How to achieve Multiple Inheritance in Java
Multiple Inheritance is the type of inheritance where one class inherits the properties of more than one super class. For example, class Child inherits (extends) the classes Father and Mother. If there are methods with the same name and parameters in both the super classes and the sub class in Java.
And we call the method, the compiler is unable to determine which class method to invoke. Thus, Java does not support Multiple Inheritance.
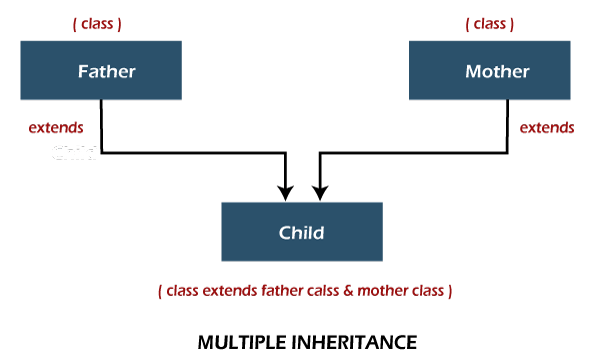
Multiple Inheritance Example
Let’s consider this example to understand why Java do not support multiple inheritance.
//super class 1
class Father{
void quality(){
System.out.println("Discipline");
}
}
//super class 2
class Mother{
void quality(){
System.out.println("Kindness");
}
}
//subclass gives error as it inherits from multiple classes
class Child extends Father, Mother{
public static void main(String[] args) {
Child ch = new Child();
ch.quality();
}
}
Output:

The above code results in compilation error as we call method quality() from the Child class’s object, causing ambiguity.
Java does not allow multiple inheritance to avoid the ambiguity caused by it.
Two main reasons Java do not have multiple inheritance:
Diamond problem:
Consider the following problem, which depicts the multiple inheritance.
- Here, we have class GrandFather having method quality().
- Then the classes Father and Mother derive the method from GrandFather, and both of them override the method quality().
- Now the class Child derives from Father class and Mother class with multiple inheritance. If it calls the method quality(), compiler will be unable to determine which method to call (overridden method of Father class OR overridden method of Mother class). This is called the Diamond problem.
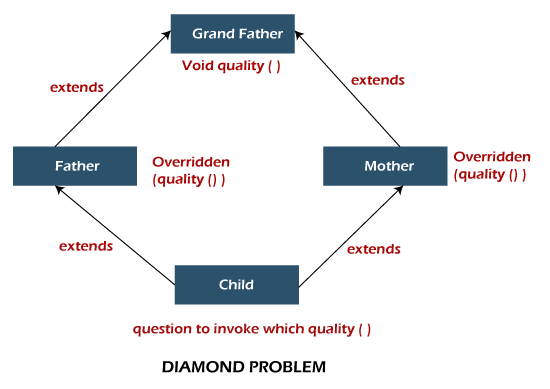
Simplicity:
Multiple Inheritance is not allowed in Java using classes, i.e., a class can only extend only one class. To handle the complexity occurring due to multiple inheritance is complex. Various operations like casting, constructor chaining, etc., are hindered due to it. So to avoid such problems, Java does not have multiple inheritance.
Although Java doesn’t support multiple inheritance, there is a way to resolve the ambiguity caused by the above examples.
Interface in Java
Multiple Inheritance in Java can be achieved by using the interfaces. The interface can be called a blueprint of a class. It contains static constants and abstract methods. By default, the interface variables are static and final, and the methods are abstract.
Java 8 has introduced default and static methods in the interface.
And Java 9 has introduced private methods in an interface.
Declaration of interface
The interface can be declared using the keyword interface. Abstraction is achieved in interfaces as all the methods are abstract, and all the variables are static and final by default.
Note: If a class implements an interface, it must implement all the methods of that interface.
Syntax:
interface interface_name {
//declaring constant variables
//declaring methods
}
Example:
//defining the interface
interface InterfaceTest{
void display();
}
//defining the class and implementing the interface
class Class1 implements InterfaceTest {
public void display(){
System.out.println("Displaying the data");
}
//main method
public static void main(String[] args) {
Class1 obj = new Class1();
obj.display();
}
}
Output:

Multiple Inheritance in Java
When a class implements multiple interfaces or an interface extends multiple interfaces, it is called as multiple inheritance.
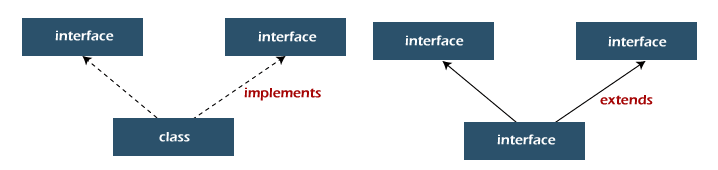
Example 1:
Let’s consider the basic example to use multiple inheritance in Java.
TestInterface.java
//interface 1
interface Display{
//declaring the method
void display_method();
}
//interface 2
interface Show{
//declaring the method
void show_method();
}
//defining the class which implements the interfaces
class TestInterface implements Display, Show {
//defining method of Interface 1
public void display_method(){
System.out.println("Interface name: Display");
}
//defining method of Interface 2
public void show_method(){
System.out.println("Interface name: Show");
}
//main method
public static void main(String[] args) {
TestInterface obj = new TestInterface();
//calling the methods
obj.display_method();
obj.show_method();
}
}
Output:
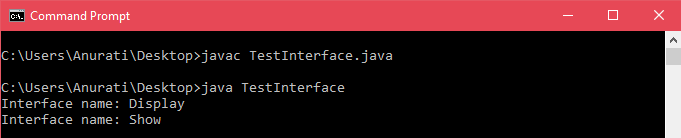
Multiple Inheritance is not supported by classes (due to ambiguity) in Java; however, it is supported by interfaces. It is because the class which implements the interface provides implementation of methods.
Example 2:
Let’s consider the below example where we create two interfaces with the names of the same methods. The methods are declared in the interfaces and implemented in the class.
TestClass.java
//interface 1
interface Interface1{
//declaring the method
void hello();
}
//interface 2
interface Interface2{
//declaring the method
void hello();
}
//defining the class which implements the interfaces
class TestClass implements Interface1, Interface2 {
//defining hello() method
public void hello(){
System.out.println("Interfaces do not cause ambiguity");
}
//main method
public static void main(String[] args) {
TestClass obj = new TestClass();
//invoking the method with class object
obj.hello();
}
}
Output:

In the above example, Interface1 and Interface2 have the same methods hello(); however, its implementation is provided by class ClassTest, so there is no ambiguity.
Default methods:
In Java, a class can implement multiple interfaces. The interfaces provide default implementation of methods. Here, all the interfaces contain default methods with the same signature, so the class that is implementing the interface should explicitly mention which default method to call or simply override the default method.
Example 3:
Consider the below example to implement multiple inheritance using default methods.
TestDefaultMethod.java
//defining first interface
interface Interface1{
//defining default method
default void display(){
System.out.println("This is method of first interface");
}
}
//defining second interface
interface Interface2{
//defining default method
default void display(){
System.out.println("This is method of second interface");
}
}
//class that implements Interface1 and Interface2
public class TestDefaultMethod implements Interface1, Interface2{
//overriding default method display()
public void display(){
//using super keyword to invoke display() of Interface1
Interface1.super.display();
//using super keyword to invoke display() of Interface2
Interface2.super.display();
}
public static void main(String[] args) {
TestDefaultMethod dm = new TestDefaultMethod();
dm.display();
}
}
Output:
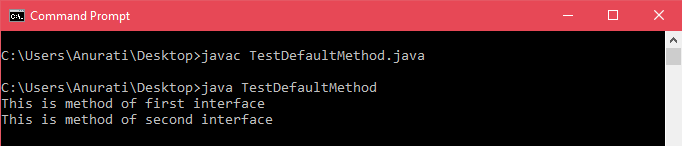
In this way, we have learned how to achieve multiple inheritance in Java with the help of Interfaces.