Rectangular Numbers in Java
In this tutorial, we will understand the meaning of a rectangular number in Java with the aid of examples, illustrations, and implementations. It is one of the popular coding interview questions.
Meaning
Rectangular numbers are known by other names such as -Pronic Number, oblong number, rectangular number, or heteromecic number.
A rectangular number is a special numberthat is the product of two consecutive integers, that is, n and (n + 1). The product can be expressed as n*(n+1)
It can also be understood that rectangular numbers are those numbers that can be arranged to form a rectangle.
Examples:
Some of the initial pronic numbers include: 0, 2, 6, 12, 20, 30, 42, 56, 72, 90, 110, 132, 156, 182, 210, 240, 272, 306, 342, 380, 420, 462…..
2 is considered the pronic number because it is the product of 2 consecutive numbers.
2 = 1 * 2
It is forming a rectangle of 1 row and 2 columns.
Similarly, 6 is considered the pronic number because it is the product of 2 consecutive numbers.
6 = 2 * 3
It is forming rectangle of 2 row and 3 columns.
Similarly, 12 is considered the pronic number because it is the product of 2 consecutive numbers.
12 = 3 * 4
It is forming a rectangle of 3rows and 4 columns.
Similarly, 30 is considered the pronic number because it is the product of 2 consecutive numbers.
30 = 5*6
Here, it is forming a rectangle of 5rows and 6 columns.
Similarly, 56 is considered the pronic number because it is the product of 2 consecutive numbers.
56 = 7 * 8
It is forming a rectangle of 7 rows and 8 columns.
42 is considered the pronic number because it is the product of 2 consecutive numbers.
42 = 6 * 7
It is forming a rectangle of 6rows and 7 columns.
72 is considered the pronic number because it is the product of 2 consecutive numbers.
72 = 8 * 9
It is forming a rectangle of 8rows and 9 columns.
0 is considered the pronic number because it is the product of 2 consecutive numbers.
0 = 0 * 1
Numbers those are not rectangular
3 is not considered as a rectangular number because it is not the product of 2 consecutive numbers.
3 = 3 * 1
Here, 1 row and 3 columns are being formed. Thus, the criteria for being a rectangular number get failed. So, it is not a rectangular number.
5 is not considered as a rectangular number because it is not the product of 2 consecutive numbers.
5 = 5 * 1
In the same way, here 1 row and 5 columns are being formed, violating the criteria of a rectangular number. So, it is also not a rectangular number.
7 is not considered as a rectangular number because it is not the product of 2 consecutive numbers.
7 = 7 * 1
In the same way, here 1 row and 7 columns are being formed, violating the criteria of a rectangular number. So, it is also not a rectangular number.
9 is also not considered as a rectangular number because it does not form a rectangle with consecutive numbers of rows and columns.
Implementation
Now, we will see a java code to understand the meaning of the rectangular number in a more practical way.
Code
//A program to check whether an entered no. is pronic or not
import java.util.*;
class RectangularNumber
{
public static void main(String args[])
{
Scanner sc = new Scanner(System.in);
System.out.print("Enter any number : ");
int num = sc.nextInt();
int flag = 0;
for(int j=0; j<num; j++)
{
if(j*(j+1) == num)
{
flag = 1;
break;
}
}
if(flag == 1)
System.out.println(num+" is a Rectangular Number.");
else
System.out.println(num+" is not a Rectangular Number.");
}
}
Outputs:
1.
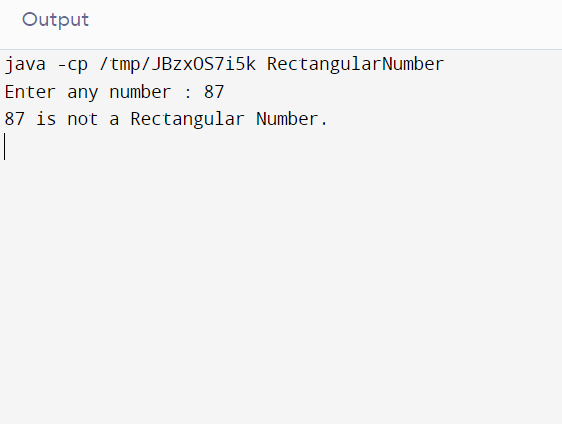
Explanation: The no. 87 is checked and is found that it is not a rectangular number.
2.
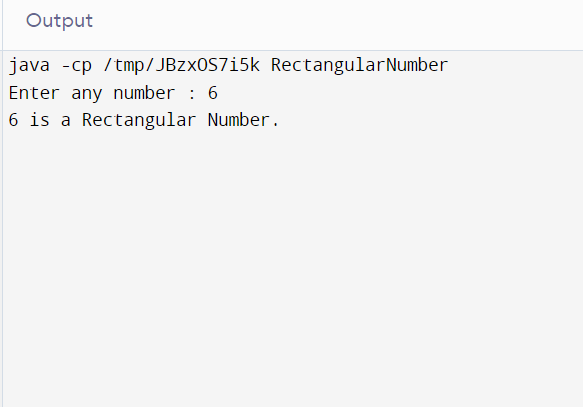
Explanation: The no. 6 is checked and is found that it is a rectangular number
Summary
In this tutorial, we tried to understand what is meant by rectangular number, saw a good number of examples, and further saw its implementation in java.