Advantages of Generics in Java
Generic offers a variety of benefits. The programmer's life is made easier by using generic Java. In this section, we are going to discuss about Java's generic’s and its benefits.
1. Type-safety: Suppose, we have accidentally inserted an Integer value to an ArrayList that you intended to use to hold the names of books. The error is thus generated at runtime by the Compiler. The issue arises when we try to get it even if the Compiler permits it.
The Compiler displays the faults at build time rather than run time, thanks to generic. Because the problem is hard to spot during runtime, it saves the programmer’s time. Finding mistakes at build time is usually preferable to doing so at run time.
Let’s see a Java program without using Java generic.
GenericsInJava.java
import java.util.ArrayList;
class GenericsInJava
{
public static void main(String[] args)
{
// List of Arrays with no types
ArrayList ListOfNames = new ArrayList();
ListOfNames.add("Abhi");
ListOfNames.add(“Keshav");
ListOfNames.add(18); // The compiler enables this
String s1 = (String)ListOfNames.get(0);
String s2 = (String)ListOfNames.get(1);
// generates a runtime exception
String s3 = (String)ListOfNames.get(2);
}
}
Output:
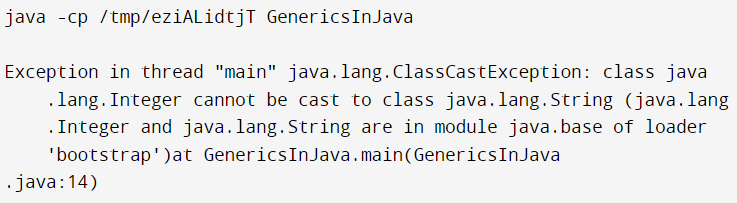
Let’s understand the above program with generic concepts.
GenericsInJava.java
import java.util.ArrayList;
class GenericsInJava
{
public static void main(String[] args)
{
// ArrayList with string type
ArrayList<String> ListOfNames = new ArrayList<String>();
ListOfNames.add("Abhi");
ListOfNames.add(“Keshav");
ListOfNames.add(18); // Compiler doesn't allow this
String s1 = (String)ListOfNames.get(0);
String s2 = (String)ListOfNames.get(1);
String s3 = (String)ListOfNames.get(2);
}
}
Output:
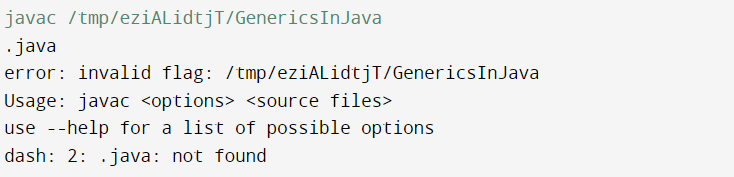
java.lang.Error exception in thread "main" Unsolved compilation issue error The parameters (int) at GenericsInJava.main is not relevant for the function add(int, String) in the type ArrayListString> (GenericsInJava.java:12)
2. Typecasting is not required: We should typecast the data while obtaining the information from the ArrayList, as you can see in the program above. If we are not using a generic ArrayList. The typecast is necessary every time. For programmers, it's a hectic problem.
Since we don't have to cast it each time if we already know the data type list, the generic addresses this problem. It is one of the best benefits of using generics in Java.
3. A generic method, class, and interface can be created to reuse the code. The Collection framework provides a wide variety of generic classes. Depending on the type of data, we can utilize them.
Program.java
import java.util.HashMap;
import java.util.Map;
class Program
{
public static void main(String[] args)
{
// Integer as the key and String as the value in a map
Map<Integer, String> mapIntString = new HashMap<Integer, String>();
mapIntString.put(1, "HI");
mapIntString.put(2, "LEARN");
System.out.println("Map of Value Forms");
for(Integer key : mapIntString.keySet())
System.out.println(mapIntString.get(key));
// Map containing Integer as key and Float as Value
Map<Integer, Float> mapIntFloat = new HashMap<Integer, Float>();
mapIntFloat.put(1, 1.8f);
mapIntFloat.put(2, 2.1f);
System.out.println("Map of Value Forms");
for(Integer key : mapIntFloat.keySet())
System.out.println(mapIntFloat.get(key));
}
}
Output
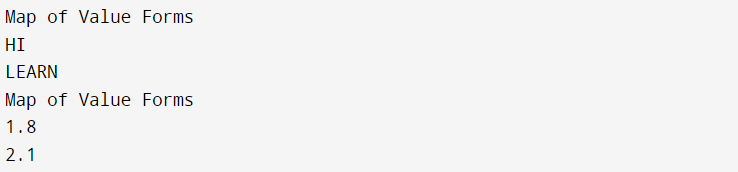
Generics guarantee compile-time safety, enabling the programmer to identify erroneous types while the code is being compiled.
Java Generics allows programmers to reuse their code for whatever type they want. The sorting of an array of objects may be accomplished by a programmer writing a generic procedure. Using generics, a programmer may apply the same logic to String, Double, and Integer arrays.
The absence of the need for individual typecasting is another benefit of utilizing generics. The programmer defines the starting type, which defers the code's operations.
We can use it to create custom algorithms.
Using generics in Java has several benefits, including the following:
- Reusability is the primary benefit of generic medications. The same code may be applied to several data types.
- Type safety is another benefit of generic.
- Typecasting may be done away with by using generics.
- It aids in preventing runtime ClassCastException.
Generics provide type security
Generics only permit holding only one kind of thing: This aids in the early detection of mistakes if a new kind of argument is supplied during compilation.
Generics enable code recycling: For instance, you will be required to build many classes if you wish to construct a class that displays the class of parameterized types such as Integers, Doubles, Strings, or even Characters, but with generics, there would only be one class.
The casting of the particular type is not needed for generics: Since generics offer the parameterized type that references an argument, type casting is unnecessary when using them.
Generics aid in error detection during compilation as opposed to runtime.