How to sort a String in Java
Sorting is the process of putting the elements in a certain order, either ascending or descending. Mostly the alphabetical order or natural order is used for a string. In other words, sorting is the process of converting data in standard form (to make it human-readable).
Java String
In Java, a string is considered as an object which stores the sequence of character values. A character array works similar to a Java string.
Java strings are immutable in nature, i.e., we cannot modify the value of string object once it is created. Therefore, when we sort a string, the characters are shuffled after sorting, and we have to create a new string to store the sorted String.
In Java, the String class does not have any method to sort a string directly; however, there are different methods and classes to sort a string.
Sorting a String in Java
Following are the ways sort a string in Java:
- Using Arrays.sort() method
- Using String.chars() method (Java 8)
- Manual sort with toCharArray() method
- Using Comparator class
Using Arrays.sort() method
The sort() method of the Arrays class sorts the characters on the basis of ASCII value, where we can define the custom Comparator to sort a string.
The steps to use Arrays.sort() method are:
- Convert the input string into a character array using the toCharArray() method.
- Once we get the character array, sort it using the Arrays.sort() method.
- Again, convert the sorted character array back to String. Here, we pass this array to the constructor of the String class.
Example:
Let's consider the below example where we sort the specified String in Java.
SortMethod.java
//importing Arrays class
import java.util.Arrays;
public class SortMethod
{
public static void main(String[] args)
{
//declaring and initializing a string
String str = "javatpoint";
//converting the string into character array using toCharArray() method
char[] ch = str.toCharArray();
//using sort() method to sort the array
Arrays.sort(ch);
//converting the sorted array back to String
//storing it in new string
String strNew = new String(ch);
//printing the before sorting
System.out.println("Input string: " + str);
//printing the after sorting
System.out.println("Output string: " + strNew);
}
}
Output:

Using String.chars() method (Java 8)
Java 8 Stream class provides the feature of sorting a string. It has a String.chars() method, which returns IntStream, which depicts the integer representation of the character of String. Once we get the IntStream, we can sort it and store the integers in sorted order in a StringBuilder object.
Example 1:
Consider the following example to sort the String using the String.chars() method in Java.
CharsMethod.java
public class CharsMethod
{
public static void main(String[ ] args)
{
//declaring and initializing a string
String str = "javatpoint";
//converting the string into IntStream using chars()
String strNew = str.chars()
.sorted()
.collect(
StringBuilder :: new,
StringBuilder :: appendCodePoint,
StringBuilder :: append)
.toString();
//printing the before sorting
System.out.println("Input string: " + str);
//printing the after sorting
System.out.println("Output string: " + strNew);
}
}
Output:

Example 2:
Rather than creating IntStream, we can convert each character in the String to a single character string and get a stream of strings.
Here, we need to import the stream.Collectors class and stream.Stream class of java.util package.
Let's consider the below example to understand.
StringSortUsingCollectors.java
//importing necessary classes
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class StringSortUsingCollectors
{
public static void main(String[ ] args)
{
//declaring and initializing a string
String str = "helloworld";
//converting the string into IntStream using chars()
String strNew = Stream.of (str.split(""))
.sorted()
.collect(Collectors.joining());
//printing the before sorting
System.out.println("Input string: " + str);
//printing the after sorting
System.out.println("Output string: " + strNew);
}
}
Output:

Manual sort using for() loop
We can also sort a Java string manually using the for loop and compare the string elements to shuffle and place them into ascending or descending order.
Here, we use two for loops to compare each element with another.
Following are the steps to sort a string manually in Java
- Convert the input string into a character array using the toCharArray() method.
- Sort the array using any array sorting technique. Here we are using bubble sort.
- Compare the first two elements of an array.
- If the first element is greater than the second, swap (switch their positions).
- Similarly, compare the second and third element and swap if the second element is greater than the third.
- Repeat the process until the end of the array.
Example:
Let’s consider the following example where we are sorting the string using manual sorting method.
ManualSortOfString.java
//importing necessary classes
import java.util.Arrays;
import java.util.Scanner;
public class ManualSortOfString {
public static void main(String args[ ]) {
int temp, size;
Scanner sc = new Scanner(System.in);
//accepting String from the user
System.out.println("Enter a string value: ");
String str = sc.nextLine();
//converting the string into character array
char charArray[] = str.toCharArray();
//finding the length of above array
size = charArray.length;
//traversing through the array to sort the elements
for(int i = 0; i < size; i++ ){
for(int j = i + 1; j < size; j++){
if (charArray[i] > charArray[j]){
temp = charArray[i];
charArray[i] = charArray[j];
charArray[j] = (char) temp;
}
}
}
//converting the character array back to string
String strNew = new String(charArray);
//printing the before sorting
System.out.println("Input string: " + str);
//printing the after sorting
System.out.println("Output string: " + strNew);
}
}
Output 1:

Output 2:
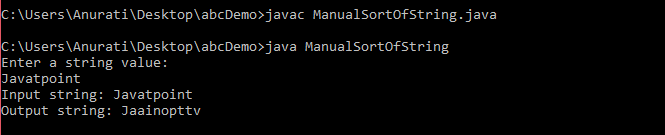
As we can see, the first output of the above example sorts all the lower case characters. However, the second output does not sort the String character in the upper case as it is a mixed string.
Sorting a mixed string (having uppercase and lowercase characters)
In order to sort a mixed string, i.e., a string with both uppercase and lower case characters, we can use the Comparator class of Java.
Following are the steps to sort a mixed string:
- Convert input string into a character array. Here we will use the for loop to add elements to the array.
- We will sort the character array using the Arrays.sort( T [ ], Comparator c) method. To use this, we have to implement the compare() method based on the custom sorting behavior.
- Now we will use the StringBuilder class to convert the sorted character array back to the String.
Example:
Let's consider the below example to understand how to sort a mixed string in Java using for loop.
MixedStringSort.java
//importing necessary classes
import java.util.Arrays;
import java.util.Comparator;
import java.util.Scanner;
public class MixedStringSort {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
//accepting string from the user
System.out.println("Enter a string value: ");
String str = sc.nextLine();
//finding the length of input string
int len = str.length();
//converting the string into character array
Character charArray[] = new Character[len];
for(int i = 0; i < len; i++){
charArray[i] = str.charAt(i);
}
//sorting the string
//ignoring case during sorting
Arrays.sort(charArray, new Comparator<Character>(){
@Override
public int compare(Character char1, Character char2)
{
// ignoring case
return Character.compare(Character.toLowerCase(char1),
Character.toLowerCase(char2));
}
});
//convert character array to String using StringBuilder class
StringBuilder sb_obj = new StringBuilder(charArray.length);
for (Character c : charArray){
sb_obj.append( c.charArray() );
}
return sb_obj.toString();
}
}
Output:
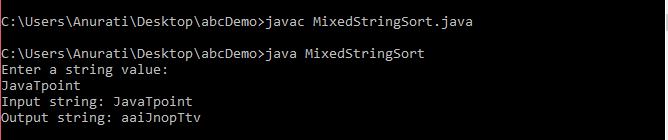
In the above output, all the characters of the string 'JavaTpoint' are sorted irrespective of the case.
In this way, we have learned how to sort a string in Java using different methods and classes.