Convert JSON File to String in Java
Before understanding the conversion of JSON file to string, one must know about JSON.
What is JSON?
JSON stands for JavaScript Object Notation. It is an open standard format lightweight, text-based, and specifically designed for human-readable data interchange.
JSON is a data format and it is independent of language. Douglas Crockford first specified JSON in the early 2000s. 2013 saw the ECMA-404 standardization of JSON, and 2017 saw the publication of RCF 8259.
It supports nearly every language, framework, and library. JSON is an open standard for data exchange on the internet. It recognizes data structures such as objects and arrays. As a result, it's easy to write and read data from JSON.
Key-value pairs are used to represent data in JSON, and curly braces are used to hold objects with a colon following each name.
Use commas to separate key-value pairs. Each value in an array is separated by a comma and stored in square brackets.
Converting a JSON file into a string
JSON files are easily converted to strings in Java. Reading the file's bytes of data can convert a JSON file into a string.
The nio (non-blocking I/O) package, a collection of Java programming language APIs that provide features for intensive I/O operations, is used to convert JSON files to strings.
Assume we have a Sample.json file in the system that contains the data below:
{
"Name" : "Virat",
"Mobile" : 89346724,
"Boolean" : true,
"Pets" : ["Lion", "Dog"],
"Address" : {
"Permanent address" : "INDIA - DELHI",
"current Address" : "INDIA - MUMBAI"
}
}
The following procedures are used to transform JSON data into a string:
- Bring in all necessary classes, including Files, Paths, and Scanner.
- Obtain the user's input for the name and location.
- Create the convertFileIntoString().
- The get() method of the Paths class is used to retrieve the file data in the convertFileIntoString() method.
- We read the bytes data from the JSON files as a string using the readAllBytes() method of the Files class.
- We return to the main() method, a variable that contains the output of the get() and readAllBytes() methods.
- We merely print the convertFileIntoString() method's returned result in the main() method.
Using the procedures described above, we can now convert or access the data in the Sample.json file:
Filename: ReadFileAsString.java
// import the necessary packages and classes
// To read the JSON file's bytes of data, we use classes from the nio package.
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.Scanner;
// make a class called ReadFileAsString to transform a JSON file into a string.
public class ReadFileAsString {
// start the main() method
public static void main(String[] args) throws Exception { //handle exception
// Declare the variables fileName, location, and file so that the user entered filename, location, and file data are each stored as a string, respectively.
String fileName, file, location;
// Scanner class object creation
Scanner sc = new Scanner(System.in) ;
System.out.print("Enter the name of the file which you want to convert or access as string: ");
// take input from the user and initialize filName variable
fileName = sc.nextLine();
System.out.print("Enter the location of the "+fileName+" file: ") ;
//initialize the location variable after receiving input from the user.
location = sc.nextLine();
// close the scanner class obj
sc.close();
// Make use of the convertFileIntoString() method to receive the file's data as a string. The data is saved into a file variable.
file = convertFileIntoString(location+"\\"+fileName);
// print the outcome, JSON data presented as a string.
System.out.println(file);
}
// To transform a JSON file into a string, create the convertFileIntoString() method.
public static String convertFileIntoString(String file)throws Exception
{
// Declare a variable in which the JSON data will be kept as a string and returned to the main() method.
String result;
// create the result variable.
// To retrieve the file data, we employ the Paths get() method.
// To read byte data from the files, we use the readAllBytes() Files method.
result = new String(Files.readAllBytes(Paths.get(file)));
// return the result to the main() method
return result;
}
}
Output:
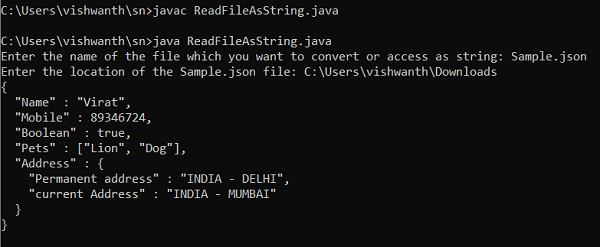
Note: In the code above, the JSON file is converted to a string and stored in a variable of the same type. Therefore, we can use the resultant variable to perform all string operations.