Java Read File Line by line
Java provides two options to read files line by line. Each option offers different benefits and has its own set of drawbacks. Following are the ways through which on accomplish the above goal:
- bufferedReader
- scanner class
BufferedReader
This class comes under the java.io package, which is implemented when there is a need to read text from a character-input stream. It buffers characters to provide an efficient reading of the input, such as characters, arrays, and lines.
The buffer size is determined by the user or an already defined size which is, by default, that can also be used as it is large enough to serve maximum cases. It uses the concepts of inheritance to inherit the reader class. It implements the following interfaces:
- Closable
- AutoClosable
- Readable
Following is an example that shows how BufferReader class is declared (BufferedReader Syntax)
Public class BufferedReader extends Reader (Where public class is an access specifier)
BufferReader Constructors
BufferReader has two types of constructors, which are as follows:
1. BufferReader( Reader in)
This will use the default size of Bufferedreader.
2. BufferReader(Reader in, int n)
This will use the size specified by the user where “n” represents the size of the constructors. It throws IllegalArgumentException if the size of the array is less than and equal to zero.
BufferReader Methods
Following are the methods of BufferReader.
- Void Close(): Other methods, such as read() and mark(), will throw an IOException if this method is used.
- Void mark(): It marks the current position.
- Boolean markSupported(): As the name suggests, the above method checks or tells that the stream supports marl() operation. It overrides the mark supported in class Reader.
- Int read( ): It is used when only 1 character is to be read. It overrides read in class Reader.
- Int read( char[] buff, int off, int l): It is implemented when there is a need to read a portion of characters from the array.
- Debuff – destination buffer.
- Off – it is the point from where sorting characters get initiated.
- L – the number of characters you can read.
- String readLine(): In this case, a whole line of text is to be read.
- Boolean ready(): As the name suggests, the above method checks and tells whether the input is ready to be read. It overrides the ready class Reader.
- Void reset(): As the name suggests, the stream is reset to its most recent mark.
- Long skip( long n): It is implemented when there is a need to skip characters. In the above syntax, n represents the number of characters to be skipped. It overrides skip in class Reader.
Example
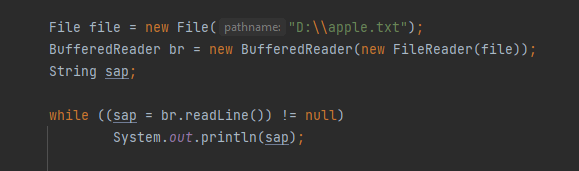
The above snippet of code is an example of how one can use BufferedReader to read contents from a file and print it.
In the first line, we have specified the path of the file. The file contains some text. We check whether the file is empty or not and then print the file's content. We can see files getting printed in the console as we run the program.
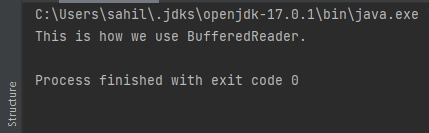
When to use BufferedReader to read files
- When we need a synchronous concept.
- When we are working with multiple threads.
- When we need to write millions of lines.
Scanner
In java, there is another way of taking input which is known as a Scanner. Java.Util.Scanner class can parse primitive types and strings. It implements the following functions:
Closable
Autoclavable
Iterator< string>
How Scanner Class Works
It takes the entire line and divides it into small elements. For example, the following is an input string.
MY name is Sahil
The scanner object will read the whole line and divide the above line into four elements or tokens, which are: " My ", " name ", " is " and " Sahil ”. Then object iterates all the above token and read each element depending upon the method type declared in the program.
Constructors
Following are the constructors provided by the Scanner class in java.
- Scanner (File Source)
This function is used to read data from a File object representing a file.
- Scanner ( InputStream source)
To read data from an InputStream, call this method.
- Scanner (Path source)
This method reads the file that the object specifies.
Example
Following a small piece of code is an example of the Scanner class's implementation.
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class a {
public static void main(String[] args) {
File file = new File("d://sample.txt");
try {
Scanner st = new Scanner(file);
while (st.hasNextLine()) {
String output = st.nextLine();
System.out.println(output);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
- First, we imported the necessary packages to implement the scanner class.
- Then we created an instance of file for the sample.txt file.
- After that, we created a Scanner object which will read the data which is inside the specified file ( Sample.txt )
- We have used the hasNextLine() method to read text line by line.
- Then the file’s content is being read and stored in the output string, and we printed the output (the reference variable of string) to see the results in the console, shown below.
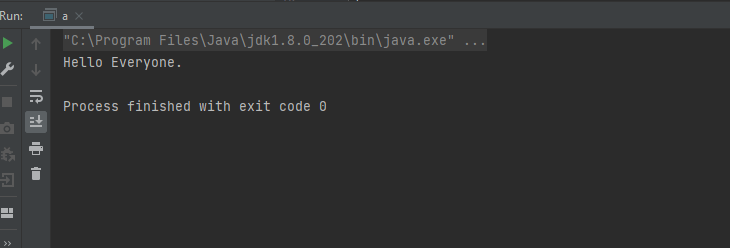
In the above code, we used a method named hasNextLine().
It is a Scanner class method with a boolean return type. It is implemented when there is a need to check wheater there is another line in the input. It was used in the while loop. If it returned true, the while loop has run again to read the content inside the file. But the file specified by us had only one line of text – Hello Everyone. Thus after running once, the hasNextLine() method returns false and makes the while loop terminate.
Comparison in Scanner class features with Buffered Reader class.
BufferedReader | Scanner |
It has a larger buffer memory. | It has a smaller buffer memory. |
It is synchronous. | It is not synchronous. |
It is comparatively faster. | It is comparatively slower. |
It should be used when we are working with multiple threads. | It should be used when we are not working with multiple threads. |