Java Program to remove duplicate characters in a string
In strings, we can find many characters present one or more times in a string; accessing a particular character is difficult due to repetition. Duplicate characters will present in the string can be removed in many ways. Some of the ways include:
- Using for loop
- By sorting method
- Hashing
- IndexOf()
Hence, many ways exist to remove duplicate characters from the given string.
These different methods have varying time complexities. We can use them based on request.
Using for loop
It is one of the easiest and simple ways to remove duplicate characters from the given string.
Steps for removing the duplicate keys using for loop.
- The given array is converted into a character array as the division of the Characters.
- Find the length of the array.
- Create a function for calling the duplicate elements in the array by passing the character as a parameter.
- Using for loop, visit all characters of the array to search the duplicate element.
- Check the character present in the string.
Example
import java.io.*;
import java.util.*;
class RemoveDuplicates
{
//creating method for removing duplicate characters
static void remove duplicate(char strings[], int len)
{
// declaring value for sttoring index
int n=0;
for(int i=0;i<len;i++)
{
// checking whether the character is present
int j;
for(j=0;j<i;j++)
{
if(strings[i]==strings[j])
{
break;
}
}
//if it was not present then add it result
if(j==i)
{
strings[n++]=strings[i];
}
}
System.out.println(String.valueOf(Arrays.copyOf(strings,n)));
}
//main section
public static void main(String s[])
{
String info=“java is a good programming language”;
//Converting to character array
char strings[]=info.toCharArray();
//finding length of character array
int lengt=strings.length;
// calling method for removal of characters
removeDuplicate(strings,lengt);
}
}
Output

Using Sorting method
The sorting method is one of the methods for removing duplicate characters in the string.
This method is faster as compared to the for-loop method. In this, first, the array is sorted.
And the duplicated elements are present adjacent to each other. Performing this operation includes:
- Sort the given string
- Take the current element and compare it with the remaining elements in the loop.
- The characters present for more than once can be removed.
- The technique does not maintain the authentic order of the input string. String order is different from initial.
A simple java Program using sorting techniques.
Example
import java.util.Arrays;
import java.util.*;
public class Main
{
static void removeDuplicates(String str)
{
//to find next character of string given
int ind1 = 1;
// to find next character in the resultant string
int ind2 = 1;
// converting string to indivial(Chaaracter array)
char arr[] = str.toCharArray();
//Checking whether the character is present in it or not
while (ind1 != arr.length)
{
if(arr[ind1] != arr[ind1-1])
{
arr[ind2] = arr[ind1];
ind2++;
}
ind1++;
}
str = new String(arr);
System.out.println(str.substring(0, ind2));
}
//method for storing the sorted string
static String sortString(String str)
{
// String is converted to character
char temp[] = str.toCharArray();
//sort array using array's sort () method
Arrays.sort(temp);
//String from character array
str = new String(temp);
// result
return str;
}
public static void main(String[] args)
{
//Declaring a default string
String str = “Java is best programming language";
//calling method for storing the sorted string
String newString = sortString(str);
System.out.println(newString);
// removeDuplicates() method for removal of same characters
removeDuplicates(newString);
}
}
Output
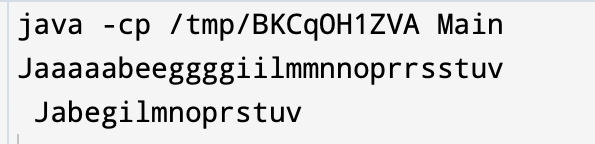
Using Hashing method
Another way to get rid of duplicate characters from a string is hashing. By using hashing, we can do that. We will use the subsequent steps to take away duplicates by using hashing:
- Inside the main() approach, we can create a string from which we ought to eliminate replica characters.
- We can call the removeDuplicates() approach and surpass the string from which we need to eliminate duplicates.
- Inside the removeDuplicates() technique, create a LinkedHashSet of character kind. LinkedHashSet includes a distinct detail. This set contains distinct elements. So that duplicate characters are removed. Ultimately, we print all of the characters of the LinkedHashSet.
- It includes characters in insertion order.
- This can be done using a simple program.
Example
import java.util.*;
//Class for accessing methods for removing duplicate characters
class Main
{
//Create a method removeDuplicates() for finding unique characters using Hashing
static void removeDuplicates(String str)
{
//HashSet class creation
LinkedHashSet<Character> set = new LinkedHashSet<>();
//each character is added to set
for(int i=0;i<str.length();i++)
set.add(str.charAt(i));
// string after removing same characters
for(Character ch : set)
System.out.print(ch);
}
//main()
public static void main(String args[])
{
//Default value is assigned to str
String str = "java is good programming language";
//calling method by passing string as a parameter
removeDuplicates(str);
}
}
Output:
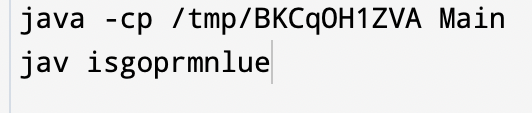
index() method
This method is used for the removal of duplicate characters from a string.
It uses index values to find distinct characters from the given string.
The steps followed in this are:
- The main() method contains a string for finding unique characters.
- The removeDuplicates() mehod is called, and the string is passed as an argument.
- The initial string length is calculated in the removeDuplicates() method.
- All characters are checked in the string.
- This method returns -1 if the element can’t be present in the string. So it was added to the resulting string.
Example
// import classes and packages
import java.util.*;
//create RemoveDuplicatesIndex class to remove characters
class RemoveDuplicatesIndex {
// Create removeDuplicates() for removing duplicate characters by index()
public static void removeDuplicates(String str)
{
//Empty string for storing
String newstr = new String();
//length is calculated using length() function
int length = str.length();
//visiting each and every character of string
for (int i = 0; i < length; i++)
{
// Index value of ith position is stored
char charAtPosition = str.charAt(i);
// check the charAtPosition index. if it returns true add it to result
if (newstr.indexOf(charAtPosition) < 0)
{
newstr += charAtPosition;
}
}
//Unique character string
System.out.println(newstr);
}
// main()
public static void main(String[] args)
{
// default value
String str = "Java is a object oriented programming language";
//calling method removeDuplicates method
removeDuplicates(str);
}
}
Output
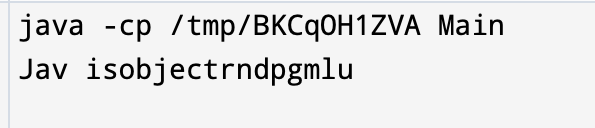
The above four methods can be used to remove duplicate characters. Any of the four methods can be used according to our choice.