Python Program to Find the Greatest Among Three Numbers
Python Program to find the greatest among three numbers
We have many approaches to get the largest of three numbers, and here we will discuss a few of them. This python program needs the user to enter three numbers. Next, Python will compare these numbers and print the greatest among them.
Input: A = 4, B = 6, C = 8 Output: C is the greatest
Method 1: By using Python Elif Statement
#Program to find the greatest among three #Take three number from user to compare num1 = int(input("Enter first number: ")) num2 = int(input("Enter second number: ")) num3 = int(input("Enter third number: ")) #compare the number if (num1 > num2 and num1 > num3): print("{0} is Greater Than both {1} and {2}". format(num1, num2, num3)) elif (num2 > num1 and num2 > num3): print("{0} is Greater Than both {1} and {2}". format(num2, num1, num3)) elif (num3 > num1 and num3 > num2): print("{0} is Greater Than both {1} and {2}". format(num3, num1, num2)) else: #Print the result print("Either any two values or all the three values are equal")
Output
CASE 1:
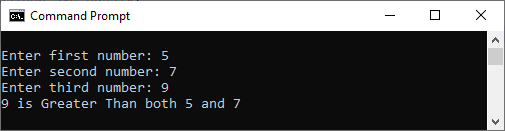
CASE 2:
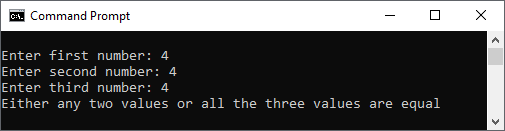
Explanation: In this program, a user will be asked to enter three numbers. This number will be stored in a separate variable. Now, we will compare all three numbers against each other using if...elif...else statement. After the comparison, the greatest among the three numbers will returns and prints on the screen.
Method 2: By using Nested If Statement
This program uses the nested IF statement to find the Largest of Three numbers in Python:
#Program to find the greatest among three #Take three number from user to compare num1 = int(input("Enter first number: ")) num2 = int(input("Enter second number: ")) num3 = int(input("Enter third number: ")) #compare the number if (num1-num2 > 0) and (num1-num3 > 0): print("{0} is Greater Than both {1} and {2}". format(num1, num2, num3)) else: if(num2 - num3 > 0): print("{0} is Greater Than both {1} and {2}". format(num2, num1, num3)) else: print("{0} is Greater Than both {1} and {2}". format(num3, num1, num2))
Output
Here is the output:
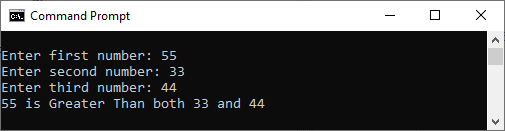