MVC in Java
A well-known design pattern is Model-View-Controller. The discipline of web development. We can organize our code in this manner. The document stipulates that a program or application must include a data model, display information, and control information. All these elements must be divided into separate objects to follow the MVC design.
Model-View-Controller Pattern is also known as the MVC pattern. This pattern is employed to divide the concerns of an application.
• Model: Model depicts a data-carrying object or Java POJO. Additionally, data changes may include logic to update the Controller.
• View: View represents how the model's data is visualized.
• Controller: The Controller affects both the View and the model. It refreshes the display and manages the data flow into the model object whenever data changes. View and model are kept apart.
The Model in Java programming comprises the basic Java classes, the Controller houses the servlets, and the View is used to show the data.
As a result of this division, the user requests are handled as follows:
1. The Controller on the server side receives a request for a page from the client.
2. The model is then called by the Controller. It compiles the needed information.
3. The data is sent from the Controller to the view layer.
4. The View is now returning the result to the client, which is the browser.
Benefits of the MVC Architecture
1. Scalability is a property of MVC that aids in expanding applications.
2. There is minimal dependence, making maintenance of the components simple.
3. Multiple views can reuse a model, allowing code reuse.
4. The developers can all work on the three layers—Model, View, and Controller—at once.
5. The program is made easier to comprehend by using MVC.
6. Each layer is maintained individually using MVC, so we don't need to deal with a tone of code.
7. It is simpler to test and extend applications.
Java implementation of MVC
We'll make an Employee object that will serve as a model. EmployeeView will be a view class that may publish Employee information on the console. EmployeeController is the controller class in charge of storing data in the Employee object and updating the View EmployeeView as necessary.
MVC Program in Java
EM.java
public class EM {
// Declaring the variables
private String employee_name;
private String employee_id;
private String employee_department;
// getId() method is used to get the id
public String getId() {
return employee_id;
}
// setId() method is used to set the id
public void setId(String id) {
this.employee_id = id;
}
// getName() method is used to get the name
public String getName() {
return employee_name;
}
// setName() method is used to set the name
public void setName(String name) {
this.employee_name = name;
}
// get department() method is used to get the Department
public String getDepartment() {
return employee_department;
}
/ setDepartment() method is used to set the Department
public void setDepartment(String Department) {
this.employee_department = Department;
}
}
EV.java
public class EV {
public void printEmployeeDetails (String EmployeeName, String EmployeeId, String EmployeeDepartment){
System.out.println("Employee Details: ");
System. out.println("Name: " + EmployeeName);
System.out.println("Employee ID: " + EmployeeId);
System.out.println("Employee Department: " + EmployeeDepartment);
}
}
EC.java
public class EC {
private Em model;
private EV v1;
public EC(Em model, EV v1) {
this.model = model;
this.v1 = v1;
}
public void setEmployee_name(String name){
model.setName(name);
}
public String getEmployee_name(){
return model.getName();
}
public void setEmployee_id(String id){
model.setId(id);
}
public String getEmployee_id(){
return model.getId();
}
public void setEmployee_department(String Department){
model.setDepartment(Department);
}
public String getEmployee_department(){
return model.getDepartment();
}
public void updateView() {
v1.printEmployeeDetails(model.getName(), model.getId(), model.getDepartment());
}
}
MVC1.java
public class MVC1{
public static void main(String[] args) {
// retrieving the employee record from the database using the employee id
Em m1 = retriveEmployeeFromDatabase();
// making a view to display employee information on the console
EV v1 = new EV();
EC c1 = new EC(m1, v1);
c1.updateView();
c1.setEmployee_name("kamal");
System.out.println("\n Details of employee after updation: ");
c1.updateView();
}
private static Em retriveEmployeeFromDatabase(){
Em E1 = new Em();
E1.setName("Rohith");
E1.setId("30");
E1.setDepartment("manager");
return E1;
}
}
Output

Program2 For MVC in Java
Student1.java
public class Student1 {
private String roll_no;
private String name;
public String getRollNo() {
return roll_no;
}
public void setRollNo(String roll_no) {
this.roll_no = roll_no;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
SV.java
public class SV {
public void printStudentDetails(String studentName, String studentRollNo){
System.out.println("Student: ");
System.out.println("Name: " + studentName);
System.out.println("Roll No: " + studentRollNo);
}
}
SC.java
public class SC {
private Student1 model;
private SV v1;
public SC(Student1 model, SV v1){
this.model = model;
this.v1 = v1;
}
public void setStudent_name(String name){
model.setName(name);
}
public String getStudent_name(){
return model.getName();
}
public void setStudent_rollNo(String rollNo){
model.setRollNo(rollNo);
}
public String getStudent_rollNo(){
return model.getRollNo();
}
public void updateView(){
v1.printStudentDetails(model.getName(), model.getRollNo());
}
}
MVC2.java
public class MVC2 {
public static void main(String[] args) {
Student1 m1 = retriveStudentFromDatabase();
SV v1 = new SV();
SC c1 = new SC(m1, v1);
c1.updateView();
c1.setStudent_name("Rohith");
c1.updateView();
}
private static Student1 retriveStudentFromDatabase(){
Student1 s1 = new Student1();
s1.setName("kamal");
s1.setRollNo("10");
return s1;
}
}
Output
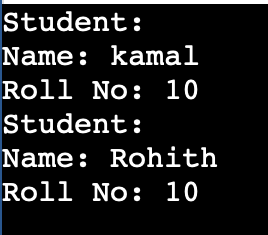