&& operator in Java
“ && ” is the conditional - And operator in Java. In Java, it is an example of a logical operator. In Java, the “ & ” operator has two purposes. In one case, it is referred to as a logical - And operator. In the opposite case, it is known as the bitwise - AND operator. These operators are all binary operators. This indicates that each operand has an operand on both its left and right. All of the expression's output can be allocated to a variable. Because these operations deal with primitive types, the programmer does not need to import its class.
The " AND ” operator in Java works based on the correctness of the expressions. That is, it only works when the operators with “ AND ” composition return a true value.
This helps in figuring out the standard conditions required to be followed for the evaluation of a problem.
You can understand the working of “ && ” operator with its truth table.
Exp1 value | Exp2 value | AND value |
True | True | True |
True | False | False |
False | True | False |
False | False | False |
By using AND, the evaluation is done by comparing the two Boolean expressions specified before and after “ && ”. The result will be true only if the return value of both expressions is true. Else false will be returned.
Let us see this with an example,
if (a == b && b > c)
{
//logic to be executed
}
Consider the above condition where you are checking for the relation between three variables “ a ” , “ b ” , “ c ”. The “ && ” operator will execute both expressions if both are true. Else it will come out of the statement.
Consider if the first expression itself is wrong, then what is the use of evaluating the second expression? This is what the “ && ” operator does. If the first operator itself is wrong, then it will not check for the second expression.
Difference between Bitwise AND ( & ) and Logical AND ( && ) :
The Bitwise AND operator refer to the bitwise AND operator ( & ). It is based on a single bit. It requires two operands. A bit in the result is one if and only if both operand's corresponding bits are 1. The operator's output can be any number.
The logical AND operators are the logical AND operators ( && ). It is mostly found in loops and conditional expressions. It is commonly found in Boolean expressions. “ && ” always produces a 0 or a 1.
Bitwise AND ( & ) operator evaluate both expressions on which it is being applied. It is helpful in operating with Boolean datatypes and as well as bits. It is used to examine the logical state and to block off specific bits like parity bits.
The logical AND ( && ) operator evaluates only the left given expression and is helpful in operating with the only Boolean datatype. It is used to examine only the logical conditions of the expressions.
AndDemo.java:
public class AndDemo
{
public static void main ( String args [ ] )
{
int abb = 10 , baa = 20 ;
if ( abb == 10 && baa == 20 )
{
System.out.println(" true condition given ") ;
}
else
{
System.out.println (" false condition given ") ;
}
int cdd = 78, dcc = 90 ;
if ( abb > cdd && baa < dcc )
{
System.out.println (" true condition is given ") ;
}
else
{
System.out.println ( " false condition given ") ;
}
}
}
Output :
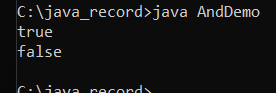
BitwiseAndDemo.java :
import java.io.*;
import java.util.*;
public class BitwiseAndDemo
{
public static void main ( String args [ ])
{
Scanner sc=new Scanner ( System.in ) ;
System.out.println ( " Enter the value of a : " ) ;
int a = sc.nextInt( ) ;
System.out.println ( " Enter the value of b : " ) ;
int b = sc.nextInt( ) ;
Solution obj=new Solution( ) ;
obj.bitAnd ( a , b) ;
}
}
class Solution
{
void bitAnd ( int a , int b )
{
System.out.println ( " bitwise and of the given inputs are : " ) ;
System.out.println ( " a & b = " + ( a & b ) ) ;
}
}
Output:
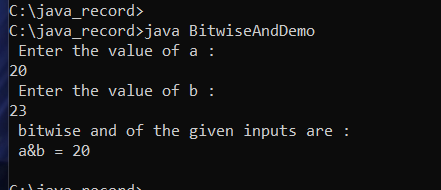