Java 9 Interface Private Method
Java 9 provides us the facility to include private methods inside the java interface. In java 8 and earlier versions, we are supposed to use only constant variables and abstract methods inside the interface. So, we are not allowed to provide implementation inside the java interface. We are allowed to declare private methods inside the interface in java 9. This helps to share code among non-abstract methods. The methods which are declared private in the interface are visible only inside the interface. It is used generally when we want to write confidential code. The default methods in the interface allow us to call private methods declared inside the interface. It is useful to remove redundancy by sharing private method code among default methods. The interface private methods need to have their implementation, they cannot be just declared. The static methods have the right to call only private static methods but the default method can call both static and non static methods.
From java 9 and later version versions interface can have:
- Constant Variables
- Static Methods
- Default Methods
- Abstract Methods
- Private Methods
Java 9 Interface Private Method Example:
interface Demo {
default void display() {
show();
}
private void show() {
System.out.println(" Welcome to Java 9 ");
System.out.println(" Executing private method in an interface ");
}
}
public class PrivateInterfaceImpl implements Demo {
public static void main(String[] args) {
Demo s = new PrivateInterfaceImpl();
s.display();
}
}
Output:
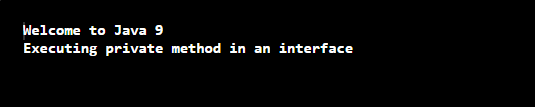
Explanation:
In java 9, we have the facility to declare an interface and include private methods inside it. The interface demo is created with java syntax and one private method show() is created displaying the statements. The default method is created which will call this private method. The main class is created which will implement our interface. Then the object of the interface is created and using the object the default method is called in the interface which will call private method show() and print the statements: "Welcome to Java 9" and "Executing private method in an interface".
Java 9 Multiple Private Method in Interface Example:
interface Demo {
default void display() {
show();
end();
}
private void show() {
System.out.println(" Welcome to Java 9 ");
System.out.println(" Executing private method in an interface ");
}
private void end()
{
System.out.println(" Explore more java 9 features ");
}
}
public class PrivateInterfaceImpl implements Demo {
public static void main(String[] args) {
Demo s = new PrivateInterfaceImpl();
s.display();
}
}
Output:

Explanation:
In java 9, we have the facility to declare an interface and include two or more private methods inside it. The interface demo is created with java syntax and two private methods show() and end() are created displaying the statements. The default method is created which will call this private method one after the another. The main class is created which will implement our interface. Then the object of the interface is created and using the object the default method is called in the interface which will call private method show() and print the statements: "Welcome to Java 9" and "Executing private method in an interface". Then it will call the end() method and print the statement: "Explore more java 9 features".
Java 9 Private Static Method in Interface Example:
interface Demo {
static void display1()
{
printLines();
System.out.println(" This is method1 ");
}
static void display2()
{
printLines();
System.out.println(" This is method2 ");
}
private static void printLines() {
System.out.println(" Starting execution of the method ");
}
default void print() {
display1();
display2();
}
}
public class Test implements Demo{
public static void main(String args[]) {
Test e = new Test();
e.print();
}
}
}
Output:

Explanation:
We can also declare static and private static methods inside the interface in java. We have declared one default method calling two static methods which will first call the private static method and then print the statements. The class will implement the interface and using object call the default method print() which will call static methods display1() and display2() which again calls private static methods printLines() which will print the statements starting execution of the methods and then print respective static methods content that is: "This is method1" and "This is method2".