Buffer reader to read string in Java
The Buffered Reader class of Java is used to read the stream of characters from the input stream.
Program to read string using Buffer reader
import java.io.*;
class Demo
{
public static void main(String s[])
{
int a=1; // Here “a” assigned to 1.
System. out. println (“a = “+a);
}
}
Output
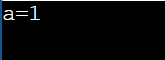
Explanation
The variable was assigned the value before running the above program. This way is called the static way.
In Java, to read inputs, there are 2 ways
1. Scanner Class
2. Buffer Reader
Buffered Reader
- Using this class, we can read data from the input stream.
- This class inherits the properties of the Reader class.
Syntax for object creation In Input Stream
Input Stream objectname = new FileInputStream();
Buffered Reader class Syntax
public class Buffered Reader extends Reader;
Constructors in Buffered Reader
- Buffered Reader(Reader r): It is used to generate an Input stream by considering a default size.
- Buffered Reader(Reader r, int size): It generates an Input stream with the exact size, passed as a parameter.
Methods in Buffered Reader class
- read ()
- close ()
- read (char[] c, int start, int length)
- ready ()
- mark ()
- reset ()
- mark supported ()
read() method
- It is used to read single characters from the stream.
- It returns -1 if it reaches the end of the input.
- It represents whether the next character is present or not.
- The return type of the read() method is int(Integer).
Syntax:
public int read()
Program
import java.io.*;
public class Main
{
public static void main(String[] args) throws IOException
{
Buffered Reader object2 = new BufferedReader(new InputStreamReader(System.in));
System. out. println("enter the string");
char s1 = object2.read();
System. out. println(s1);
}
}
Output
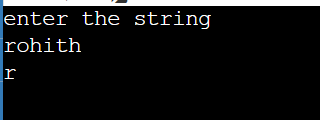
readLine() method
- It is used to read the entire line of the input.
Program
import java.io.*;
public class Main
{
public static void main(String[] args) throws IOException
{
BufferedReader object2 = new BufferedReader(new InputStreamReader(System.in));
System.out.println("enter the string");
String s1 = object2.readLine();
System.out.println("The string is");
System.out.println(s1);
}
}
Output
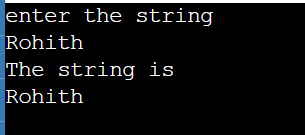
close () method
- Close method is used to close the character stream.
- If the stream has been closed, performing methods like reading (), readLine and other methods throw an IOException.
read(char[] c, int start, int length)
- It stores the reading characters in the array form.
- It returns the count of characters.
Syntax:
public int read(char[] c, int start, int length)
skip (long s) method
This method returns the input that matches the specified pattern, ignoring delimiters.
Syntax:
public long skip(String s)
Program
import java.util.*;
import java.util.regex.Pattern;
class Main {
public static void main(String args[]){
Scanner scan = new Scanner("Hello! Everyone");
scan.skip(Pattern.compile("..llo"));
System.out.println("Remaining Stringi s: " +scan.nextLine());
scan.close();
}
}
Output:

ready() method
- It checks whether the input stream is ready to be read.
- Return ready() method is boolean.
Syntax:
public boolean ready();
Program
import java.io.*;
import java.util.*;
class Main {
public static void main(String[] args)
{
try {
String str = "Java Language";
// create reader instance
Reader reader= new StringReader(str);
System.out.println("Is Reader ready "+ "to be read: "+ reader.ready());
// check whether it is ready or not
reader.close();
}
catch (Exception e) {
System.out.println(e);
}
}
}
Output

mark(int Limit)
- This method is used to represent the present position in the stream.
- Void is the return type.
Syntax:
public void mark(int Limit)
Program:
import java.io.*;
class Example17{
public static void main(String[] s) throws IOException{
object1 = new FileInputStream(“Fruit.txt”);
BufferedReader b = new BufferedReader(new InputStreamReader(object1));
b . mark(1);
System . out . println(b.read());
b . close();
}
}
Output

In the fruits file, we have content as Apple.
reset ()
- It is used to reset the stream.
- Void is the return type.
Program
import java.lang.*;
import java.util.concurrent.atomic.DoubleAdder;
public class Main {
public static void main(String args[])
{
DoubleAdder number = new DoubleAdder();
number.add(3);
// reset operation on variable number
number.reset();
// Print after add operation
System.out.println(" the current value is: " + number);
}
}
Output:
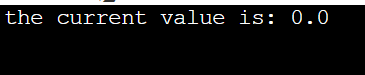
mark supported () method
- It is used to check whether it supports the mark() method.
- void is the return type.
Syntax:
public void markSupported()
Program
import java.io.ByteArrayInputStream;
import java.io.IOException;
public class Main {
public static void main(String[] args) throws IOException {
byte[] b = {1,2,3,4,5};
ByteArrayInputStream ba = null;
ba = new ByteArrayInputStream(b);
boolean isMarkSupported = ba.markSupported();
System.out.println("Is mark supported : "+isMarkSupported);
}
}
Output
