The final Keyword in Java
The final keyword is employed in several instances. Firstly, the non-access modifier final only applies to variables, methods, and classes. The final can be used in the following situations.
Final Variables
When the final keyword is used to declare a variable, it becomes a constant because its value cannot be changed. The initialization of a final variable is also required in this case. If the final variable is a reference, it cannot be re-bound to reference another object. Still, the internal state of the object pointed by that reference variable can be changed, allowing you to add or remove elements from the final array or final collection. Final variables should be written in all capital letters, with underscores used to separate words, as this is considered good practice.
Example 1
final int P = 5 ;
// Final variable
Example 2
final int P ;
// Blank final variable
Example 3
static final double PI = 3.141592653589793 ;
// Final static variable PI
Example 4
static final double PI ;
// Blank final static variable
Initializing a final Variable
A final variable needs to be initialized; otherwise, the compiler will raise a compile-time error. An initializer or assignment statement can only be used once to initialize a final variable. A final variable can be initialized in any one of the three ways:
- When a final variable is declared, it can be initialized. The most typical strategy is this one. A final variable not initialized during declaration is a blank final variable. The two methods for initializing a blank final variable are listed below.
- An instance-initializer block or the constructor are acceptable places to initialize a blank final variable. If the class has more than one constructor, it must be initialized in each one, or a compile-time error will be thrown.
- Within a static block, a blank final static variable could be initialized.
Filename: FinalExample.java
// Java Program to Showcase Various Initialization Methods for a Final Variable
// the Main class
class FinalExample {
// a final variable
// direct initialize
final int P = 15;
// a blank final variable
final int C;
// another blank final variable
final int M;
// a final static variable PI
// direct initialize
static final double PI = 3.141592653589793;
// a blank final static variable
static final double E;
// instance initializer block for
// initializing C
{
C = 25;
}
// static initializer block for
// initializing E
static{
E = 2.3;
}
// constructor for initializing M
// Note that if there is more than one
// constructor, you must initialize M
// in them also
public Example1()
{
M = -1;
}
public static void main(String args[]){
System.out.println(PI);
System.out.println(E);
}
}
Output
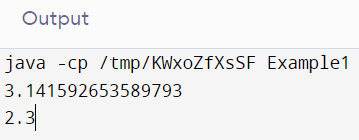
The above-displayed output prints the PI and E. P, C, and M values that cannot be printed because they are not defined with the static keyword.
When should a final variable be used?
The only distinction between a normal and a final variable is that a standard variable's value can be changed. In contrast, a final variable's value cannot be altered once assigned. Therefore, only values we want to remain constant throughout the program's execution should be stored in the final variables.
What is the reference final variable?
The term "reference final variable" refers to a final variable that contains a reference to an object. A final StringBuffer variable, for instance, is defined as follows:
final StringBuffer t;
As everyone is aware, a final variable cannot be changed. However, a reference final variable allows for altering the internal state of the object to which it refers. Keep in mind that this is not a reassignment. Non-transitivity is the name for this feature of a final variable. Look at the example below to better understand what is meant by the phrase "internal state of the object":
Filename: Example2.java
//Java program to display the reference of a final variable.
// Main class
class Example2 {
// Main driver method
public static void main(String[] args)
{
// making an object of the class StringBuilder
// Final reference variable
final StringBuilder s = new StringBuilder("Hello");
// Printing the element in the StringBuilder object
System.out.println(s);
s.append(" World");
//Printing the element in StringBuilder once more after appending the above
//element to the object
System.out.println(s);
}
}
Output
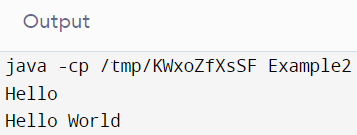
The above-displayed output prints "Hello" and "Hello World" strings.
Since arrays are objects in Java, the non-transitivity property also applies to them. Final arrays are another name for arrays with the final keyword.
Note: As was previously mentioned, it is not permitted to rename a final variable; doing so will result in a compile-time error.
Filename: Example3.java
//Re-assigning a final variable in a Java program
//will throw an Error during compilation
// Main class
class Example3 {
//Creating a static final variable
//and initializing it as needed.
static final int C = 4;
// Main driver method
public static void main(String args[])
{
// Reassigning a final variable will
//result in a compile-time error.
C = 5;
System.out.println(C);
}
}
Output
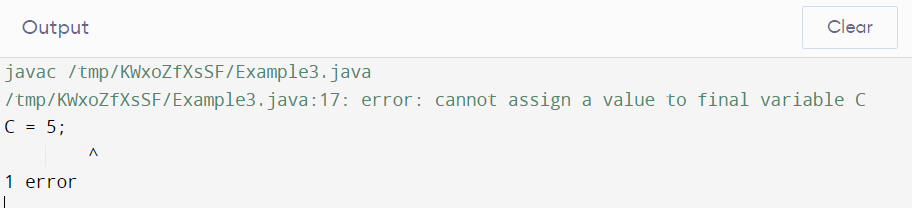
The above-displayed output displays an error when a value is re-assigned to the variable C.
Note: A final variable is called a local final variable when it is formed inside a method (function), constructor, or block. Local final variables must initialize once at the location of creation. For the local final variable's program, see below.
Filename: Example4.java
//Java example program for local final variable
// Main class
class Example4 {
// Main driver method
public static void main(String args[])
{
// local final variable declaration
final int i;
//Initializing it with an integer value at this point
i = 10;
//The value is printed on the console.
System.out.println(i);
}
}
Output
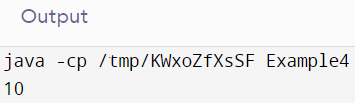
The above-displayed output shows the value 10.
Before continuing, keep in mind the following crucial points, which are given below:
- Be aware of the distinction between Java final variables and C++ const variables. In C++, const variables must have a value when declared. As we can see from the examples above, it is not required for final variables in Java. Later on, a final variable can only be given a value once.
- Using the final with foreach loop results in a legally valid statement.
Filename: Example5.java
//Java program to show a final with a for-each statement
// Main class
class Example5 {
// Main driver method
public static void main(String[] args)
{
//Declaring and creating a unique integer array
int arr[] = { 1, 2, 3 };
// final with for-each statement
// legal statement
for (final int i : arr)
System.out.print(i + " ");
}
}
Output
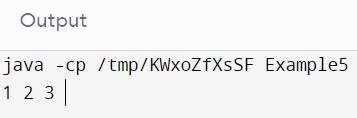
The above-displayed output prints the array elements.
Since the "i" variable exits the loop with each iteration, it is effectively declared again, enabling the usage of the same token I to denote numerous variables.
Final classes
A class is referred to as a final class if the keyword final is used in its declaration. There is no way to extend a final class (inherited).
A final class can be employed in two ways.
- Given that final classes cannot be expanded, one is sure to avoid inheritance. For instance, all final classes, such as Integer, Float, etc., are wrapper classes. They are not extendable.
final class A
{
// methods and fields
}
// The following class is prohibited
class B extends A
{
// COMPILE-ERROR! A cannot be a subclass
}
- Final can also be used with classes to create immutable classes, such as the default String class. Making a class final is necessary to make it immutable.
Final Methods
A method is referred to as a final method if the term final is used in its declaration. An ultimate approach cannot be changed. This is done by the Object class, which has a few final methods. Methods with the same implementation across all derived classes must be declared using the final keyword.
Filename: Example6.java
class B {
// create a final method
public final void display() {
System.out.println("This is a final method.");
}
}
class Example6 extends B {
// trying to override the final method
public final void display() { //final keyword with a method
System.out.println("The final method is overridden.");
}
public static void main(String[] args) {
Example6 obj = new Example6();
obj.display();
}
}
Output
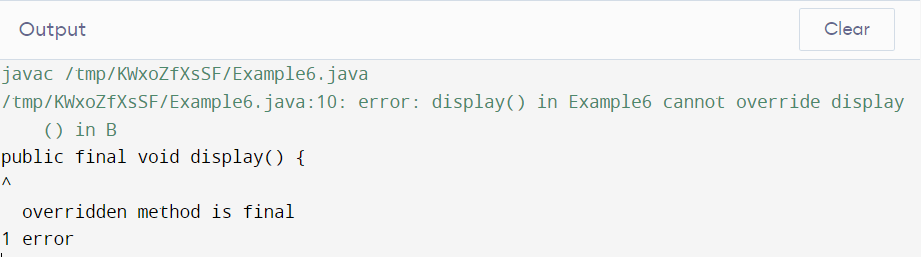
The above-displayed output displays an error that occurs when the final method is set to override.