Static Array in Java
In this tutorial, we will study static arrays in Java. An array is a data structure that is of great importance in any programming language. It is classified into two types – static arrays and dynamic arrays. In this tutorial, we will restrict ourselves to a static array only.
We will begin our discussion with an understanding of the topic, its examples, the syntax for its declaration, and its advantages and disadvantage. Further, we will sum up our discussion with a java program.
Static Array
A static array is declared with the static keyword. Its nature is hinted at in the name itself. We cannot change or alter the static array. The memory is allocated at compile-time and its size also gets fixed therein.
Limitations in altering the size of static arrays led to the rise of the dynamic array where one can easily take the size as input at run time.
Example
Let’s consider an array named arr[20] which creates an array of size 20.
It means we can insertelements up to 20 only; we cannot insert a 21st element as the size of the Array is fixed.
int arr[] = { 81, 83, 84 , 88, 89 }; // static integer array
int arr[] = { 32, 67, 44, 77 }; // static integer array
int arr[] = { 542, 167, 414, 707, 55 }; // static integer array
int* arr = new int[5]; // dynamic integer array
Declaration of a Static Array
Let us look at the way to declare a static array in java.
<data type> <name of variables> []={<data1>,<data2>,<data3>, .....<dataN>};
Here,
Data types – the type of data of the array
Name of variables – it consists of a list of variable names to be used.
{data1, data2, …..} – it consists of the list of data.
Examples
Let us look at the following examples to understand the ways to initialize the static array in java.
Example 1: By the aid of a new keyword
String[] states = new String[] {
"Arunachal Pradesh",
"Madhya Pradesh",
"Uttar Pradesh",
"Himachal Pradesh"
};
Example 2:
The array can also be declared in this way
String[] states = {
"Arunachal Pradesh",
"Madhya Pradesh",
"Uttar Pradesh",
"Himachal Pradesh"
};
Example 3:
This is another way to declare a static array. Here, it is being declared as a list.
List states = Arrays.asList(
"Arunachal Pradesh",
"Madhya Pradesh",
"Uttar Pradesh",
"Himachal Pradesh"
);
Advantages of Static Array
- Its execution time is efficient.
- Statically allocated arrays remain alive throughout the complete runtime of the program.
- Global variables are declared “in advance of time,” such as a fixed array.
- The size of memory allocated to “data” is static. But it is possible to alter the content of a static structure without growing the memory space allocated to it.
Disadvantages of Static Array
- It often leads to the wastage of space if a great number of static data has been allocated in the beginning.
- It is impossible to increase the space once after allocating the memory initially. So, if one fails to allocate an adequate amount of space, it becomes problematic later on.
Implementation
Let us see a program to understand the topic clearly.
Example 1
public class StaticArrayExample1
{
private static String[] arr;
static
{
arr = new String[5];
arr[0] = "India";
arr[1] = "is";
arr[2] = "a";
arr[3] = "developing";
arr[4] = "country";
}
public static void main(String args[])
{
for(int i = 0; i<arr.length; i++)
{
System.out.print(arr[i] + " ");
}
}
}
Output
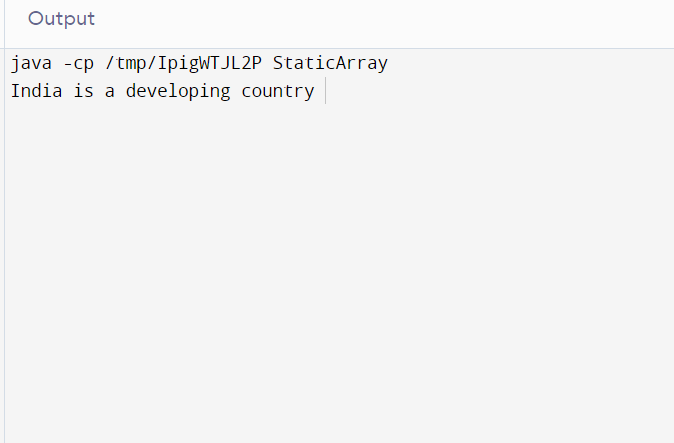
Description: This image shows the output of the code written above. Here, the keyword new has been taken into account to create a static array of type strings.
Example 2
public class StaticArrayExample2
{
// A static array of integer type will be created
static Integer[] intArray;
static
{
intArray = new Integer[] { new Integer(13), new Integer(42), new Integer(83), new Integer(49), new Integer(89)};
}
public static void main(String args[])
{
//for loop to iterate over static array
for (int j = 0; j <intArray.length; j++)
{
//to print array elements
System.out.println(intArray[j]);
}
}
}
Output
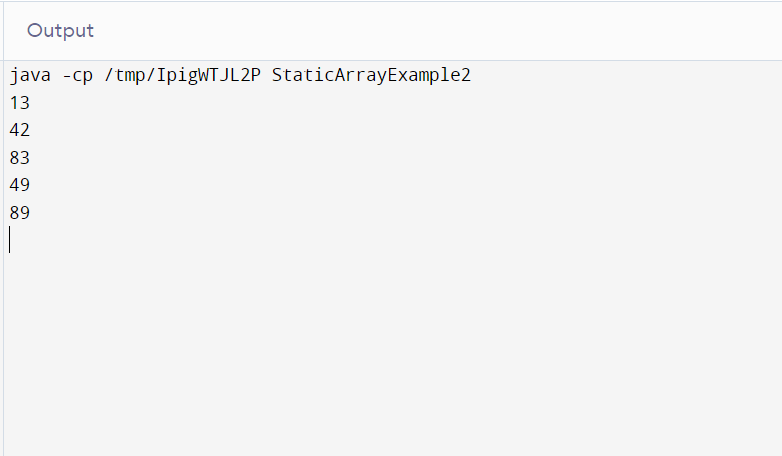
Description: This image shows the output of the code written above. Here, a static array of type integershas been created.
Summary:
In this tutorial, we understood what is meant by a static array with the help of certain examples. We saw the implementation to understand the topic even better.