Java Full Stack
A person who can create both the front end and back end of an application is a full-stack developer. In essence, the term "Java full-stack" refers to a web developer who uses Java to build the entire technology stack. An engineer known as a full stack developer works on both the client and server sides of the software application. This kind of developer works on a software application's Full Stack.
"A candidate who can design both client and server software" is the simplest definition of a full-stack developer. If a developer is full-stack, they handle all the work related to the front end, back end, database, and integration process. As a full-stack developer, you must be concerned with writing the server-side API and back-end programming languages, running the client-side JavaScript code, and accessing databases and version control systems. A full-stack developer brings value to the team and organization since they have a diverse skill set and the capacity to work on a project independently, which lowers operating expenses.
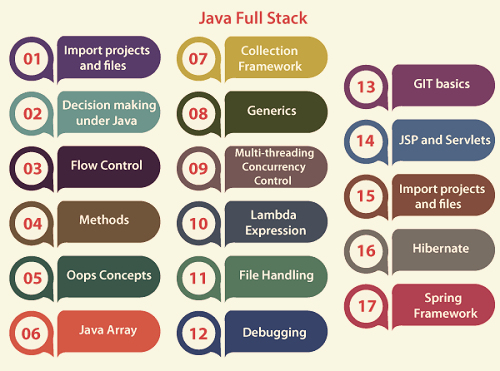
To become a Java full-stack developer, a developer should have the following skills:
1. Import projects and files
The developer should understand how to install IDEs such as IntelliJ and Eclipse on the system, how to run the hello world program in IntelliJ, how to import project files, and how to import Eclipse (Java IDE) projects in IntelliJ. These are fundamental concepts that both experienced and inexperienced candidates should understand.
2. Decision-making under Java
The developer should be familiar with decision-making statements and the operators used to make decisions. Operators such as arithmetic, assignment, and ternary operators are used for decision-making. If, else, if, and else statements are helpful in decision-making. As a result, a developer should be familiar with all of the decision-making statements and operators.
3. Flow Control
The developer should be familiar with control flow statements and looping statements after reaching a decision, including while, do-while, for, switch case, break, continue, modulo operator, and nested loops. If a developer is unfamiliar with flow control, they cannot write code. First and foremost, if you want to work in a full-stack position, you should learn all the fundamentals of Java. Otherwise, you won't be able to write complex Java code for a business.
4. Methods
Both parameterized and non-parameterized methods with the return type should be familiar to the developer. The two most crucial polymorphism ideas, method overloading and method overriding are essential for creating Java desktop and web applications. Since Java is an object-oriented programming language, you should have a solid understanding of how processes work. Additionally, the developer must be well-versed in code blocks, indentation, and statements.
5. Oops Concepts
Because Java is entirely built around objects, methods, and classes, the developer must be familiar with all Oops principles. Before moving on to more advanced Java, you should be familiar with basic Java concepts such as classes, getters, setters, constructors, inheritance, composition, encapsulation, abstraction, objects, and polymorphism.
6. Core Concepts
Every language that uses objects as its building pieces is called object-oriented. Interfaces, Multiple inheritances utilizing an interface, an anonymous object, an anonymous inner class, user input, and static elements come under the core concepts.
A static inner class, the final keyword, packages, scope, an access modifier, and an abstract class Exception handling, several try-catch blocks, a block after the Finally clause, throws and more throws, User-defined exceptions, checked and unchecked exceptions, also take part in the core concepts.
Enumerator enums, and strings (including literal strings, objects made of strings, methods that manipulate strings, and string formatting), among others. Learn all of the above ideas thoroughly if you are unfamiliar with the fundamentals of Java.
7. Java Array
Although Java array can be incorporated into core concepts, it would be best to keep it separate. Because we may utilize an array for numerous operations, it is one of the significant concepts in and of itself. We need to understand how to define and initialize arrays, iterate over them using a for loop, access and remove variables from them, call by value and call by reference methods, and many other things.
8. Collection Framework
The core of Java includes all of the ideas that were just covered. Once familiar with the fundamental Java principles, you are prepared to delve into advanced Java and learn its concepts. The collection framework is the first and most significant, which offers several helpful classes like Iterators, ArrayList, Stack, LinkedList, Comparable interface, etc. All of these classes and interfaces should be familiar to us in terms of both their methods and implementation.
Additionally, the collection framework has several advanced classes like Set, Queue, Dequeue, Map, and TreeMap.
These classes are highly beneficial for maintaining data when creating complex logic and procedures. The developer should also be familiar with various Set types, comparative custom sorting, equal and hashcode methods, and searching within maps in addition to these classes.
9. Generics
A Java developer should know type parameters, generic methods, bounded type comparable interfaces, wildcards, and generics. For writing complex and protracted codes, these are crucial.
10. Multi-threading Concurrency Control
The most crucial concepts in advanced Java are concurrency control and multi-threading concurrency. The developer should be familiar with multi-threading, extending the thread class or implementing the runnable interface, and other related concepts. Static synchronization, the volatile keyword, wait and notify, interrupt, join, Deadlock, CountDownLatch, Blocking Queue, Reentrant Lock, and thread pools are some examples of synchronization under concurrency management. These ideas are necessary for creating logic or creating multi-threading Java programs.
11. Lambda Expression
To represent one method interface using an expression, Lambda Expression was added in Java SE 8. Predicates, lambda expressions with methods, and iterators are other concepts that the developer should be familiar.
The Lambda expression:
- Excellent for the library's collection.
- Assists in iterating over data from the collection.
- Helps to extract data from the group by filtering it.
- Provides for the implementation of a functional interface.
- Saves a lot of code.
12. File Handling
Every programming language has to understand the concept of file handling. To deal with files, the developer needs to be familiar with all of the read- and write-related principles. The developer should have familiarity with writing to files, creating directories, creating files on the disc, and working with data streams. Scanner vs Buffered reader when reading files, Reading files with a buffer while using a scanner, deleting files, Try using resources and object serialization.
All the above ideas must be understood to create programs that work with files. Therefore, you should be familiar with these ideas to work as a full-stack Java developer.
13. Debugging
Finding defects and errors in the code involves a process called debugging. Enhancing code quality through debugging is crucial. Debugging skills should be possessed by developers. Debugging is beneficial for both fixing bugs in the code and comprehending how programs work. The developer must understand how variables are updated at runtime, what a watch is, and how to use a watch on variables.
14. Basics of GIT
One of the most popular current version control systems is GIT. Linus Torvalds created it as an open-source project in 2005. Java programmers use GIT to maintain any collection of projects under version control.
The following knowledge is required for the developer to become a Java full-stack developer:
- Attributes of the GIT.
- GIT configuration.
- Clone the GIT project and import it into Eclipse.
- Clone a GIT project directly from Eclipse.
15. Servlets and JSP
Knowledge of the following ideas is required for developers:
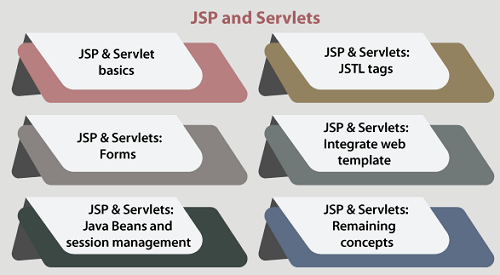
- Basics of JSP & Servlets
It covers topics like the Servlets life cycle, the JSP expressions element, the JSP scriptlets element, the JSP declarations element, the JSP comment element, the JSP directive element, the deployment descriptor, and the annotations element, Using the deployment descriptor's JSP settings, Reading URL parameters, including files in JSP pages, importing classes, forwarding and redirecting within JSP, an overview of MVC, fundamental redirection using servlets, and MVC-based applications. - JSP & Servlets: Forms
The developer should be familiar with form elements, JSP and Servlet forms, form validation, and other related topics. Java form design requires the understanding of these ideas. For security purposes, every application must have an authentication page, such as a login or register page. As a result, designing or implementing an application would be impossible without a form. - Java Beans and Session management in JSP and Servlets
The creation of an application requires both Java Beans and session management. A bean is a reusable software component that combines multiple objects into one that can be accessed from various locations. Session management is crucial for Java web applications to maintain user sessions using numerous methods like Cookies and HttpSession, etc.
Request scope, page & application, session, bean scope, and online forms for beans, Meeting under the JSP, cookie read and write operations, user logout, and organizing software are essential concepts. To become a Java full stack developer, you must understand fixing redirect and forward links, managing sessions without cookies, and servlet filters. - JSTL tags for JSP and Servlets
The five different JSTL tags are called JSTL core tags, JSTL functions tags, JSTL XML tags, JSTL formatting tags, and JSTL custom tags.
The terms "JSTL setup," "JSTL set and remove," "Reading from Bean using expression language," "JSTL choose and when," "JSTL for loop," "JSTL forEach," "JSTL for Tokens," "JSTL import and param," "JSTL URL and redirect," and "JSTL catch" are examples of JSTL core tags.
The JSTL length function, JSTL trim, and escapeXml functions, JSTL more function, JSTL even more function, and JSTL split and join operations are all examples of JSTL functions tags. The JSTL XML and Parse and Out tag, the JSTL XML ForEach and if tag, and the JSTL XML Choose, When, and otherwise are all examples of JSTL XML tags. The formatting date and the number are supported by JSTL formatting tags (Document). A tag that the developer creates is a JSTL custom tag. - Integrate web templates using JSP and Servlets
It is an additional essential concept in JSP and Servlet. We frequently need to embed web templates into our program. To integrate web templates, we need to understand how to extract the header and footer from the template, integrate the template with the project, and use JSTL. - The remaining concepts for JSP and Servlets
A developer should also be familiar with databases, using workbenches, setting up JNDI, listing data on webpages, using include directives, and adding, updating, deleting, and fetching records from databases, as well as providing JSTL support and image/file upload capabilities.
16. Hibernate
Another Java concept is known as Hibernate. An effective developer should be knowledgeable of Hibernate's implementation and architecture.
The developer ought to know MySQL and SQL Workbench. The developer should be knowledgeable about session factory and sessions in hibernate. Embedding Entity class, Hibernate in Action, HQL (Hibernate Query Language), HQL where clause and CRUD (Retrieving Record from Database, Updating a Record in Database, and Deleting Record from Database) are acronyms for these actions. Updating the record with HQL, Using HQL to delete a record, integrating hibernate with JSP and Servlet, configuring hibernate, Hibernate entity class with JSP, and Servlet included in the Hibernate concept.
Hibernate in action with JSP and Servlet, display image files, enhance the page's visual appeal, add an update information form, implement an update information feature, use hibernate to update specific column data, add a view image action, implement a view image page, and add a delete image action are essential concepts.
17. Spring Framework
For Java, the Spring framework is primarily employed. Modern Java-based enterprise applications may be programmed and configured using its complete programming and configuration paradigm. We should be familiar with the necessary tools to work with the Spring framework, Dependency injection, autowire situations, and inversion of control annotation of qualifier, Constructor injection, Spring Bean, Springtime IntelliJ project, Adding support for SpringMVC in Eclipse simple project for a dynamic web, model in a web project, basic setup for Spring MVC, a simple form with ModelAndView, Spring MVC, and foreach on the data Input and radio buttons, radio-buttons with backgrounds, a drop-down list, a text box, a checkbox, and other Springform components Eclipse's STS 3 support has been added. Add an outside source, a stylesheet, validation of forms JDBC is used for database connectivity (XML configuration and Annotation), Spring MVC's handling of exceptions, Web services with a restful API, hibernate database connectivity, JAX-RS, Spring MVC with a background in restful web services, and spring boot for constructing microservices with a restful API and a dependable microservice with database connectivity.
To become a full-stack Java developer (A person who can create both the front end and back end of an application is a full-stack developer), you must master the above topics. You will undoubtedly pass the Java full-stack developer interview process if you are familiar with these concepts.