Java Swing Time Picker
Prerequisites
In this tutorial, we will learn the time picker in java swing. Before learn time picker, we should learn about java swing.
Java Swing Introduction
The Java Foundation Classes include Java Swing. It is suitable for constructing light desktop apps because it is used to generate window-based applications.
Java Swing is based on an abstract windowing toolkit API that is completely implemented in Java.
Java Swing offers light and platform-agnostic components, making it ideal for designing and creating desktop-based applications (systems).
Differences between Java AWT and Java Swing
To create graphical user interfaces in Java, both Java Abstract Window Toolkit (AWT) and Java Swing are utilized.
Although, depending on what one wants to do, they have variances that make one more suitable than the other.
The following are some of the differences:
- The Java AWT framework contains a set of functions and processes for developing applications that can access functionality or data from the operating system, other programs, or even services. The Java AWT Application Programming Interface (API) is used to create Java graphical user interfaces. Java Swing, on the other hand, is made up of Java Foundation Classes and may be used to create standalone apps.
- Because they interface with the operating system, Java AWT components are large. Java Swing, on the other hand, provides more capability than Java AWT because its components are light and strong.
- When compared to Java AWT applications, Java Swing applications are faster and more efficient. The swing takes less time to execute than AWT.
- Because its components are platform-dependent, Java AWT is a platform-dependent API framework. Swing components, on the other hand, are independent of the platform. As a result, Swing is platform agnostic.
Swing Components for Desktop Applications that are Commonly used (GUI):
Swing is used to create window-based applications, as previously mentioned. The javax.swing package contains Swing components.
Java Swing Elements are critical elements for designing, developing, and deploying an application. For the Java programming language, the Swing Graphical User Interface widget toolkit is made up of these components.
Components are located under the palette in the NetBeans IDE. Java Swing components allow users to interact with the application.
We'll look at a few Swing components that are often utilized in desktop application development in this tutorial.
These include:
- JFrame
- JLabel
- JTextField
- JButton
Others Includes: JComboBox, JPanel, JCheckBox, JRadioButton, JList, JTable, JFileChose, ImageIcon, JTextArea.
JFrame
In the Java Swing Component hierarchy, JFrame is the very first component. It can be made in one of two ways:
- By constructing a Frame class object. That is association-based production.
For instance:
import javax.swing.JFrame;
public class Main {
public static void main(String[] args) {
JFrame sectionFrame = new JFrame();
sectionFrame.setSize(600, 600); // 600 width & 600 height
sectionFrame.setLayout(null); // no layout managers
sectionFrame.setVisible(true); // frame visible
}
}
- You can do this by extending the Frame class. That is class inheritance-based OOP construction.
For instance:
import javax.swing.JFrame;
public class sectionHomeWindow extends JFrame {
/**
* Creates new form sectionHomeWindow
*/
public sectionHomeWindow() {
}
}
JLabel
JLabel is a Swing Container User Interface component that displays readable text or an image. The text presented in the JLabel cannot be edited by the application user.
The text can, however, be changed by the application itself via action events. Both plain and HTML text can be shown using the JLabel component.
For example:
JLabel labelName = new JLabel("Name");
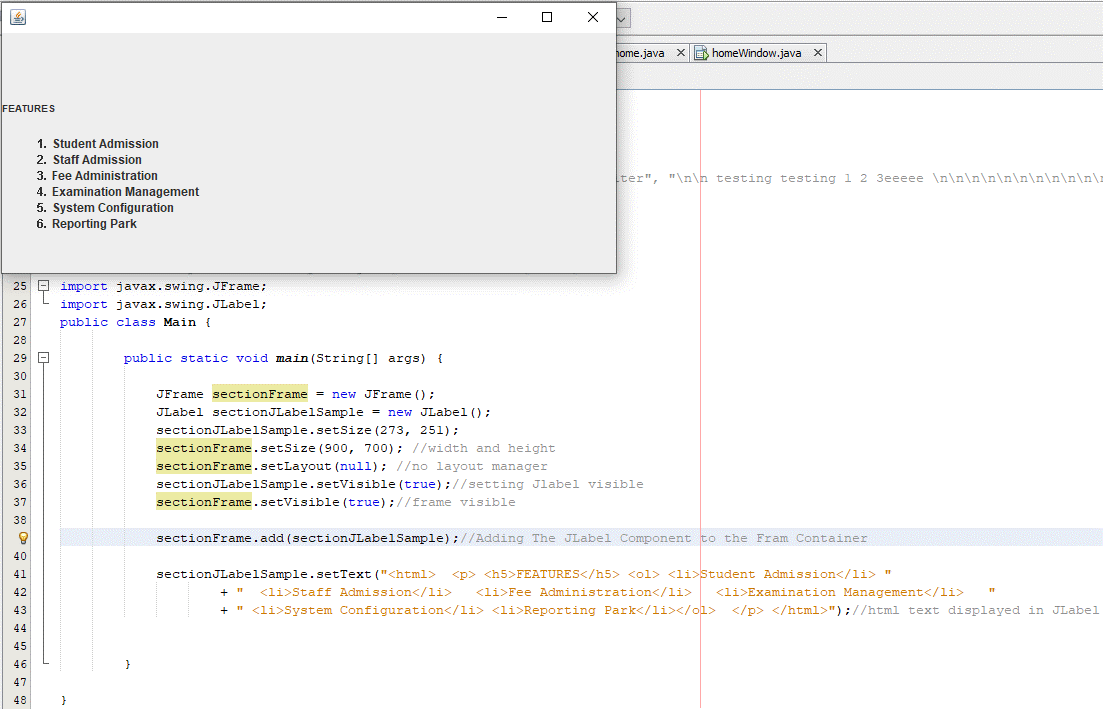
JTextField
JTextField is a swing element that lets users enter a single line of text. JTextField is a descendant of the javax.swing Library's JTextComponent class.
For example:
JTextField sectionTextField = new JTextField(20);
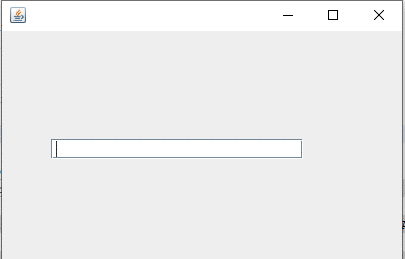
The enable property must be set to true for JTextField to be available and editable. JTextField can be initialized by giving an integer type parameter to the JTextField constructor.
The number of characters a user can type in the text field is not limited by the integer argument given in the constructor. It does, however, provide the text field box's width,i.e.the number of columns to be assigned to it.
JButton
Swing's platform independence is provided by JButton, one of the swing components. This component adds a click effect to the user interface of the program. Any of the class constructors can be used to implement it in an application.
By clicking or double-clicking on it, data from databases is retrieved and displayed on the UI, or user data is collected on the UI and stored/saved in a database.
In most applications, JButton has text or a picture that tells the user what the button does.
For example:
JButton submitButton = new JButton("Submit");
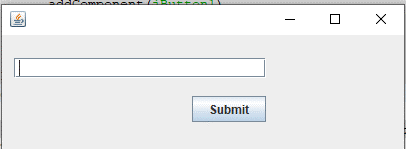
Use NetBeans to Create a Java Swing Desktop Application
NetBeans is an integrated development environment (IDE) for developing applications in a variety of languages. NetBeans supports a variety of programming languages, including Java.
Because it provides a user-friendly interface for planning and coding the UI, NetBeans is the ideal IDE for building desktop apps using swing. It has built-in swing libraries that auto-populate code.
Whn constructing a swing application in NetBeans, the built-in libraries give a palette area in the IDE. When creating an application, this makes it is easier to drag and drop components.
Let's create a simple Swing application that demonstrates the components discussed previously. It's a straightforward application that gathers personal information from customers.
1. Open the NetBeans IDE and go to File -> New Project.
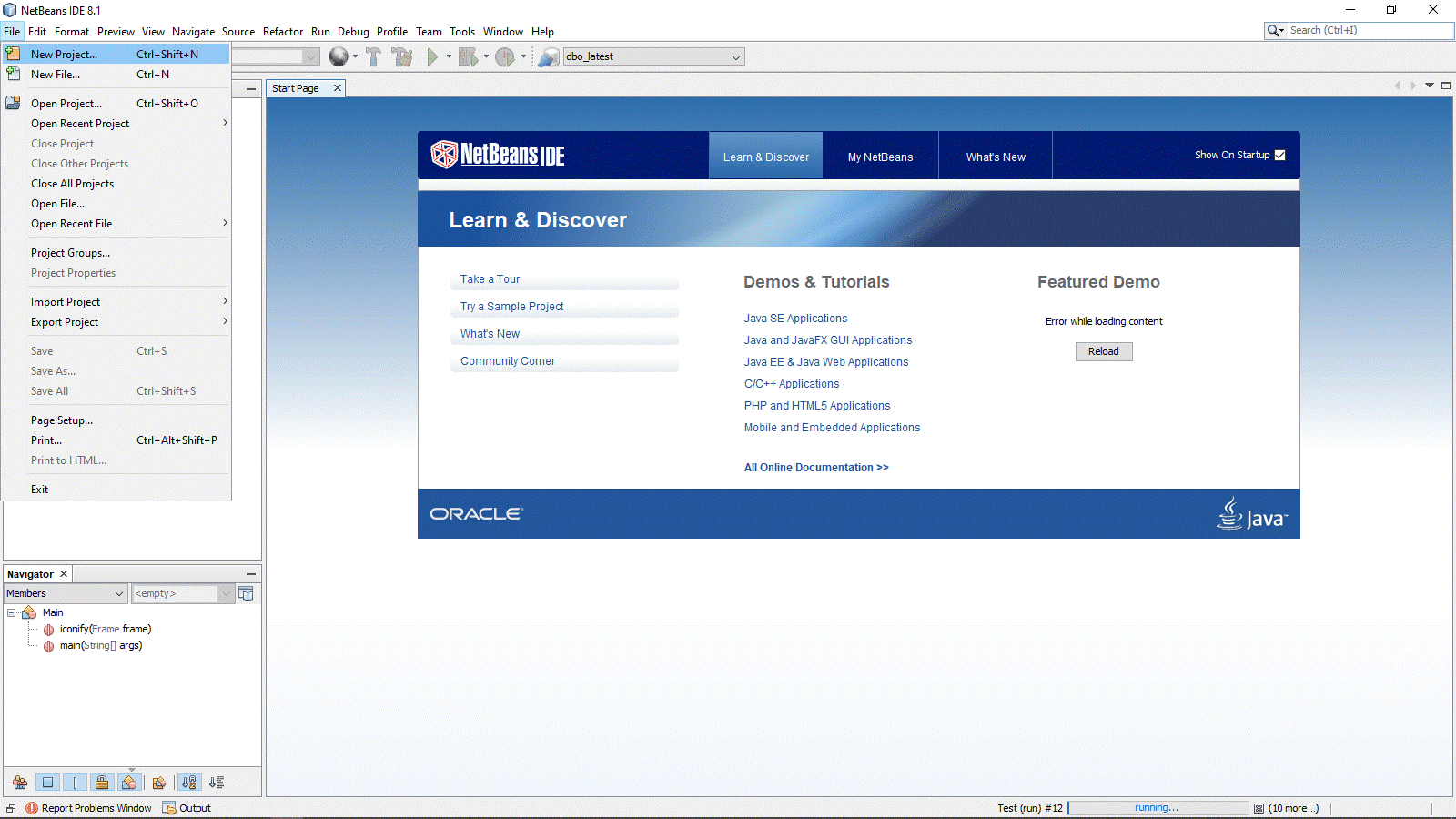
2. In the project window, select Java and Java Application, then click Next.
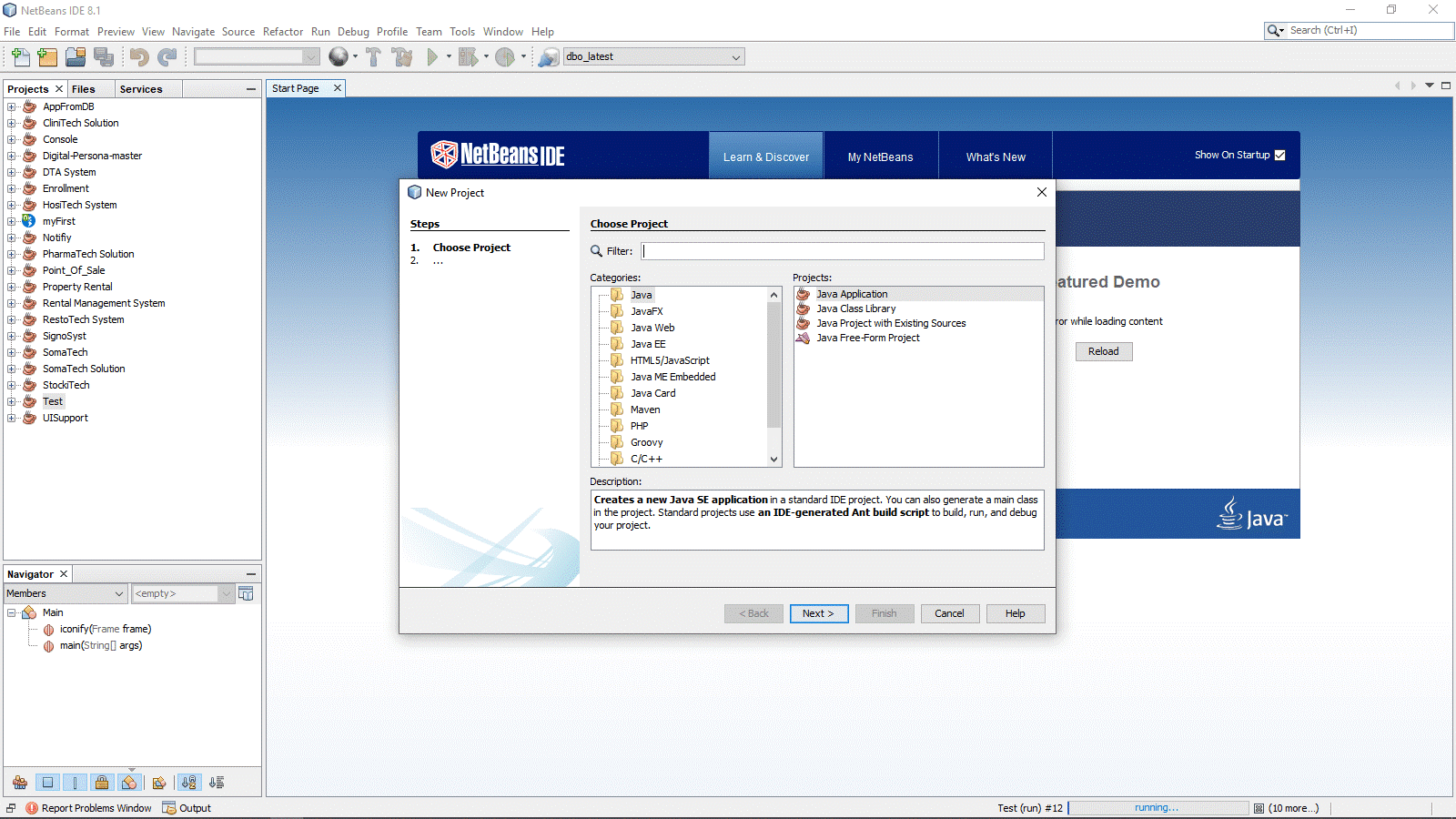
3. Leave sectionSampleSwingGUI as the project name and save it in the default directory. If you need to make the main class, check the Create Mainclass checked and the Use Dedicated Folder for Storing Libraries checkbox to make a dedicated library folder. Both checkboxes will be checked in our scenario. Wait for the project to finish before clicking Finish.
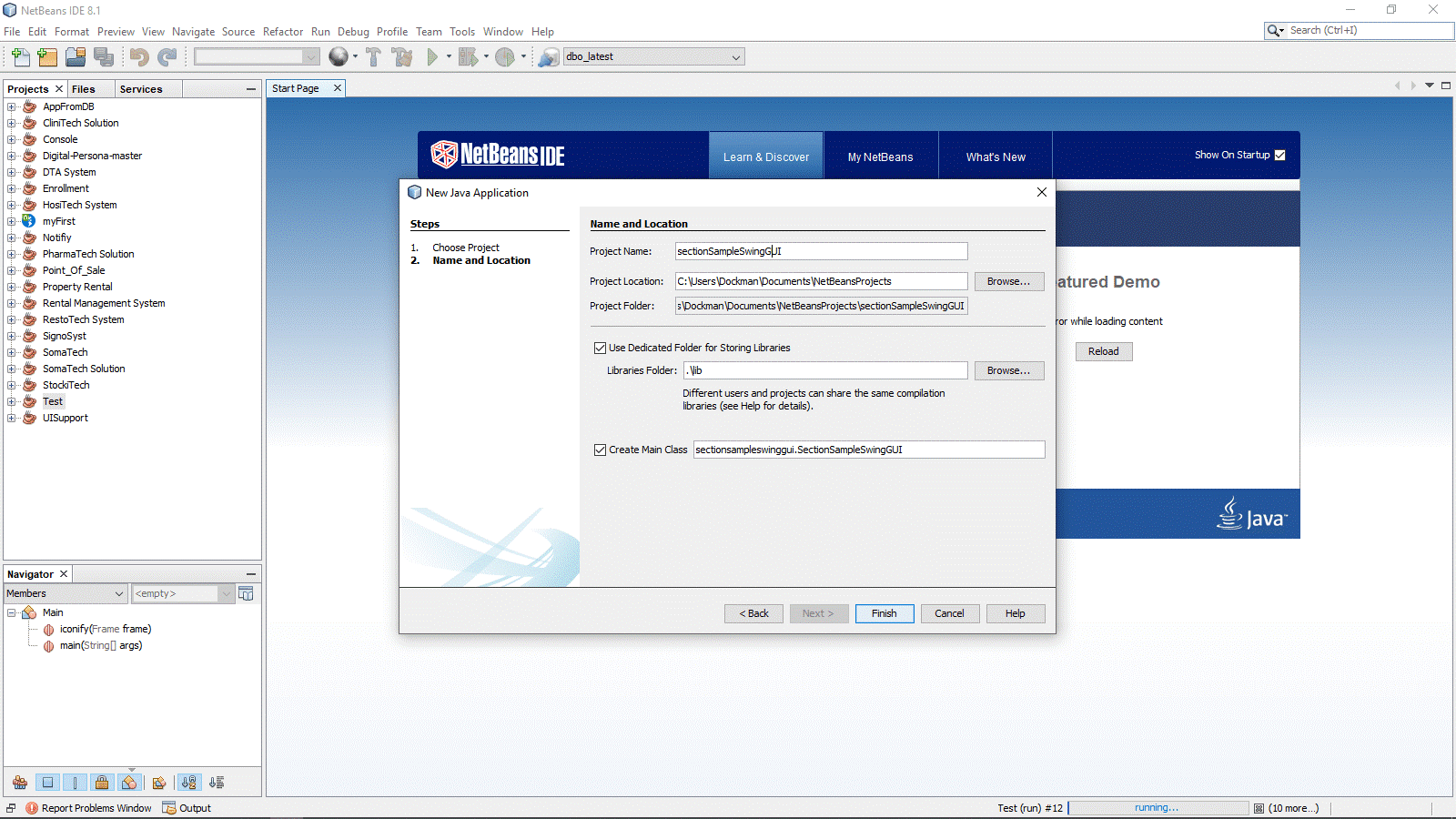
4. Select new from the project's right-click menu, then JFrame Form. This will be the main window as well as our main class.
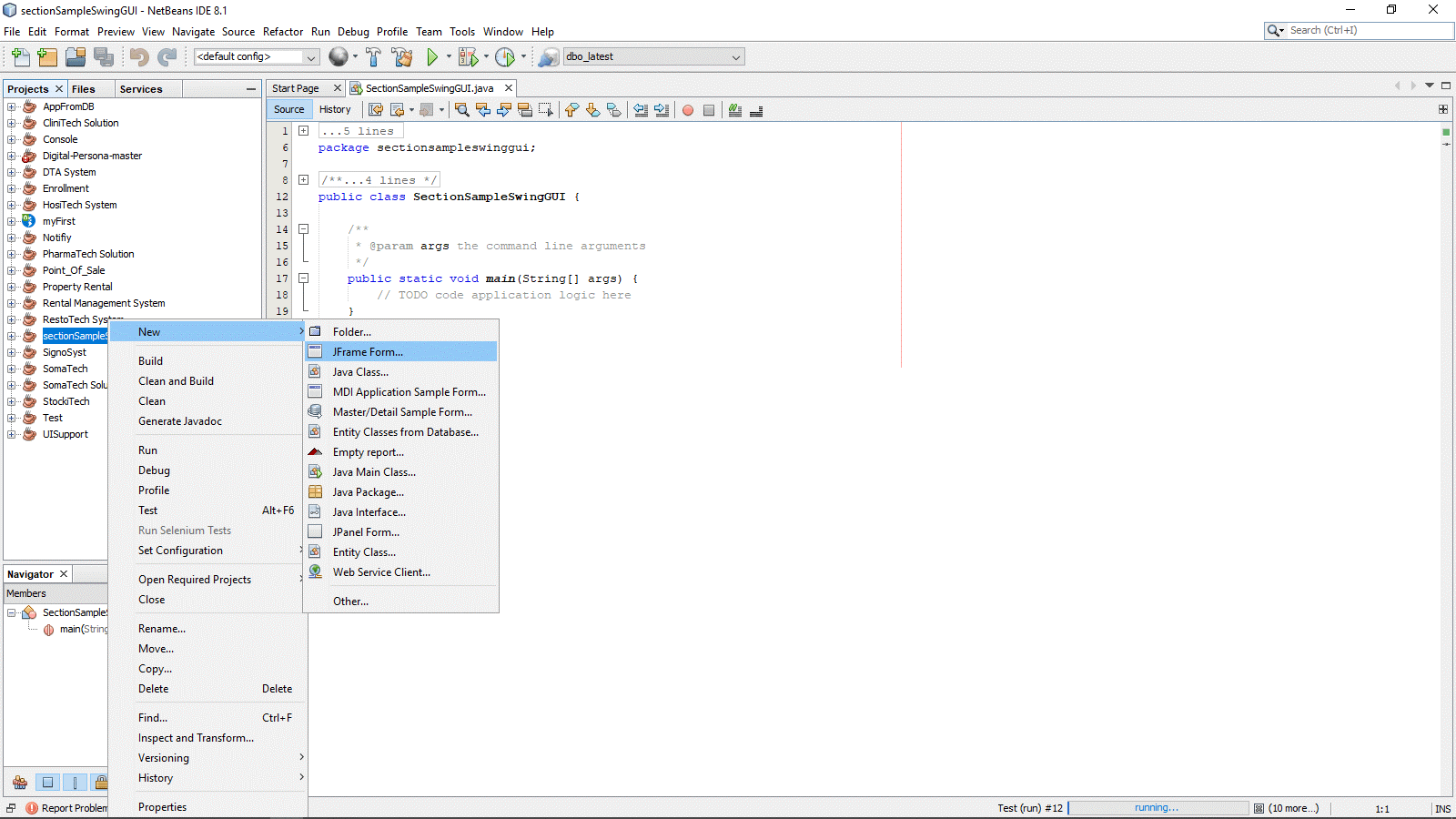
5. Next, give the class sectionHomeWindow a name. Select the package that was created when the project was created, i.e. sectionsampleswinggui, and then click Finish
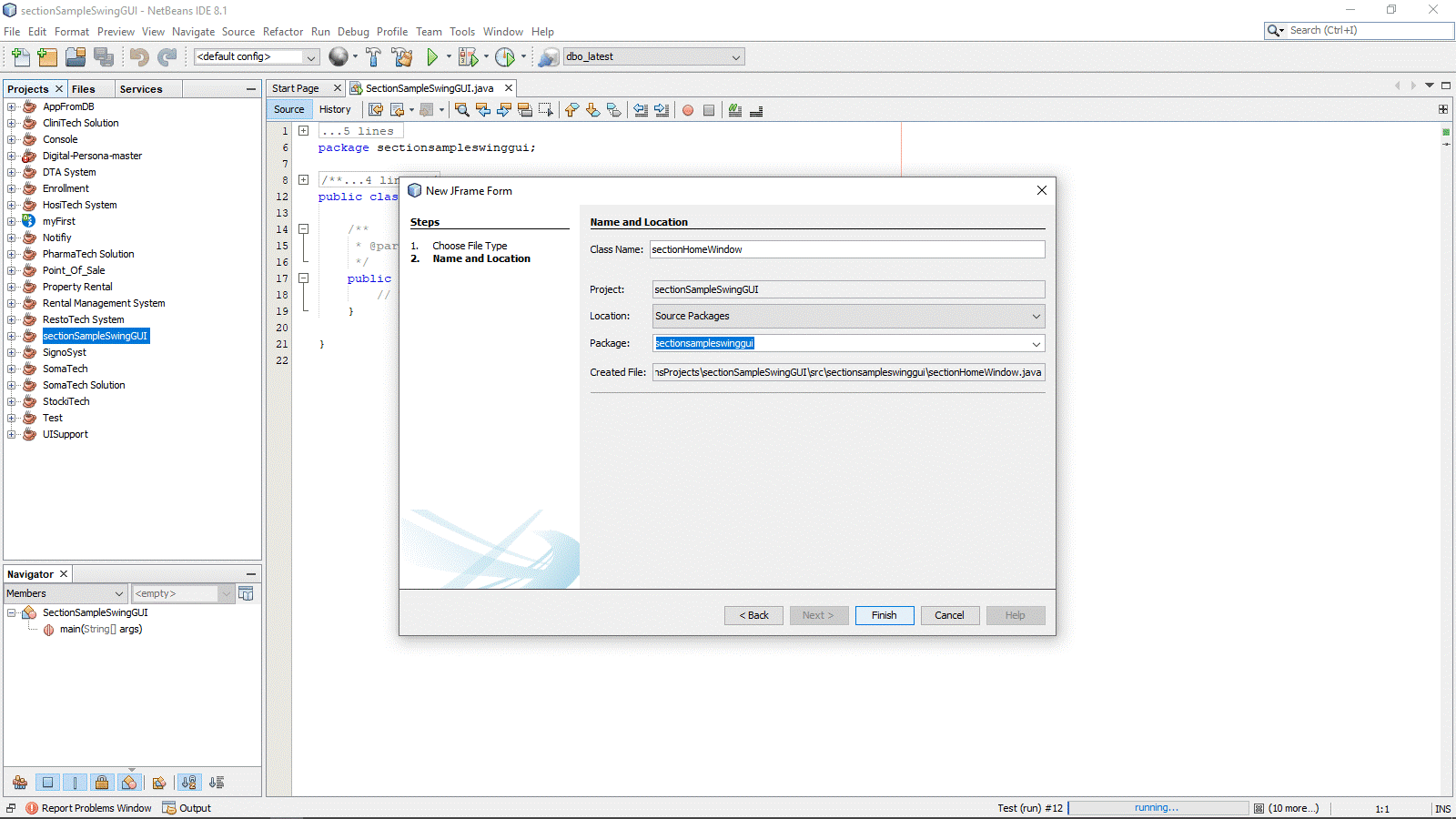
Our Window is now created. This is the main element that will house the other swing elements.
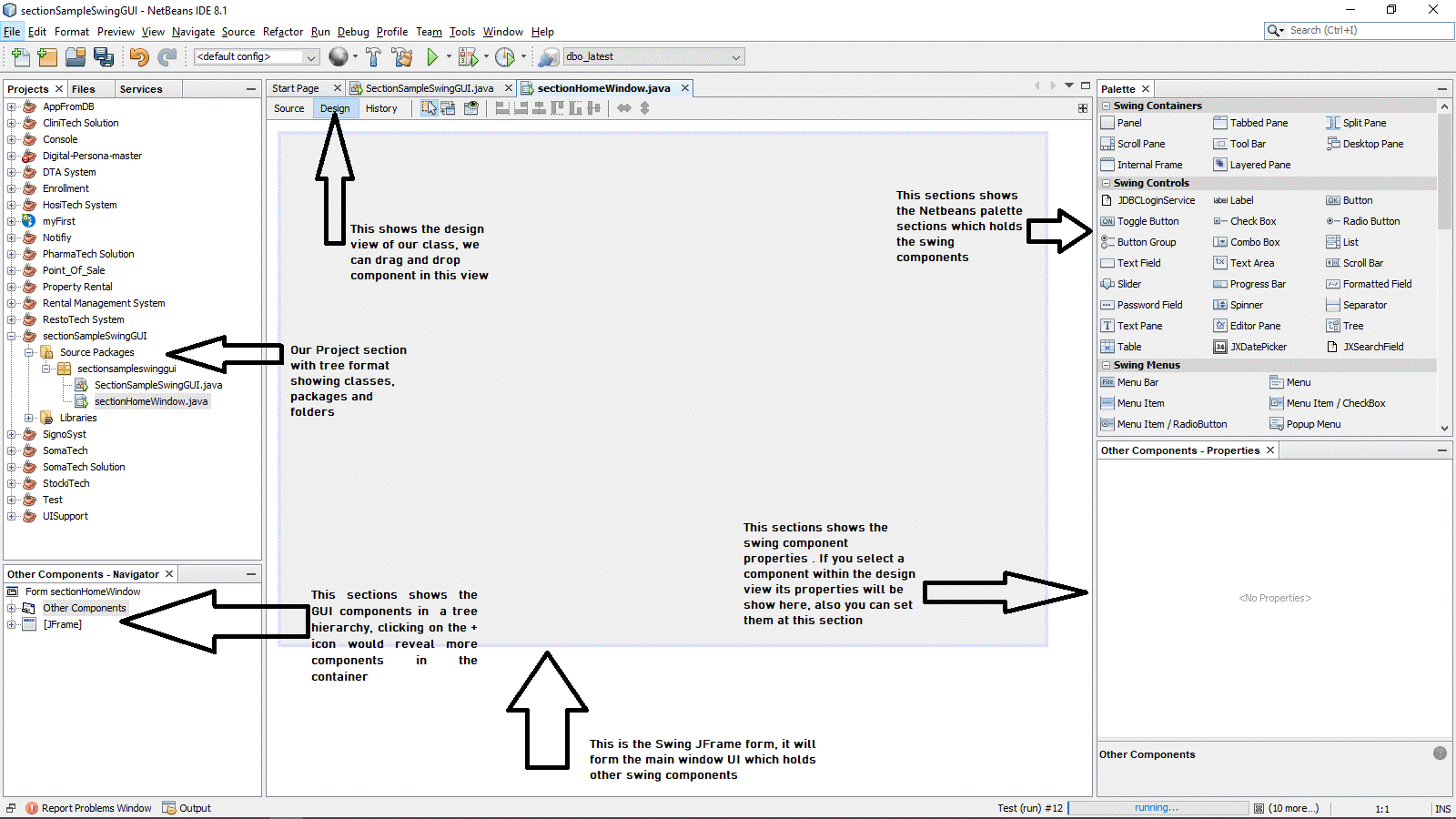
Java Swing Time Picker
1. We'll now drag and drop the elements covered earlier in this article into the JFrame. After that, we'll run our program to check how it looks. To execute the application, go to the IDE's Run button and select Run project from the menu bar.
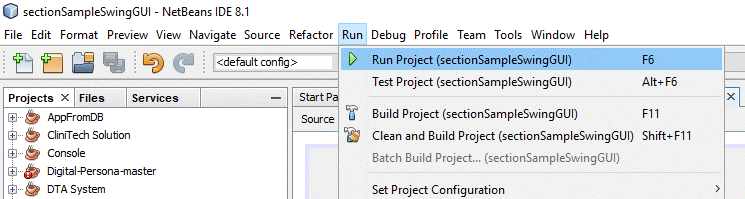
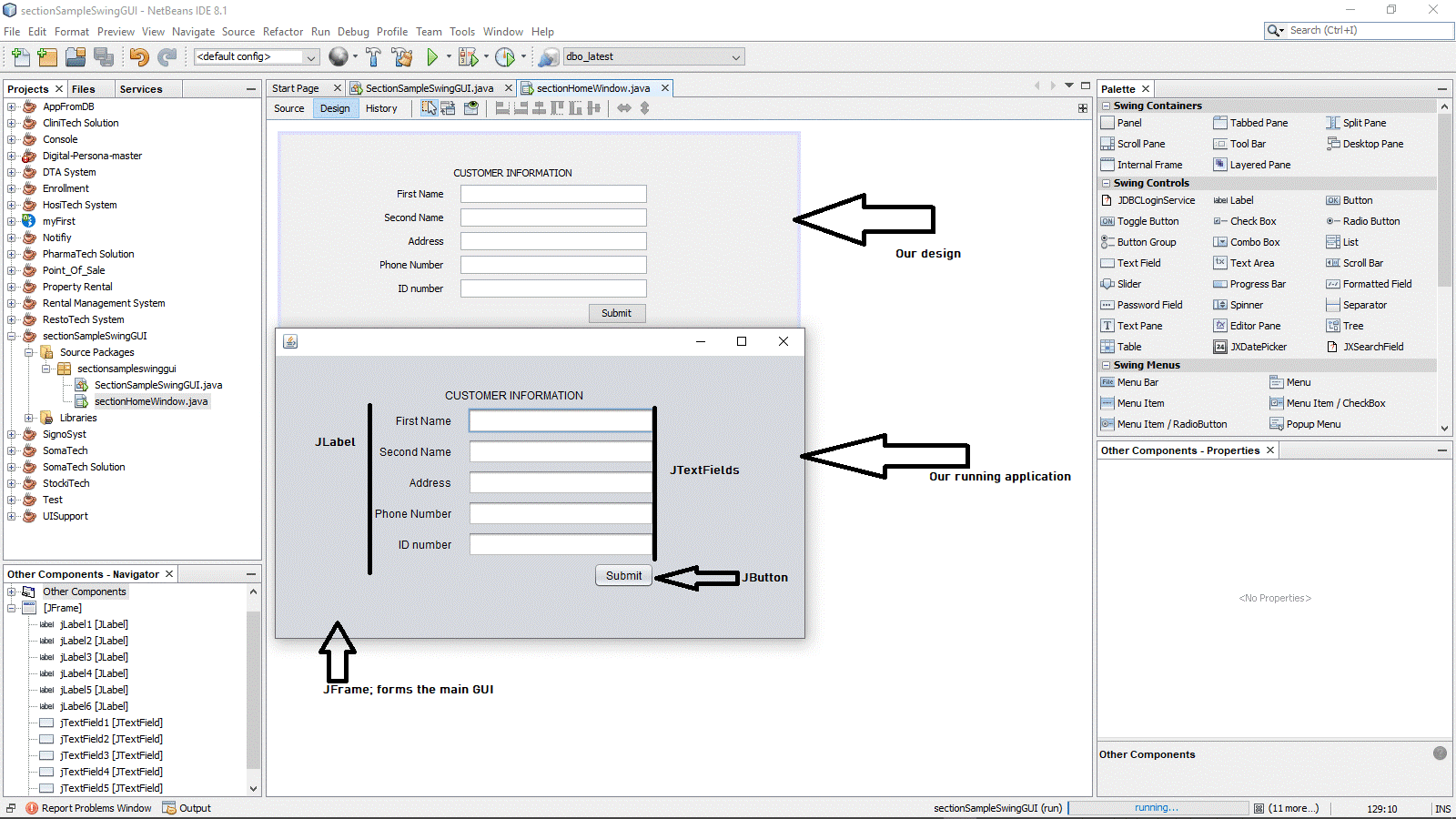
Conclusion
Java Swing is a fairly simple framework to work with. Its applications are substantially faster and more dependable since they are platform-independent and light.
The NetBeans IDE provides a simple and attractive GUI for dragging and dropping Swing components, making application building a breeze.
Swing Time Picker
Optional parameters allow you to modify the TimePicker. Language (locale), drop-down menu default times, typefaces and colours, display/menu/parsing formats,12 or24 hour clock, seconds or nanoseconds precision, and so on are just a few of the options.
The DatePicker and DateTimePicker components are also included in the library. Each of the three components is simple to use. (Each one may be created with just one line of code.)
Below are screenshots of the components as well as the example application.
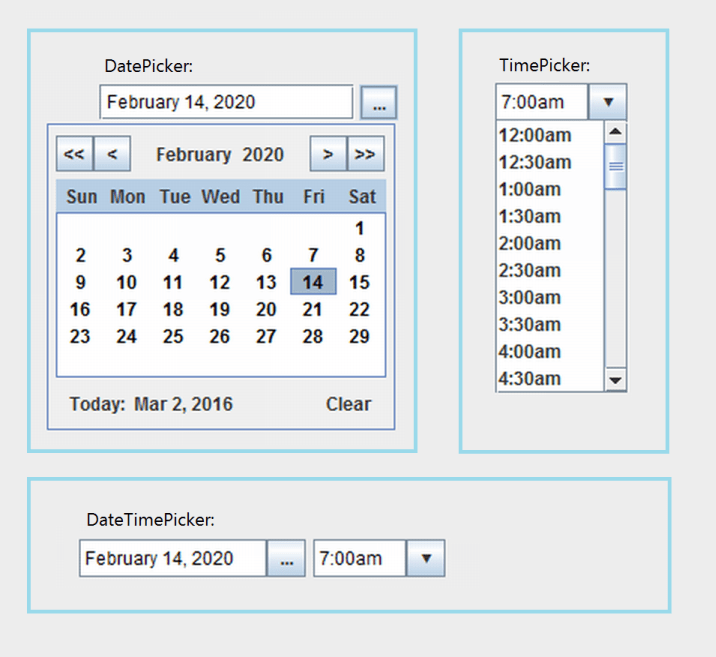
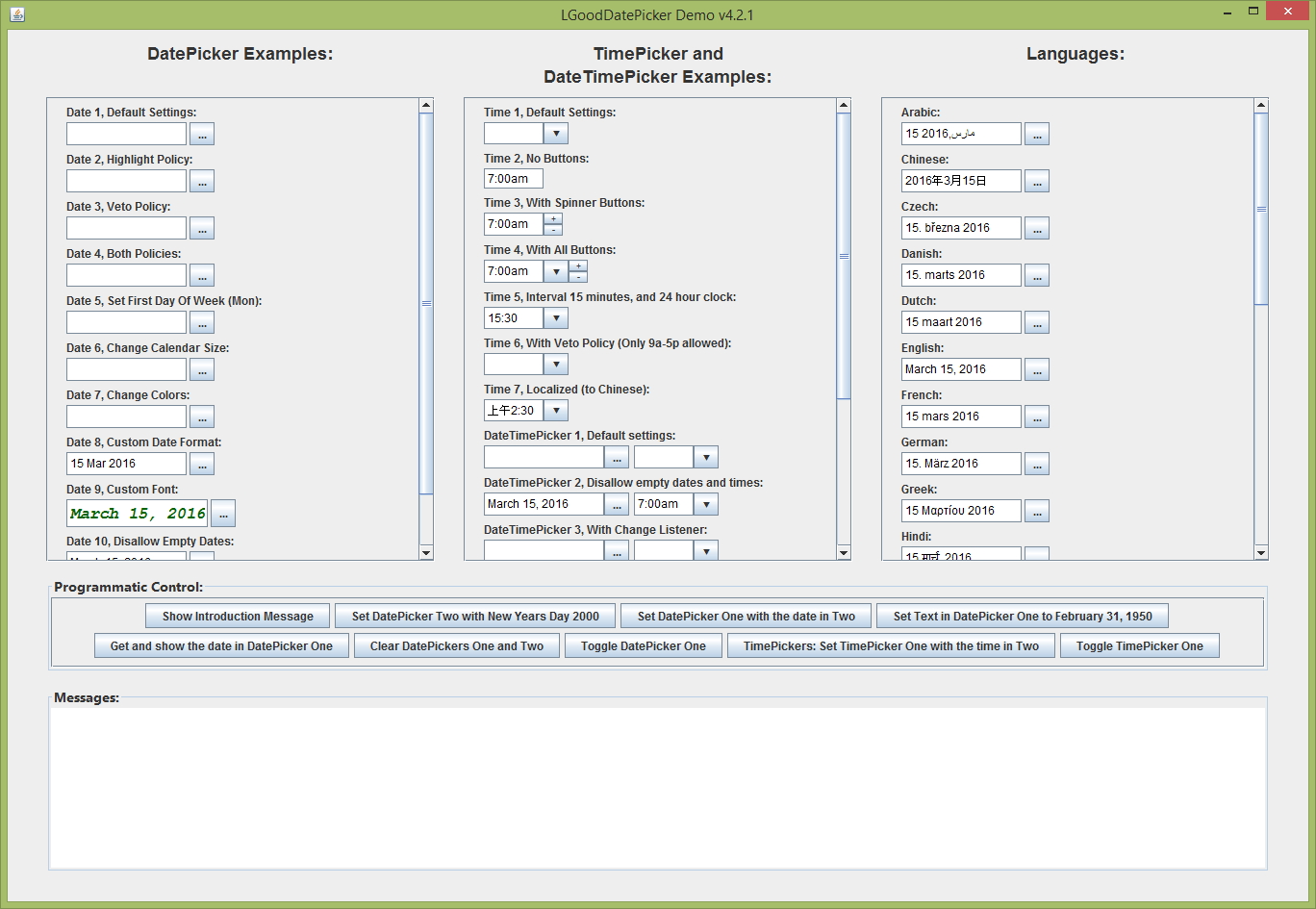