Java Future Example
Future is an interface in the Java language that is a part of java.util.concurrent package. It serves as a symbol for the output of an asynchronous computation. The interface offers ways to determine whether a computation has finished, wait for it to finish, and receive its result. Once the task or calculation is finished, it cannot be undone. A Future interface offers ways to determine whether the computation is finished, to wait for it to finish, and to receive the computation's results. When the computation is finished, the result is retrieved using the Future's get() method, and the computation stalls until it is finished. Future and FutureTask are both included in the Java 1.5 java.util.concurrent package.
Future Inteface() Methods
Method | Description |
cancel() | It tries to halt the task's execution |
get() | Receives the result after, waiting for the computation to finish. |
isCancelled() | If the task was stopped before it finished, the isCancelled() function returns true. |
isDone() | The isDone() function returns true if the work has been finished. |
Getting Result
Future is what an asynchronous task produces. The Java Future interface provides the following two iterations of the get() method, both of which return an object to receive the outcome of that asynchronous task.
Syntax
Object r = f.get();
Cancel Asynchronous task
Using the cancel() function of the Future interface, we can end an asynchronous job at any time.
Syntax
Future future = ret;
future.cancel();
Verify if an asynchronous task has been completed
The interface offers the isDone() method to determine whether or not the asynchronous task has been finished.
Syntax
Future future = ...
if(future.isDone())
{
Object result = future.get();
}
Check if an Asynchronous Task is Cancelled
The isCancelled() method of the Future interface can be used to determine whether or not the asynchronous job that Future represents has been cancelled. If the task is successfully canceled, it returns true; otherwise, it returns false.
Syntax
Future future = ...
if(future.isCancelled())
{
}
- FutureTask implements the Future interface and the RunnableFuture interface, allowing it to be used as a runnable and sent to the ExecutorService for execution.
- ExecutorService typically creates FutureTasks when Callable or Runnable objects are called with Future. submit(), but it is also possible to manually construct FutureTasks.
- FutureTask functions as a latch.
- FutureTask's computation model is built using the Callable interface.
- The Future or Callable interface is implemented.
- The task's state affects how the get() method behaves. If tasks are not finished, the get() method waits or blocks until they are. When a job is finished, it returns the outcome or raises an ExecutionException.
Java program for Future Example
FutureExample.java
// import required packages
import java . util . concurrent . * ;
import java . util . logging . Level ;
import java . util . logging . Logger ;
class R1 implements Runnable {
//
private final long wt ;
public R1 ( int time )
{
this.wt= time ;
}
@Override
public void run ( )
{
try {
Thread . sleep ( wt ) ;
System . out . println ( Thread . currentThread() . getName () ) ;
}
catch ( InterruptedException ex) {
Logger . getLogger ( R1 . class . getName () ) . log ( Level . SEVERE ,null , ex ) ;
}
}
}
class FutureExample {
public static void main ( String[] args )
{
R1 runnable1 = new R1 ( 1000 ) ;
R1 runnable2 = new R1 ( 2000 ) ;
FutureTask <String> ft1 = new FutureTask <> ( runnable1 ," FutureTask1 is complete " ) ;
FutureTask <String> ft2 = new FutureTask <> ( runnable2 ,"FutureTask2 is complete " ) ;
ExecutorService exe = Executors . newFixedThreadPool ( 2 ) ;
exe . submit ( ft1 ) ;
exe . submit ( ft2 ) ;
while (true) {
try {
if (ft1.isDone() && ft2.isDone()) {
System.out.println("Both FutureTask Complete");
exe.shutdown();
return;
}
if (!ft1.isDone()) {
System.out.println(" output of futureTask1 = "+ ft1.get());
}
System.out.println( "FutureTask2 to complete Waiting " ) ;
String str = ft2 . get ( 250 , TimeUnit . MILLISECONDS ) ;
if (str != null) {
System . out . println ( " Output of FutureTask2 = " + str ) ;
}
}
catch ( Exception ex ) {
System . out . println ( " Exception: " + ex ) ;
}
}
}
}
Output:
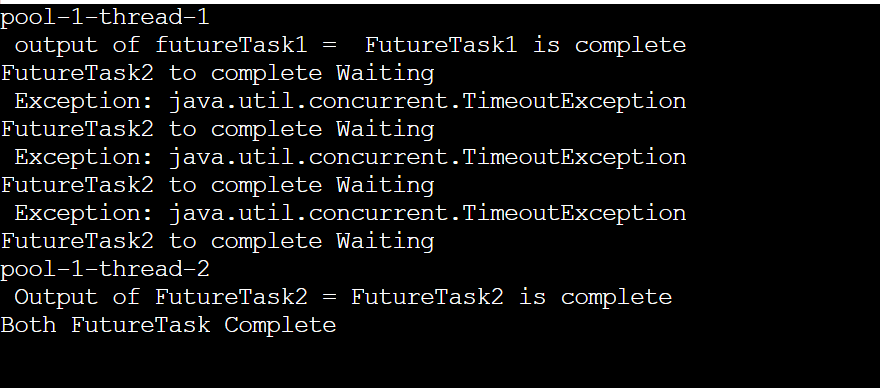