Thread Concept in Java
What is a Thread?
A subprocess that is lightweight in Java is known as a thread. It is the smallest unit of a process. For the running of multiple tasks parallelly we can use threads. Before we were unable to run multiple tasks at the same time. To overcome that drawback, the thread concept was introduced. A thread is similar to a program. It is a subpart of a program. And it has a flow of control.
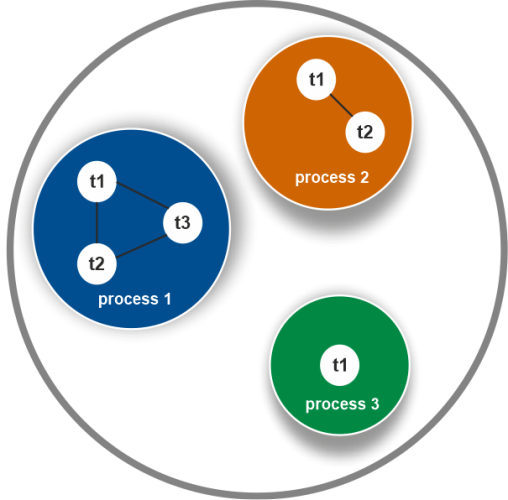
As shown in the above figure, inside a process a thread is executed. Inside the OS (Operating system) we will have multiple processes. And inside each process, we will have multiple threads.
Advantages of Threads
- Threads are independent.
That means threads have a separate path for execution. If we have an exception in one thread, all the remaining threads are not affected.
- Threads use a shared memory area.
Java Thread Class
To achieve thread programming, we have a " Thread class " in Java. This Thread class provides many methods as well as constructors to perform and create operations on the thread.
Thread methods in Thread class
Many methods are provided by the Thread class. A few of them are listed below.
S.No | Return Type | Method Name | Description |
1 | void | start() | Execution of the thread will start when we use this method. |
2 | void | run() | For doing an action to a thread this method is used. |
5 | int | getPriority() | The priority of the thread is returned by this method. |
9 | int | getId() | This method gives the ID of the thread as output. |
10 | Boolean | isAlive() | This method returns true if the thread is alive or else it returns false. |
11 | void | suspend() | A thread is suspended using this method. |
12 | void | resume() | To resume the suspended thread, we use this method. |
13 | void | stop() | A thread is stopped by using this method. |
14 | void | interrupt() | To interrupt the thread, we use this method. |
15 | Boolean | isInterrupted() | If a thread is interrupted, then it returns true, or else this method returns false. |
16 | Static Boolean | interrupted() | To check whether the current thread is interrupted or not we use this method. |
17 | void | notify() | To notify only one thread that is waiting for a particular object we use this method. |
18 | void | notifyAll() | To notify all the threads which are waiting for particular objects we use this method. |
These are the most used methods of the Thread class.
Constructors of Thread class:
- Thread()
- Thread(Runnable r)
- Thread(String name)
- Thread(Runnable r, String name)
The life cycle of a Thread or Thread States
The Thread goes through 5 stages. They are
Stage 1: New
Stage 2: Runnable
Stage 3: Running
Stage 4: Non-runnable
Stage 5: Terminated
But according to Sun, a thread has only four stages that are only new stage, the runnable stage, the non-runnable stage, and terminated stage. There is no running stage in the life cycle of the thread. But for better understanding, we have to use the running stage. The JVM ( Java virtual machine ) controls the entire life cycle of the thread.
By using a figure, we can easily understand the life cycle of a thread.
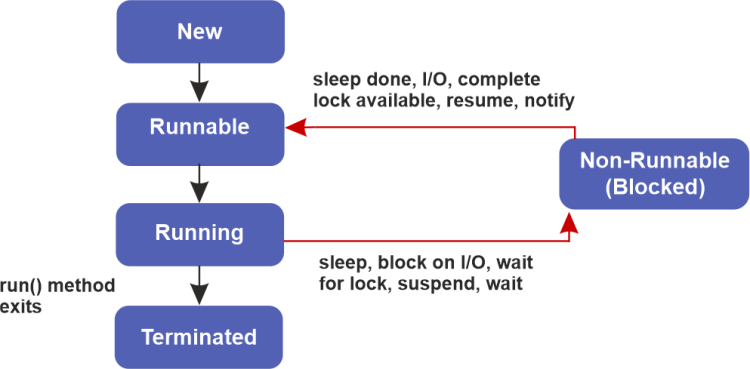
Now let us understand each stage clearly,
- New
Before invoking the start() method an instance of a Thread class is created then the Thread is said to be in the new stage. - Runnable
After the invocation of the start() method, we can say that a thread is in the runnable stage. - Running
The thread scheduler has selected a thread means it is said to be in the running stage. - Non – Runnable
This stage can also be called as Blocked stage. At this stage, the thread is not eligible to run currently but is still alive. - Terminated
This method is also known as the dead stage. When the run() method exists the thread is terminated.
How to create a thread?
Two ways are available to create a thread, they are:
- By extending the Thread class.
- By implementing a Runnable interface.
Creation 1
ThreadCreation.java
class ThreadCreation extends Thread
{
public void run() // run method
{
System.out.println(" The thread is running... ");
}
public static void main(String args[])
{
ThreadCreation tc = new ThreadCreation();
tc.start(); // start method
}
}
Output:

Now let’s understand how to create a thread by implementing a runnable interface by using an example.
ThreadCreation1.java
class ThreadCreation1 implements Runnable
{
public void run() // run method
{
System.out.println(" The Thread is running ... ");
}
public static void main(String args[])
{
ThreadCreation1 tc = new ThreadCreation1();
Thread t1 = new Thread(tc);
t1.start(); // start method
}
}
Output:
