Program to find the duplicate characters in a string
Problem statement
You have given with a string and your task is to find out the repeated characters from the string and print them. If no character is repeated, then you need to output “no character is repeated in the given string”.
Example
Consider the string “javaprogramming”, the repeated characters are a, r, g, m. Therefore, you need to output a, r, g, m.
import java.io.*;
import java.util.*;
public class Main
{
public static void main (String [] args) {
Scanner sc = new Scanner ( System.in ) ;
HashMap <Character,Integer> p = new HashMap<> ();
// creating hashmap to store the individual character as Key
// and storing count of each character as value of hashmap
System.out.println(“enter the string:”);
String s = sc.next ();
for (int i = 0; i < s.length (); i++)
{
if (p.containsKey (s.charAt (i)))
{
p.put (s.charAt (i),p.get (s.charAt (i))+1);
// increment count if character already exists in hashmap
}
else
{
p.put (s.charAt (i),1);
// inerting characters into hashmap
}
}
System .out. println ("repeated characters are");
for (int i = 0; i < s.length (); i++)
{
if (p.get (s.charAt (i)) >1)
{
System .out. println (s.charAt(i));
p.put (s.charAt (i),0);
// making the count of traversed character to zero
// to prevent repating of same character (on output ).
}
else
{
System.out.println("no chacter is repeated in the given string");
}
}
}
}
Time complexity for this code is O(n*log(n)) where n is the length of the string and it take O(log(n)) for the insertion into the map.
Space complexity of this code is O(k), k is the size of map.
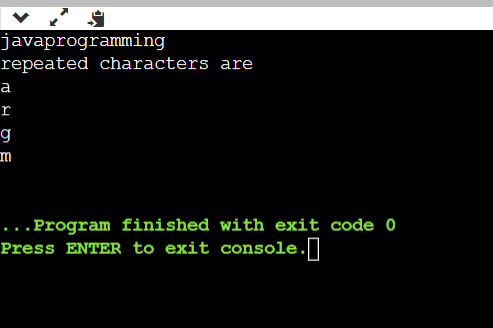
Example 2
import java .io. *;
import java .util. *;
public class Main {
public static void main (String [ ] args) {
Scanner sc = new Scanner (System.in);
System.out.println("enter the string:");
String str = sc.next ();
int count ;
int found=0;
// Convert the given string into character array
char str1 [ ] = str.toCharArray ();
System.out.println ("characters repeated in the given string: ");
// Counts each character present in the string
for (int i = 0; i <str1.length; i++) {
count = 1;
for (int j = i+1 ; j < str1.length; j++) {
if (str1 [ i ] == str1 [ j ]) {
count++;
// Set str [j] to 0 to avoid printing visited character
str1 [ j ] ='0';
}
}
// A character is considered as duplicate if count is greater than 1
if (count > 1 && str1[i] != '0')
{
System.out.println (str1 [ i ] );
found++;
}
}
if(found==0)
{
System.out.println ("no character is repeated in the given string");
}
}
}
Output
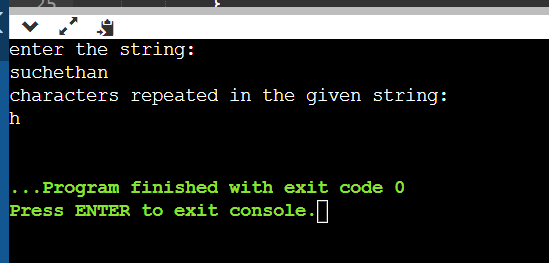
If user gives an input that string does not have repeated character like browncat, the output will loke like shown in the below:
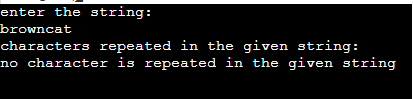