How to take Multiple String Input in Java using Scanner class
Scanner class is a class which takes the multiple input data or the single input data through an objects and methods. The Scanner class will be available in the java.util package.
In this article we are excited to learn how to read the multiple string input in the java using the Scanner class.
The Methods in the Scanner class which we invoke in the data types with respect to the methods:
DATA TYPES | METHODS |
Integer | nextInt() |
Floating point | nextFloat() |
Boolean | nextBoolean() |
String | nextLine() |
Character | next().charAt(0) |
Syntax for creating the Scanner class
Classname objectname = new Classname(parameters);
Scanner sc = new Scanner();
Here we are using the Scanner as the Class name and sc as the object name.
Now we will see the data types with the examples in java.
For writing the programs we mainly use some data types. I.e., String data type, Integer data type, Floating data type, Character data type etc...
Java nextInt() Method:
The java nextInt() method moves the Scanner class to the next integer value. It reads the integer input data.
Java nextFloat Method:
The java nextFloat() method moves the current scanner class to the next Floating-point value. It reads the floating-point values.
Java nextBoolean() Method:
The java nextBoolean() method moves the current class to the next Boolean values. The scanner class reads the Boolean values as true or false.
Java nextLine() Method:
The java nextLine() moves to the current scanner class to the next line. The java scanner class reads the String input data including the spaces between the words.
Java next.charAt(0) Method:
The next.charAt(0) method is similar as the nextLine() method. The only difference is that it reads the character data and it doesn’t read the spaces between the words.
Examples
Let's see a simple example program for reading the person data using scanner class.
Example 1:
import java.util.Scanner;
public class Demo // class name
{
public static void main(String[] args) // main method
{
Scanner ss = new Scanner(System.in); // scanner class
System.out.print("Enter the number of characters:");
String[] str1 = new String [ss.nextInt()];
sc.nextLine();// nextLine() Method
for (int i = 0; i < str1.length; i++) // initializing for loop
{
str1[i] = ss.nextLine();
} //i
System.out.println("The character you have to entered");
for(String str: str1)// for-each loop syntax
{
System.out.println(str);
}// for
} // Demo
}// main
OUTPUT:
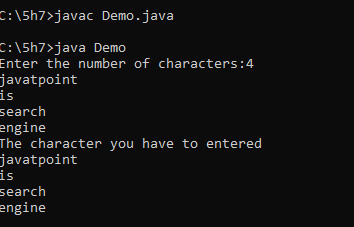
In the above example we consider the multiple string inputs. We used the methods like nextLine() for moving the next current line.
Example 2:
import java.util.Scanner;
public class Demo // class name
{
public static void main(String[] args)// main method
{
int n=5;
System.out.println("Enter the elements: ");
Scanner sc = new Scanner(System.in); // scanner class
for(int i = 0; i < n; i++) // initializing for loop
{
String str1 = sc.nextLine();// nextLine() method
System.out.println(str1);
}
}
}
OUTPUT:
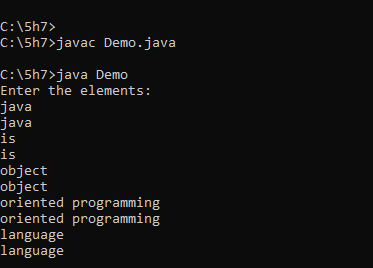
In the above java example, we taken the multiple string inputs. In this example we used the nextLine() method. This method it returns the true statement if the another nextLine() method of the scanner class is currently in use. In the following code the for loop executes until the method returns the false.
Example 3:
import java.util.Scanner;
public class Demo11 // class name
{
public static void main(String[] args) // main method
{
Scanner scn = new Scanner(System.in);// scanner class
while(scn.hasNextLine()) //while loop with nextLine() method
{
System.out.println(scn.nextLine());
}
}
}
Output:
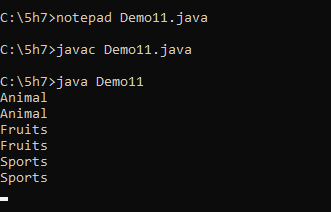
In the above java example, we taken the multiple string inputs. In this example we used the nextLine() method. This method it returns the true statement if the another nextLine() method of the scanner class is currently in use. In the following code the while loop executes until the method returns the false.
Example 4:
import java.util.Scanner;
public class Demo13
{
public static void main(String[] args)
{
String name;
int id, age;
Scanner sc = new Scanner(System. in );
System.out.println("please enter the person details");
System.out.println("Enter your name");
name = sc.nextLine();
System.out.println("Enter your id");
id = sc.nextInt();
System.out.println("Enter your age");
age = sc.nextInt();
System.out.println("You have entered the following person details:");
System.out.println("Name: " + name);
System.out.println("Id: " + id);
System.out.println("Age: " + age);
}
}
Output:
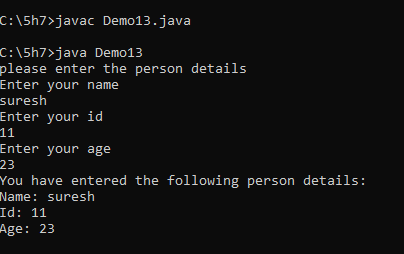
This is the basic scanner class program for the beginner level students. Here we are using two methods like nextLine() for the String inputs and second Method nextInt() for the integer inputs.