List of Constants in Java
In this tutorial, we are going to deal with constants available in Java.Every programming language has its constants. Similarly, Javaalso has got constants of its own.
In this tutorial, we will discuss what is meant bya constant in java, and various types of constants in java. We will deal with each of these constants - their syntaxes, and examples. We will sum up our understanding through a java program.
List of Constants in Java
Constants
It refers to the value that is impossible to change the valueif it gets assigned. In Java, there is a special keyword called final to define the constant values. It is used to denote that the value of the variable written after the final keyword cannot be changed or altered. It is to be kept in mind that the identifier name must be in capital letters as we know constants can also be defined as static.
Syntax:
static final data_type identifier_name = value of identifier;
Examples:
- static final double PI = 3.14;
- static final int RADIUS = 5;
- static final double SIDE = 7;
- static final int SALARY = 50000;
Points to keep in mind:
- The name of an identifier that we want to declare as a constant has to be written in capital letters. For example, COST = 81500.
- We cannot change or alter the value of the constant if the name of the constant is followed by private access specifier.
- The value of the constant can be changed or altered in the program if the name of a constant is followed by the public access specifier.
Types of Constants
Now, let us understand six types of constants available in Java
- Numeric Constants
- Integer Constants
- Real Constants
- Non-numeric Constants
- Character Constants
- String Constants
Let us now discuss each of the above constants in depth.
Numeric Constants
It refers to the constants consisting of the numerals. A foremost sign and decimal points are allowed to be used.
Rule to Define Numeric Constants
- Must have at least one digit.
- It should not containanycommas, space, and other special symbol.
- It may have positive or negative signs. If no sign is preceded then the constant is assumed positive. It is not mandatory to precede a constant with a positive sign.
Integer Constants
It refers to those constants that contain digits (0-9). It does not include any decimal point and is called an integer constant. By default, its datatype is int.
There exist three types of integer constants in java:
- Decimal Constants: It consists of digits between 0 to 9. Any Numbers formed with the combination of these 10 digits are allowed here. Here number should not start with 0. For example, 7218, 97, 89.
- Octal Constants: The digits between 0 to 7 are included here and must begin with 0. For example, 0182, 091, 047.
- Hexadecimal Constants: It contains digits lying between 0 to 9 and letters from a to f (including upper caseas well as a lower case). It must begin with 0X or 0x. For example, 0x213, 0x89, 0X9a, 0XRR, Ox56sA.
Real Constants
It refers to those Numeric constants that have a decimal point and are called real or floating-point constants. the real constants are of double type automatically. We can indicate the type of a floating-point constant as a float by adding the letter f or F at the end of the constant. For example, 67f, 98F, 3f, 45f, -0.14f, 5.6F, etc.
The following two forms are used to write a real constant:
1. Fractional Form:
Protocols to define Fractional Form:
- It is compulsory to use at least one digit.
- It is mandatory to use a decimal point (.)
- It mightcontain positive or negative signs. The default sign is a positive (+) so, it is not mandatory to use that.
- It should not contain symbols such as - Commas, spaces, etc.
For example, 4.14, -18.1, 0.67, -9.6, 4.21, -6.82, 2.64.
2. Exponential Form
This form is used to represent a real constant when a number is too insignificant or huge.
For example, 0.00000328 can be represented as 3.28e-6. The portion of the number before e is called mantissa i.e 3.28, whereas, the portion after e is termed as the exponent i.e, 6.
Rules to Define Exponent Form
- The letter e or E is taken into account to separate Mantissa and exponent parts.
- Mantissa can either be positive or negative, the default is positive.
- Exponent must contain at least one digit.
- The exponent can also be positive or negative but positive is the default one.
For example: 800.34e4, -41E10, 0.96E10, -0.94e15, 5.32e45.
Non-numeric Constants
A constant without digits is termed a non-numeric constant. There are the following two types of non-numeric constants:
- Character Constants
When a single alphabet, digit, or any special symbol isenclosed withina single quotation mark, then they are termed character constant. For example, 'X', 'd', '6', 'e', '@', '&', '8', ‘3’.
The extreme length of a character constant is 1 character long. It means that it cannot contain more than one character inside single quotation marks.
Every character constant has its unique value called ASCII value associated with it and the value gets stored in ASCII format only. We already know everything gets stored in the binary format inside the computer memory. - String Constants
When any character is enclosed within a double quotation mark, they are referred to as a string constant. Even the double quote can have zero characters enclosed within. The null character i.e '\0' automatically gets placed by the compiler at the end of the string.
For example - "world", " " (it denotes blank space), "531" , “Computer”.
Backslash Character Constants
The backslash character constants are also supported in Java. These are also termed escape sequences. These are not printed on the screen, hence are called non-printable characters. These are used in output methods. It consists of 2 characters backslash and an alphabet but represents single character only.
A backslash symbol here is followed by an alphabet. For Example- \n, \f, \t, \a, etc.
A table containing a list of backslash characters
The following table represents the backslash character constants used in Java.
Backslash character | Description |
\n | Takes the cursor to a new line |
\f | From the feed |
\b | Used for backspacing |
\r | Returns carriage |
\t | Tab - Horizontal |
\v | Tab - Vertical |
\? | Denotes question mark |
\an | Denotes alert |
\’ | Single quotation |
\” | Double quotation |
\xN | Denotes a hexadecimal constant |
\N | Denotes octal constant |
\\ | Denotes backslash |
Let's use these constants in a Java program.
Implementation
Let us now see a program to understand the concept in a better way.
The following program whose class name is ConstantExample shall help us grasp more about the topic.\
public class ConstantExample
{
public static void main(String args[])
{
//declaration of byte constant
final byte x1 = 32;
final byte x2;
x2 = -15;
//declaration of short constant
final short x3 = 89;
final short x4;
x4 = -82;
//declaration of int constants
final int x5 = 190;
final int x6;
x6 = -952;
//declaration of long constant
final long x7 = 60000;
final long x8;
x8 = -21345;
//declaration of float constant
final float x9 = 16.72f;
final float x10;
x10 = -117.34f;
//declaration of double constant
final double x11 = 8000.1234;
final double x12;
x12 = -12715.111;
//declaration of boolean constant
final boolean x13 = false;
final boolean x14;
x14 = true;
//declaration of char constant
final char x15 = 'a';
final char x16;
x16 = 'p';
//declaration of a string constant
final String str="India is a developing country";
//octal constant representation
final int x=0232, y=016; //x=100 and y=20
int z=x-y;
//representation of hexadecimal constants
final int one = 0X321, two = 0xAFC;
//representing double constant in exponential form
final double exponent= 3.13E4;
//displaying the values of all variables
System.out.println("value of variable 1: "+x1);
System.out.println("value of variable 2 : "+x2);
System.out.println("value of variable 3: "+x3);
System.out.println("value of variable 4: "+x4);
System.out.println("value of variable 5 : "+x5);
System.out.println("value of variable 6 : "+x6);
System.out.println("value of variable 7 : "+x7);
System.out.println("value of variable 8 : "+x8);
System.out.println("value of variable 9: "+x9);
System.out.println("value of variable 10 : "+x10);
System.out.println("value of variable 11 : "+x11);
System.out.println("value of variable 12 : "+x12);
System.out.println("value of variable 13 : "+x13);
System.out.println("value of variable 14 : "+x14);
System.out.println("value of variable 15 : "+x15);
System.out.println("value of variable 16 : "+x16);
System.out.println(str);
System.out.println(z);
System.out.println("Hexadecimal: "+one+", "+two);
System.out.println(exponent);
}
}
Output:
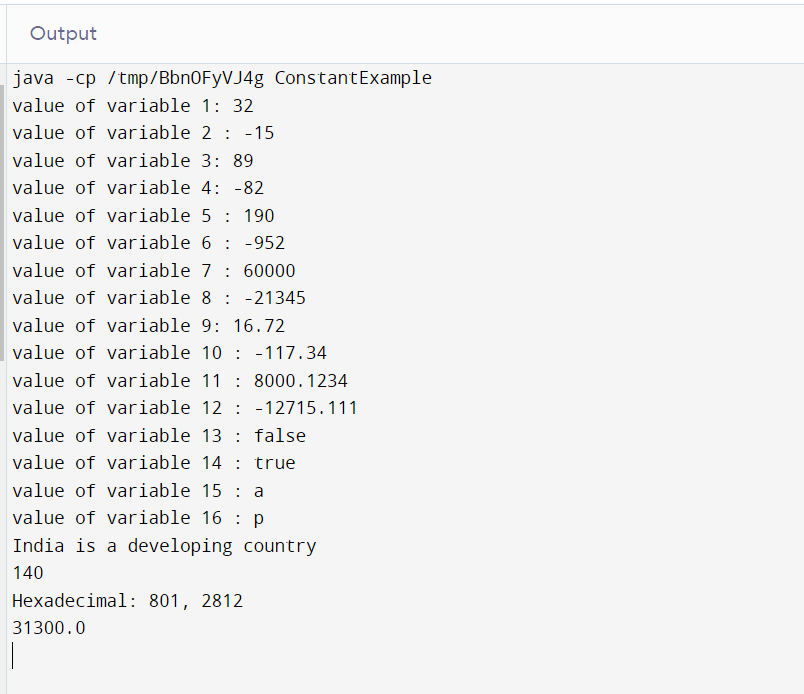
Explanation: This image shows the output of the above program. It shows the different types of constants in Java. The program shows 12 kinds of constants There are 18 variables to understand the concept well.
Summary
In this tutorial, we dealt with the various constants in java. We initiated our discussion with the basics of constants and moved forward towards the list of constants and understanding each one separately. We also saw a practical approach to comprehending the topic. That is all about the topic.