Java Subtract Days from Current Date
Dealing with date and time in Java is not a particularly challenging operation because Java has an API for date and time that simplifies duties for developers. There are two ways to subract the current date and any particular day from the number of days.
A duplicate of this LocalTime is returned by the LocalTime class's minus() function with the supplied amount deducted from the date-time.
The number of days to deduct from the current date is represented by a long value that the method takes.
The number of days to subtraction may be both positive and negative, so keep that in mind.
After removing the days, it gives back a duplicate of the date.
If the outcome exceeds the supported date range, it throws a DateTimeException.
- Java Calendar Class Utilization
- Using the LocalDate Class in Java
Java Calendar Class Utilization
We have incorporated the Java Calendar class into the following Java application. The getTime() function is used to output the current date and time on the console after first creating an instance of the Calendar class in order to obtain the current date. By using Calendar.DATE and the add() function, you may subtract the days.
Java program to subtract days from current date using Java Calender Class utilization
CalenderClass.java
import java . util . Calendar ;
public class CalenderClass
{
public static void main ( String s [ ] )
{
Calendar c = Calendar . getInstance ( ) ;
System . out . println ( " Current Date = " + c . getTime ( ) ) ;
c . add ( Calendar . DATE , -2 ) ;
System . out . println ( " Modified date = " + c . getTime ( ) ) ;
}
}
Output

To obtain the current date, we may alternatively utilise the DAY OF YEAR field rather than the DATE field.
Java program to subtract days from current date using Java Calender Class
CalenderClass2.java
public class CalenderClass2
{
public static void main ( String s [ ] )
{
Calendar c = Calendar . getInstance ( ) ;
Date today = c . getTime ( ) ;
System . out . println ( " Present Date : " + today ) ;
c . add (Calendar.DAY_OF_YEAR , -150 ) ;
Date d = c . getTime ( ) ;
System . out . println ( " before 150 days date is : " + d ) ;
}
}
Output

Using Java LocalDate Class
For managing date and time, Java 8 adds a new DateTime API.
The API offers numerous Java classes.
However, the LocalDate class, which stores a date value, is one of the most helpful classes in this API. The Java.time package contains it.
We have utilised the parse() function of the LocalDate class in the following Java programme.
SubtractDays1.java
import java .time . LocalDate ;
public class SubtractDays1
{
public static void main ( String args [ ] )
{
LocalDate date1 = LocalDate . now ( ) ;
System . out . println ( " Present Date " + date1 ) ;
LocalDate newD = date1 . minusDays ( 46 ) ;
System . out . println ( " Modified Date: "+ newD ) ;
}
}
Output:
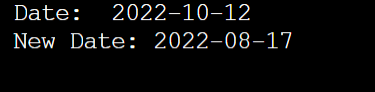
Program to subtract days from the specified date
SubtractDays2.java
import java .time . * ;
public class SubtractDays2
{
public static void main ( String s [ ] )
{
LocalDate da = LocalDate . parse ( "2022-02-11" ) ;
System . out . println (" Date : "+ da ) ;
LocalDate newD = da . minusDays (15);
System . out . println ( " New Date: "+newD ) ; }
}
Output:
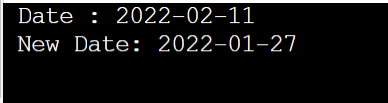
We can also include the time zone in the above program.
SubtractDaysZone.java
public class SubtractDaysZone
{
public static void main (String s [ ] )
{
LocalDate d = LocalDate . now ( ZoneId . of("Europe/Paris")) . minusDays ( 30 ) ;
System . out . println ( " New Date: "+ d ) ;
}
}
Output
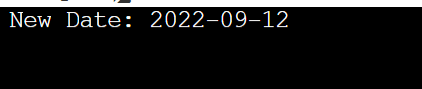
Java program to subtract days from the given time
SubtractDaysTime . java
public class SubtractDaysTime
{
public static void main ( String s [ ] )
{
LocalDate d = LocalDate . parse ( " 2018-11-14 " ) ;
System . out . println ( " LocalDate "+ " before subtracting days: " + d ) ;
LocalDate value = d . minusDays ( -15 ) ;
System . out . println ( " LocalDate after "+ " subtracting days : " + value );
}
}
Output
