Highest precedence in Java
In Java, the operator is the first thing that springs to mind when discussing precedence. The order in which the operators in an expression are evaluated is controlled by a set of rules in Java. The idea of operator precedence is used to select the set of terms in an expression. The expressions are evaluated by the operator precedence. Parentheses() and Array subscript[] are given priority over all other operations in Java. For instance, the operators for addition and subtraction come before the left and right shift operators.
The table below is defined with the lowest precedence operator at the top.
Precedence | Operator | Type | Associativity |
1) | = + = - = * = / = % = | Assignment Addition assignment Subtraction assignment Multiplication assignment Division assignment Modulus assignment | Right to left |
2) | ? : | Ternary conditional | Right to left |
3) | || | Logical OR | Left to right |
4) | && | Logical AND | Left to right |
5) | | | Bitwise inclusive OR | Left to right |
6) | ^ | Bitwise exclusive OR | Left to right |
7) | & | Bitwise AND | Left to right |
8) | ! = = = | Relational is not equal to Relational is equal to | Left to right |
9) | < < = > > = instanceof | Relational less than Relational less than or equal Relational greater than Relational greater than or equal Type comparison (objects only) | Left to right |
10) | >> << >>> | Bitwise right shift with sign extension Bitwise left shift Bitwise right shift with zero extension | Left to right |
11) | - + | Subtraction Addition | Left to right |
12) | * / % | Multiplication Division Modulus | Left to right |
13) | - + ~ ! ( type) | Unary minus Unary plus Unary bitwise complement Unary logical negation Unary typecast | Right to left |
14) | ++ -- | Unary post-increment Unary post-decrement | Right to left |
15) | · () [] | Dot-operator Parentheses Array subscript | Left to Right |
Precedence order
The operator with the highest precedence takes priority when two operators share a single operand. In contrast to x * y + z, which is considered as (x * y) + z since the * operator has higher precedence than the + operator, x + y * z is treated as x + (y * z).
Associativity
The term "associative" refers to the operators used when two operators with the same precedence are present in an expression. To get out of that dilemma, the associativity notion is really beneficial. Consider the expression a + b - c. Since the + and - operators have equal priority, this expression will be interpreted as (a + (b - c)), since the + and - operators are right-to-left associative. The unary post-increment and decrement operators, on the other hand, are right to left associative, thus a++--b+c++ will be interpreted as ((a++)+(--b)+(c++))).
To clarify how an expression is assessed using precedence order and associativity, a definition is provided below.
Expression: x = 4 / 2 + 8 * 4 - ( 5+ 2 ) % 3
Solution:
- The highest precedence operator in the preceding expression is (). Therefore, the parenthesis starts the calculation process first.
x = 4 / 2 + 8 * 4 - 7 % 3 - Now that the /, *, and % operators have the same precedence and are higher than the + and -, we can solve them using the associativity idea. These operators have a left to right associative. Therefore, the / operator executes first, followed by * and% concurrently.
x = 2 + 8 * 4 - 7 % 3
x = 2 + 32 - 7 % 3
x = 2 + 32 - 1 - Now, + and - operators both also have the same precedence, and the associativity of these operators lest to the right. So, + operator will go first, and then - will go.
x = 34 - 1
x = 33
Program
Filename: HighestPrecedence.java
//import classes
import java.util.*;
//creating HighestPrecedence class to evaluate the expression
public class HighestPrecedence {
//main() method starts
public static void main(String[] args) {
//initialize variables with default values
int x = 2;
int y = 5;
int z = 12;
//calculating exp1, exp2, and exp3
int exp1 = x +(z/x+(z%y)*(z-x)^2);
int exp2 = z/x+y*x-(y+x)%z;
int exp3 = 4/2+8*4-(5+2)%3;
//printing the result
System.out.println(exp1);
System.out.println(exp2);
System.out.println(exp3);
}
}
Output
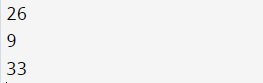