1]) print(list[-5:-2]) # Here step-size is two print(list[-3:]) print(list[1:-3:2]) print(list[:7:-2]) print(list[::-1]) # step-size is -1.it will reverse entire list
Output:
[70, 80, 90] [20, 30, 40, 50, 60, 70, 80] [50, 60, 70] [70, 80, 90] [20, 40, 60] [90] [90, 80, 70, 60, 50, 40, 30, 20, 10]
Modification (update) in List
Lists are mutable; it means we can modify the elements of the list using the slice and assignment operator. The append() function of list is also used to add value in list.
list = [10,21,30,45,50,63,78,80,90] list[0]=1 print(list) list[1:4]=[2,3,4] print(list) list[2]= 'Himanshu' print(list) list[3:5]='abc' print(list)
Output:
[1,21, 30, 45, 50, 63, 78, 80, 90] [1, 2, 3, 4, 50, 63, 78, 80, 90] [1, 2, 'Himanshu', 4, 50, 63, 78, 80, 90] [1, 2, 'Himanshu', 'a', 'b', 'c', 63, 78, 80, 90]
Deletion in List
The del keyword is used to delete the element of list. It is used when we know exactly which element needs to be deleted. The remove () or pop () method can also be used for deleting the element. For example:
list = [10,21,30,45,50,63,78,80,90] del list[0] print(list) del list[1:4] print(list) del list[::2] print(list)
Output:
[21,30, 45, 50, 63, 78, 80, 90] [21, 63, 78, 80, 90] [63, 80]
We can also delete the entire list. As per the following example:
list = [10,21,30,45,50,63,78,80,90] del list #TypeError because list is deleted and we are accessing the first element of list print(list[0])
Output:
TypeError:'type' object is not subscriptable
Basic Operations in List
There are various operations in the list as follows.
- Repetition
The repetition operator (*) is used to repeat list elements for multiple times.
list1 =[1,2,3,4,5] print(list1*2)
Output:
[1, 2, 3, 4,5, 1, 2, 3, 4, 5]
- Concatenation
The concatenation operator(+) is used to concatenate two or more list.
list1 =[1,2,3,4,5] list2 =[6,7,8,9,10] print(list1+list2)
Output:
[1,2, 3, 4, 5, 6, 7, 8, 9, 10]
- Iteration
The list can be iterated using for loop
list1 =[1,2,3,4] for i in list1: print(i)
Output:
1 2 3 4
- Membership
If a particular item exists in a particular list than It returns true otherwise false.
list1 =[1,2,3,4] print(3 in list1)
Output:
True
Built-in functions in List
Sr. no. | Functions | Description |
1. | len(list) | It is used to compute the length of list |
2. | cmp(list1, list2) | It compares the elements of both the lists. |
3. | max(list) | It returns the maximum element of the list. |
4. | min(list) | It returns the minimum element of the list. |
5. | list(seq) | It changes any sequence to the list. |