Shift right zero Fill Operator in Java and Operator Shifting
Left shift operator ( << ) :
The left shift operator is an operator which performs its action at the bits level of a binary Operator. That means when you perform a bitwise left Shift on any operator, and it shifts the position of that particular bit to its next higher bit representation in binary order.
It is denoted with as “ << ”.
If X<< 1 is applied on an operator, then the bits shifted only once to the left. If X << 2 is applied, then the bit gets shifted twice to the left side. Similarly , if X<< n is applied , then the bit gets shifted n times to its left.
For example ,
Consider 7 << 2 ( read as seven left Shift of 2 )
Shifting the value of X to the left two positions results in the loss of the leftmost two bits. X is equal to 7. The binary equivalent of 7 is 00000111. The following example demonstrates how to perform a left shift :
In the preceding example, the binary number 00000111 ( in decimal 7 ) becomes 00011100 after shifting the bits to the left ( in decimal 28 ). This is how the left shift operator works on numbers.
Bitwise right shift ( << ) :
The Right Shift Operator adjusts the bits of an integer to the right by a specified n place. The right shift operator is represented by the symbol “ >> ” , which stands for twofold larger than. The definition of x >> n is to shift the bits x to the right n specified places.
“ >> ” transfers the bits to the right while retaining the sign bit, which is the leftmost bit. The number's sign is represented by the leftmost bit. The sign bit 0 denotes a positive number, while the sign bit 1 denotes a negative number. So, when we perform “ >> ” on a positive value, we likewise get a positive value as a result. When we use “ >> ” on a negative sign, we receive another negative value.
If X >> 1 is applied on an operator, then the bits shift only once to the right. If X >> 2 is applied , then the bit gets shifted twice to the right side. Similarly , if X >> n is applied , then the bit gets shifted n times to its right.
For example ,
Consider 7 >> 2 ( read as 7 right shift of 2 )
Shifting the value of X to the right two positions results in the loss of the rightmost two bits. X is equal to 7. The binary equivalent of 7 is 00000111. The following example demonstrates how to perform a right shift:
In the preceding example, the binary number 00000111 ( in decimal 7 ) becomes 00000001 after shifting the bits to the left ( in decimal 1 ). This is how the right shift operator works on numbers.
Bitwise Zero Fill Right Shift Operator ( >>> ) :
The zero-fill right shift operator (>>>) shifts the binary representation of the left-hand operand by the number of bits given by the second operand, modulo 32. It examines the left-hand operand as just an unsigned integer.
Excess bits are thrown to the right, while zero bits are moved in from the left. The outcome is always positive because the sign bit is set to zero. Unlike the other bitwise operators, zero-fill right shift produces an unsigned 32-bit integer. The Bitwise Zero Fill Right Shift Operator moves an integer's bits n places to the right. There were zeros in the sign bit. The letter ">>" stands for the Bitwise Zero Fill Right Shift Operator.
When we use “ >>> ” on a positive number, we get the same result as “ >> ” . When we apply “ >>> ” to a negative number, it produces a positive result. MSB is substituted with a 0.
This Operator moves every bit by the specified number of bits to the right. What makes Right Shift & Zero Fill different from one another? Right Shift preserves the sign bit (i.e., a >> 2 will become a negative value if a = -8), whereas Zero fill does not. Right Shift doesn't (i.e., a >> 2 becomes a positive value if a = -8).
You might be wondering how a zero fill right shift operator works if the integer is negative. If N is the number of bits to be right shifted, excess bits are relocated N places to the right and discarded, while zero bits are transferred N places to the left and retained. Because the sign bit is set to zero, the outcome is always positive.
In the above example , the binary number 00100000 ( in decimal 32 ) becomes 00000100 once the bits are shifted to the right ( in decimal 4 ). The final three bits moved out and were lost.
Difference between right shift ( >> ) and zero fill right shift ( >>> ) :
“ >> ” and “ >>> ” are both used to shift the bits to the right . The distinction is that the operation >> preserves the sign bit , but the operator “ >>> ” does not. To keep the sign bit , you must add 0 to the MSB.
Main.java
public class Main
{
public static void main ( String [ ] args ) {
byte a, b ;
a = 7 ;
b = -7 ;
System.out.println ( " Left Shift: a<<2 = " + ( a << 2 ) ) ;
System.out.println ( " Right Shift: b>>2 = "+ ( b >> 2 ) ) ;
System.out.println ( " Zero Fill Right Shift: a >>> 2 = " + ( a >>> 2 ) ) ;
System.out.println ( " Zero Fill Right Shift: b >>> 2 = " + ( b >>> 2 ) ) ;
}
}
Output :
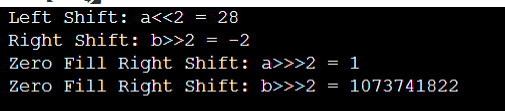