Reverse a String in Java
Reversing a string means that if we have a string called “what is your name”, the reversed format is “eman ruoy si tahw”. Reversing a string involves totally flipping the sequence of the characters, or in other words, reading a text backwards.
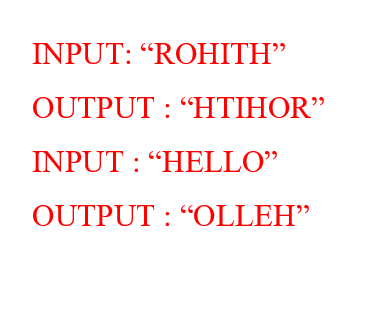
Program for string reverse
//stringReverse.java — file name
import java.io.*;
import java.util.Scanner;
class rev {
public static void main (String[] args) {
String s= "what is your name", sb="";
char c;
System.out.print("Original string: ");
System.out.println("what is your name"); //sample string
for (int i=0; i<s.length(); i++)
{
c= s.charAt(i);
sb= c+sb;
}
System.out.print("Reversed word: "+ sb);
}
}
Output

Explanation
In the above program, “what is your name” is the input string. Using for loop to iterate through the string, traverse through each character, and add it to the output string variable sb.
Different ways of reversing a string are as follow
- Using StringBuilder
- Using StringBuffer
- By Reverse Iteration
- String to Bytes
- String to a character array.
Generally, the String class doesn’t contain reverse() methods. Other techniques are used to reverse a string.
1)By StringBuilder
StringBuilder is a Java class used to construct a mutable, or changeable, sequence of characters. The Java Strings class offers an immutable string of characters; similarly, to StringBuffer, the StringBuilder class offers an alternative.
Program to reverse a string using StringBuilder
import java. lang.*;
import java.io.*;
import java.util.*;
class reversestr {
public static void main(String[] args)
{
String s = "welcome to the string reverse program";
StringBuilder sb = new StringBuilder();
sb.append(s);`
sb.reverse();
System.out.println(sb);
} //main
}//Main
Output

2)StringBuffer
The string class does not have a reverse method inbuilt. We have to use StringBuffer to use the reverse method. A string buffer always contains a specific character sequence, but by calling specific methods, the length and content of the sequence can be altered. The use of string buffers by many threads is secure.
Example 1. Program to reverse a string using StringBuffer
public class rev {
public static String reverseString(String str){
StringBuilder sb=new StringBuilder(str);
sb.reverse();
return sb.toString();
}
}
public class TestRev {
public static void main(String[] args) {
System.out.println(rev.reverseString("welcome to the string reverse"));
}
}
Output

Example 2. Program to reverse a string using StringBuffer
//second example
import java.lang.*;
import java.io.*;
import java.util.*;
public class Test {
public static void main(String[] args)
{
String s = "hello";
StringBuffer sb = new StringBuffer(s);
sb.reverse();
System.out.println(sb);
}
}
Output

3)By Reverse Iteration
Given a string to reverse. Create an empty string variable. Use a for loop to get the characters of the string you wish to reverse in reverse order. Within the for loop, append each character to the name you've given as an empty string. Print the outcome.
Program to reverse a string using Iteration
//reverseString.java — file name
import java.io.*;
import java.util.*;
class Main {
public static void main (String[] args) {
Scanner sc = new Scanner (System.in);
System.out.println("Enter the string to be reversed:");
String s = sc.nextLine();
char[] a = s.toCharArray();
String reverse = "";
for(int i = s.length() - 1; i >= 0; i--)
{
reverse = reverse + s.charAt(i);
}
System.out.println("The reversed string is:");
System.out.print(reverse);
}
}
Output
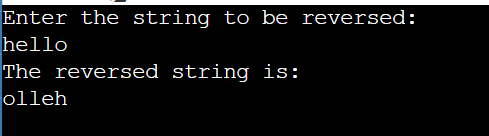
4)Converting String into Bytes
Make a temporary byte[] that is the same size as the input string. Put the bytes into the temporary byte[] in reverse order. To store the result, create a new String object with byte[].
Program to reverse a string using Bytes function
import java. lang.*;
import java.io.*;
import java.util.*;
class Main {
public static void main(String[] args)
{
String s = "hi there";
byte[] sb = s.getBytes();
byte[] r = new byte[sb.length];
for (int i = 0; i < sb.length; i++)
{
r[i] = sb[sb.length - i - 1];
}
System.out.println("Original string is :"+s);
System.out.println("Reversed string is:");
System.out.println(new String(r));
}
}
Output
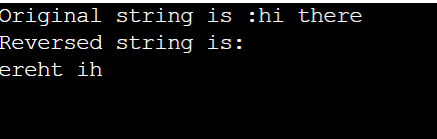
5)Converting String to a character array
This string is converted to a character array in Java using the toCharArray() function. It produces a newly formed character array with the same length as this string and the same contents as this string.
Program to reverse a string using toCharArray function
import java.lang.*;
import java.io.*;
import java.util.*;
class reverseString {
public static void main(String[] s)
{
String r = "hello everyone, good to see you";
char[] t = r.toCharArray();
for (int i = t.length - 1; i >= 0; i--)
System.out.print(t[i]);
}
}
Output
