Crown Pattern in Java
We know the importance of solving pattern problems. We can solve pattern problems by using any programming language. There is no rule to translating into a particular programming language. We can use java, C++, C, python and other programming languages also. We can develop our coding skills and logical thinking by solving the patterns. Mostly each pattern program uses two or more loops. Loops' number depends on the complexity of logic. In these loops, the first loop work for rows and the second loop work for columns. The time and space complexity of the program pattern depends on the number of loops and statements we use and a number of variables we use in the program we have written.
Here, we are going to learn about how to print the crown pattern by using java programming language using loops. We can print crown patterns by using stars, hash, also by using numbers. Like this, we can print the pattern of the crown by using any symbol we want, not only a crown but also other patterns too.
How do we print the crown pattern?
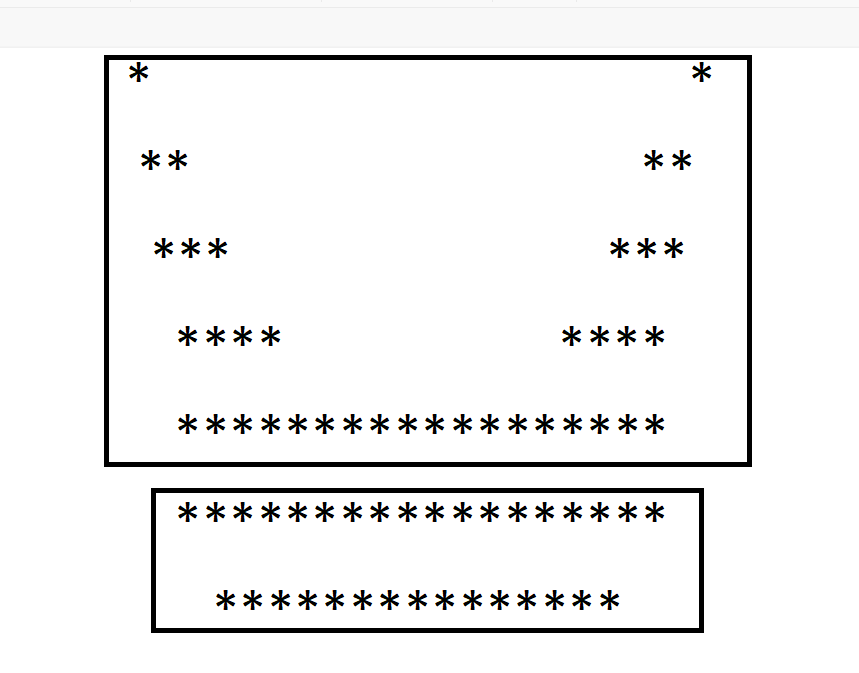
To print the crown pattern, we divide the whole crown into two parts. The reason behind dividing it into parts is to write the code easily and to print the pattern efficiently. If we observe the above picture, we divide the first part into five rows. And the second pattern into two rows.
Approach to follow to print the crown pattern.
- Either we can mention the number of rows, or else we can read the number of rows from the keyboard, after reading store the number of values in an integer.
- By using (rows-1)/2, we can calculate the height of the crown pattern.
- We have to define two loops for printing the pattern.
The first for loop is an outer loop and the second for loop is the inner loop.
- For printing the symbol, we can use the first for loop.
- For printing the row value also, we can make the first for loop.
- Use the below condition for printing the pattern,
if (column == 0) ||column == height||column == row – 1) and (r == height – 1) and
if (column < r||column > height – r) && (column < height + row|| column >= row – r))
else print the spaces in the pattern.
- Now print the symbol that you want according to the loop.
Example 1: Crown Pattern
CrownPatternExampleOne.java
public class CrownPatternExampleOne
{
public static void main(String[]args)
{
for(int i=1; i<=5; i++)
{
for(int k=1; k<=i; k++)
{
System.out.print(" ");
}
for(int j=1; j<=i; j++)
{
System.out.print("#");
}
for(int k=1; k<=3*(4-i+1); k++)
{
System.out.print(" ");
}
for(int j=1; j<=2*i-1; j++)
{
System.out.print("#");
}
for(int k=1; k<=3*(4-i+1); k++)
{
System.out.print(" ");
}
for(int j=1; j<=i; j++)
{
System.out.print("#");
}
System.out.println();
}
for(int i=1; i<=2; i++)
{
for(int k=1; k<=5+i-1; k++)
{
System.out.print(" ");
}
for(int j=1; j<=2*(9-i+1)+1; j++)
{
System.out.print("#");
}
System.out.println();
}
}
}
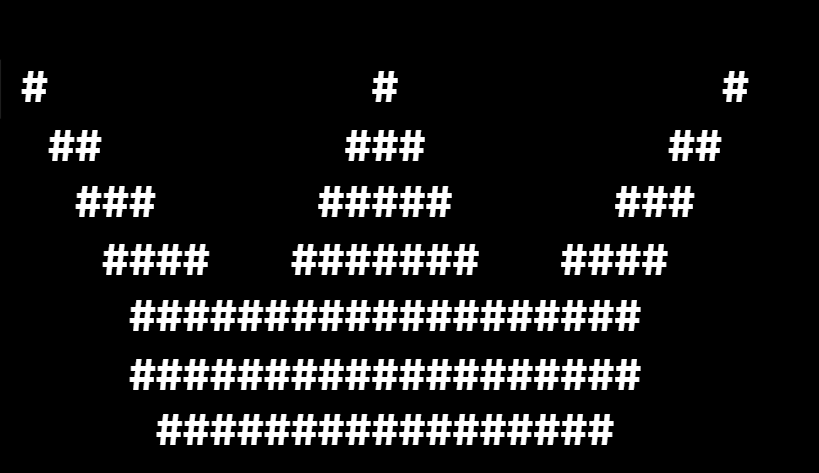
Example 2: Crown Pattern
CrownPatternExampleTwo.java
import java.io.*;
public class CrownPatternExampleTwo
{
static void crown(int length, int height)
{
for (int i = 0; i < height; i++)
{
for (int j = 0; j < length; j++)
{
if (i == 0)
{
if (j == 0 || j == height || j == length - 1)
{
System.out.print("#");
}
else {
System.out.print(" ");
}
}
else if (i == height - 1)
{
System.out.print("#");
}
else if ((j < i || j > height - i) &&
(j < height + i || j >= length - i))
System.out.print("#");
else
System.out.print(" ");
}
System.out.println();
}
}
public static void main (String[] args)
{
int length = 30;
int height = (length - 1) / 2;
crown(length, height);
}
}
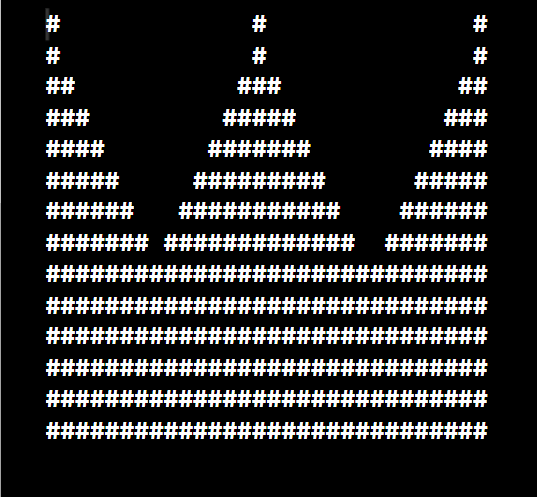
Example 3: Crown Pattern
In this crown pattern program, we can choose the number of rows we want to print.
Let us see the program.
CrownPatternExampleThree.java
import java.util.*;
public class CrownPatternExampleThree
{
public static void main(String args[])
{
int row,r,c,h;
char crown;
Scanner s = new Scanner(System.in);
System.out.print("Enter the number of rows : ");
row = s.nextInt();
System.out.print("Enter character you want print the crown pattern: ");
crown = s.next().charAt(0);
h= (row-1)/2;
for (r = 0; r < h ; r++)
{
for (c = 0; c < row; c++)
{
if (r == 0)
{
if (c == 0 || c == h || c == row - 1)
System.out.print(crown);
else
System.out.print(" ");
}
else if (r == h- 1)
System.out.print(crown);
else if ((c < r || c > h - r) &&(c < h + r || c >= row - r))
System.out.print(crown);
else
System.out.print(" ");
}
System.out.println();
}
}
}
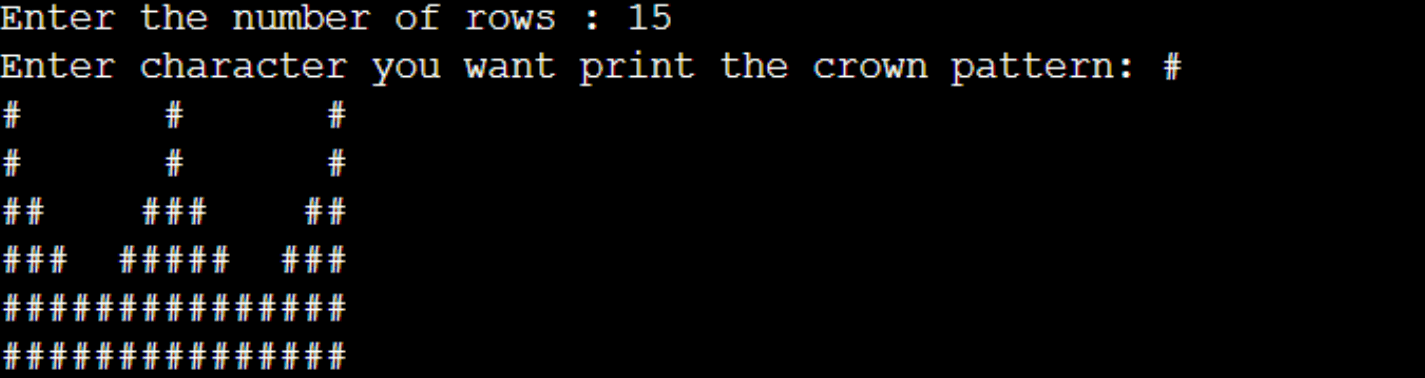