What’s new in Java 12
On March 19th, 2019, the Java 12th edition was released. After releasing this edition, they have decided to release every new edition every six months. This version is the advanced or updated version of JDK 11. Here, we will learn about the high-level performance of Java 12 and its new features.
Features and Language changes
A lot of new language features are available in Java 12 which are more advanced than Java 11. Some of the features are listed below.
- String class new methods
- File:: Mismatch method
- Teeing collector
Now let us discuss each of them in brief with the code as well as output.
String class new methods
In the updated version that is in the Java 12 version, two new methods are there in the Java String class. The two new methods are
- indent ()
- transform ()
Let us see about each method in brief.
indent () Method
This method takes the parameters and gives indentation for each line depending upon the parameter or number we give in the method. If we give numbers or parameters greater than zero or a positive number, then at the beginning of each line spaces will be added. Or else, if we give a negative number that is a number less than zero then this method will remove spaces from the beginning of the line. If the text does not have sufficient white spaces, then all the leading white characters are removed.
Now let us take an example first we will try to add spaces and then we will try to remove the spaces.
IndentExpl.java
// importing the packages
import java.util.*;
import java.lang.*;
import java.io.*;
class IndentExpl
{
public static void main (String[] args) throws java.lang.Exception
{
String txt = " Hello JavaTpointers !\n This is an updated version of Java. Welcome to Java 12.";
txt = txt.indent(4); // using the indent() method. Giving a positive number means spaces will be added
System.out.println(txt); // printing the txt
txt = txt.indent(-10); // using the indent() method. Giving a negative number means spaces will be reduced.
System.out.println(txt); // printing the txt
}
}
Output:
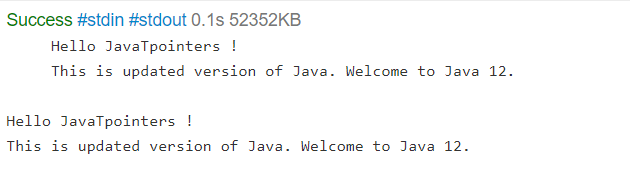
Code Explanation:
In the above code firstly, we have imported the packages they are util.*, lang. * and io.*. Next, we created a class called IndentExpl. Inside the main method, we have declared a string txt. Next, we used the indent () method. First, we have given a positive number 4, so the spaces are added to the txt. In the next method, we have given the negative number. Which means spaces will be deleted. Here in the program, we have given -10 which exceeds our spaces, but only the spaces present there are removed. Text is not affected by the number exceeding.
transform () method:
As the name of this method indicates that we can transform a string. Here this new function of Java 12 accepts only one parameter as input and returns the transformed form as the output.
Let’s understand more about this function using a program along with output.
import java.util.*;
import java.lang.*;
import java.io.*;
class TransformExpl
{
public static void main (String[] args) throws java.lang.Exception
{
String s = "Welcome, To, JavaTpoint";
List l = s.transform(s1 -> {return Arrays.asList(s1.split(","));});
System.out.println(l);
}
}
Output:

Code Explanation:
In the above code firstly, we have imported the packages that are the package util.*, lang.* and io.*. Next, we have created a new class that is TransformExpl. Inside the main method, we have declared a string s. Next, we have transformed the string to the list l. And we have used the split() function to split the txt. Hence, we got the output as displayed in the above pic.
File:: Mismatch method
In the “ nio.file.Files utility ” class java 12 version came with a new mismatch method.
public static long mismatch(Path path, Path path2) throws IOException
Generally, this method is used to compare two files and the first mismatched byte is returned as output. If the files are the same means, we will get -1L as output. If there is any mismatch in the file, then the output will be in the range of 0L to the byte size of the smaller size.
let us understand more about this method using an example syntax. In the first program, we will create two same files to get the output as -1L. And next, we will
Path path1 = Files.createTempFile("file1", ".txt");
Path path2 = Files.createTempFile("file2", ".txt");
Files.writeString(path1, " Hello ! Welcome to JavaTpoint ");
Files.writeString(path2, " Hello ! Welcome to JavaTpoint ");
long mismatch1 = Files.mismatch(path1, path2); // using mismatch method
System.out.println(
" Below the mismatch position in a file is returned. If the files are the same, then -1 is returned. ");
System.out.println(
" In file 1 and file 2 the mismatch position is at : "
+ mismatch1);
Path path3 = Files.createTempFile("file3", ".txt");
Path path4 = Files.createTempFile("file4", ".txt");
Files.writeString(path3, " Hello ! Welcome to JavaTpoint ");
Files.writeString(path4, " Hello ! Welcome to Tutorial and Example ");
long mismatch2 = Files.mismatch(path3, path4); // using mismatch method
System.out.println(
" In file 3 and file 4 the mismatch position is at : "
+ mismatch2);
Explanation:
We have created two strings and those strings are the same. Next, we used the method mismatch to check whether the texts have any mismatches or not. Since these are the same files, we will not get any mismatch and we get -1L as output. Next also we have created different texts and used this mismatch method since they are different texts, we will get the position of the mismatched place.
Teeing Collector
To the Collectors, class teeing collector is added in the new version of Java which is Java 12.
Here is the syntax of teeing collector. Through this syntax, we can easily understand about teeing collector.
Collector<T, ?, R> teeing(Collector<? super T, ?, R1> downstream1,
Collector<? super T, ?, R2> downstream2, BiFunction<? super R1, ? super R2, R> merger)
Here in the syntax of teeing collector, we use two downstream collectors. So, each element by using both the downstream collectors they are processed. Now the processed elements are sent to the merge function. Inside the merge function, the elements are merged, and the final output is produced.
The teeing collector contains three components they are two collectors and one bi function. So input is given to the collectors, and we will receive the output through the bifunction.
Let us understand more about this teeing collector using a syntax example.
double meanofthedigits = Stream.of(10,5,2,6,1)
.collect(Collectors.teeing(
summingDouble(i -> i),
counting(),
(sum, n) -> sum / n));
System.out.println(meanofthedigits);
Here we have given numbers to the collector. Next, we want to find the mean of the digits. We have calculated the mean and the output will be 4.8.