Zig Zag star and Number Pattern in Java
We covered many Java pattern applications in the preceding part. We will write Java applications for zigzag star and number patterns in this part.
Printing Zig Zag Number Pattern Steps
- Print one backslash first, followed by one forward slash, then keep going.
- Put the number of characters in such a row into the integer variable row.
- Enter count as an integer variable to hold the overall number of zigzags.
- Take the first for iteration to print all the rows while printing backward slashes.
- Use an inner loop to output the values for the columns.
- Afterward, keep printing the numbers in accordance with the iteration.
- Take the first iteration to print all rows in order to print the forward slash.
- Print all column values using an inner loop.
- Afterward, keep printing the numbers in accordance with the iteration.
Let's put the aforementioned actions into a Java program.
// ZigZagStar1.java
import java.util.Scanner;
public class ZigZagStar1
{
public static void main(String[] args)
{
// Consider user input regarding no for rowscd desktop
System.out.print(" Type in how many characters are on a line: ");
Scanner sc = new Scanner(System.in);
int row,coloumn,row1;
//taking the initial ASCII value 64
int ascii=64;
// row of storage for the supplied value
row1 = sc.nextInt();
System.out.print(" Type the number of zigzag lines here: ");
int c = sc.nextInt();
for (int j=1;j<=c;j++)
{
// backward
for(row=1; row<=row1; row++)
{
// number-printing loop
for(coloumn=1; coloumn<=row1; coloumn++)
{
// row and column must have the same value. type a symbol
if(row == coloumn)
System.out.print(row+" ");
else
System.out.print(" ");
}
System.out.println("");
}
// forward
for(row=1;row<=row1;row++)
{
// number-printing loop
for(coloumn=1;coloumn<=row1;coloumn++)
{
// if column Equals row 1+1-row, print symbol; otherwise, display spaces.
if(coloumn <= (row1+1-row))
{
if( coloumn == (row1+1-row) )
System.out.print(row+" ");
else
System.out.print(" ");
}
}
System.out.println("");
}
}
}
}
Output:
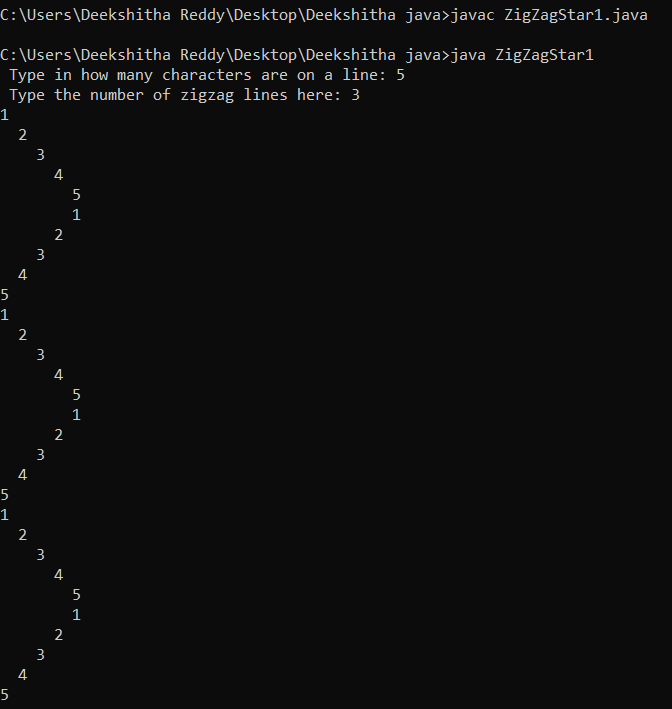
Zig Zag Star pattern
// ZigZagpattern.java
import java.util.Scanner;
public class ZigZagpattern
{
public static void main(String[] args)
{
// Consider user input regarding no for rowscd desktop
System.out.print(" Type in how many characters are on a line: ");
Scanner sc = new Scanner(System.in);
int row,coloumn,row1;
//taking the initial ASCII value 64
int ascii=64;
// row of storage for the supplied value
row1 = sc.nextInt();
System.out.print(" Type the number of zigzag lines here: ");
int c = sc.nextInt();
System.out.print(" Type any Symbol: ");
char s=sc.next().charAt(0);
for (int j=1;j<=c;j++)
{
// backward
for(row=1; row<=row1; row++)
{
// number-printing loop
for(coloumn=1; coloumn<=row1; coloumn++)
{
// row and column must have the same value. type a symbol
if(row == coloumn)
System.out.print(s+" ");
else
System.out.print(" ");
}
System.out.println("");
}
// forward
for(row=1;row<=row1;row++)
{
// number-printing loop
for(coloumn=1;coloumn<=row1;coloumn++)
{
// if column Equals row 1+1-row, print symbol; otherwise, display spaces.
if(coloumn <= (row1+1-row))
{
if( coloumn == (row1+1-row) )
System.out.print(s+" ");
else
System.out.print(" ");
}
}
System.out.println("");
}
}
}
}
Output:
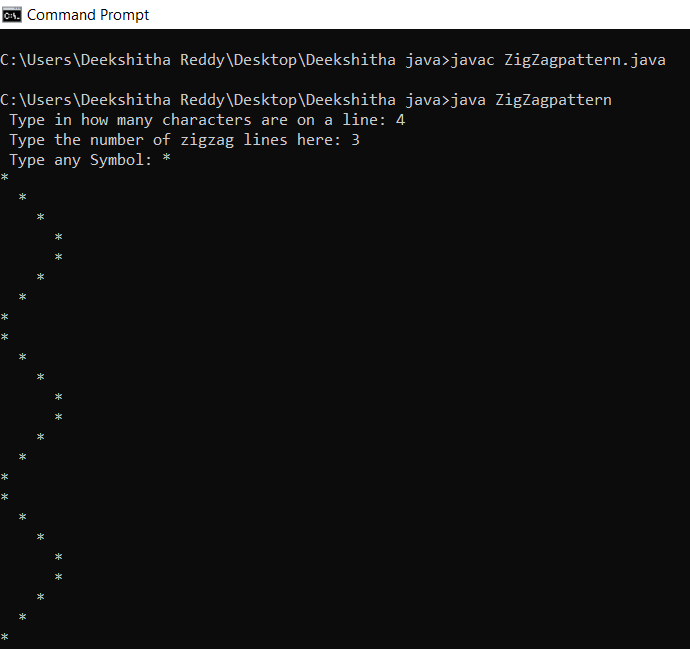