How to Split String by Comma in Java
strsplit() technique permits you to break a string given the explicit Java string delimiter. The Java string split property is frequently a space or a comma(,) that you want to break or divide the string with.
Syntax
public String split(String regex)
public String split(String regex, int limit)
Parameters
Regex: The standard articulation in Java split is applied to the text/string
Limit: A cutoff in Java string split is the greatest number of values in the exhibit. On the off chance that it is overlooked or zero, it will return every one of the strings matching a regex.
Exemption Thrown: PatternSyntaxException - on the off chance that the given ordinary articulation's punctuation is invalid.
The cutoff boundary can have three qualities:
- Limit> 0 - If this is the situation, at that point, the example will be applied to all things considered limit-multiple times, the subsequent cluster's length won't be more than n, and the subsequent exhibit's last section will contain all contribution past the last paired design.
- Limit < 0 - For this situation, the example will be applied whatever number of times as would be prudent, and the subsequent cluster can be of any size.
- Limit = 0 - For this situation, the example will be applied whatever number of times as would be prudent, the subsequent cluster can be of any size, and the following void strings will be disposed of.
On the off chance that you are not exceptionally acquainted with the customary articulation, then you can likewise utilize the StringTokenizer class, which can likewise part a comma-delimited String, yet StringTokenizer is an old class and not suggested. You ought to constantly attempt to utilize the split () capability from java.lang.String class, as any exhibition improvement, will probably occur on this technique than the StringTokenizer class. We should see two or three guides to part a comma-isolated String in Java.
Internal Implementation
public String[] split(String regex, int limit) {
/* fast path if the regex is a
(1)one-singe String and this character isn't one of the
RegEx's metacharacters ".$|()[{^?*+\\", or
(2)two-singe String and the principal roast is the oblique punctuation line and
the second isn't the ASCII digit or ASCII letter. */
char ch = 0;
if (((regex.value.len == 1 &&
".$|()[{^?*+\\".indexOf(ch = regex.charAt(0)) == -1) ||
(regex.length() == 2 &&
regex.charAt(0) == '\\' &&
(((ch = regex.charAt(1))-'0')|('9'-ch)) < 0 &&
((ch-'a')|('z'-ch)) < 0 &&
((ch-'A')|('Z'-ch)) < 0)) &&
(ch < Character.MIN_HIGH_SURROGATE ||
ch > Character.MAX_LOW_SURROGATE))
{
int off = 0;
int n = 0;
boolean l= limit > 0;
ArrayList<String> list = new ArrayList<>();
while ((n = indexOf(ch, off)) != -1) {
if (!l || list.size() < limit - 1) {
list.add(substring(off, n));
off = n + 1;
} else { // last one
//assert (list.size() == limit - 1);
list.add(substring(off, value.length));
off = value.length;
break;
}
}
// If no match was found, return this
if (off == 0)
return new String[]{this};
// Add remaining segment
if (!l || list.size() < limit)
list.add(substring(off, value.length));
// Construct result
int resultSize = list.size();
if (limit == 0)
while (resultSize > 0 && list.get(resultSize - 1).length() == 0)
resultSize--;
String[] result = new String[resultSize];
return list.subList(0, resultSize).toArray(result);
}
return Pattern.compile(regex).split(this, limit);
}
Split a String in Java with Delimiter
The model beneath describes the best technique to split a text in java using a delimiter:
Assume we have a string variable called strMain that has a few words separated by commas, such as Apple, Ball, Cat, Dog, and Egg. Assuming we require every single unique string, the best example is divided by the comma. As a result, we will obtain five distinct strings, as follows:
- Apple
- Ball
- Cat
- Dog
- Egg
Apply the string split in the java approach to the string to be partitioned and provide the separator as a parameter. The separator in this java split string by delimiter scenario is a comma (,), and the result of the java split string by comma activity is an exhibit split.
Example
class StrSplit{
public static void main(String []args){
String str = "Apple, Ball, Cat, Dog, Egg";
String[] Split = str.split(", ");
for (int i=0; i < Split.length; i++)
{
System.out.println(Split[i]);
}
}
}
Output
Apple
Ball
Cat
Dog
Egg
Three Java Methods for Splitting a Comma-Separated String:
- Index() and Substring() Method to Split Comma-Separated String in Java
- String List and Split Method to Split a Comma-Separated String in Java
- Array List and Split Method to Comma Separate a String Value in Java
The Java string class is plenty of valuable components that you may utilize to create simple projects that address complicated problem declarations. This instructional exercise will show cool ways of parting a string from a comma-isolated beginning stage or any division you could use in the string esteem set. Likewise, we will utilize the IndexOf(), a technique for our most special program and coordinate split () with java's dynamic cluster and string classes.
1) Index() and Substring() Method to Split Comma-Separated String in Java:
We can straightforwardly utilize simpler strategies to do a similar occupation since java has many saved capabilities in its string class.
indexof() Method in Java
It returns the place of the primary event of the predetermined worth in a client
determined string.
Parameter:
String | String Str = “I, am, a, Developer”; | Assume the beginning record is set to 0, the indexOf() look from “I”. |
From Index | An int esteem determining the record position to start the pursuit. | It will allow you to experiment with indexes (s). |
Char | An int esteem that contains a specific person. | discretionary |
Substring () Method in Java
It gives back a string that is a substring of this string. It starts at the specified position and ends with the person at the specified point.
Example
public class UseIndexofAndSubString {
public static void main(String[] args) {
// Specified string to search comma from and then split it in a substring
String java = "I'm, a, Developer!";
String comma = ","; // To search ',' from the first occurrence of the specified string
// Our starting index is 0 since we do not want to miss any occurrence in the
// given string value while the end index is also initialized
int start = 0, end;
// Now the indexOf will find the specified character which in this case is a
// comma (,)
// Note: The second parameter (start) determines that the method starts from the
// beginning, otherwise it will
// Basically, we will be able to locate the occurrence of (comma) from where we
// want it to be split
end = java.indexOf(comma, start);
// If the loop does not find (comma)
// We already mentioned the return type of indexOf in case if the given char
// never occurred, right? That is what the loop searches for!
while (end != -1) {
//// Substring will give us each occurrence until the loop is unable to find
//// comma
String split = java.substring(start, end);
System.out.println(split); // print each sub string from the given string
// Then add 1 to the end index of the first occurrence (search more until the
// condition is set false)
start = end + 1;
end = java.indexOf(comma, start);
}
// Since there is no comma after the last index of the string (language!), the
// loop could not find the end index; thus, it never printed the last word!
// We will get the last instance of the given string using substring and pass
// start position (Starts from L and ends at the !)
String split = java.substring(start);
// Brave, you got it all right!
System.out.println(split);
}
}
Output
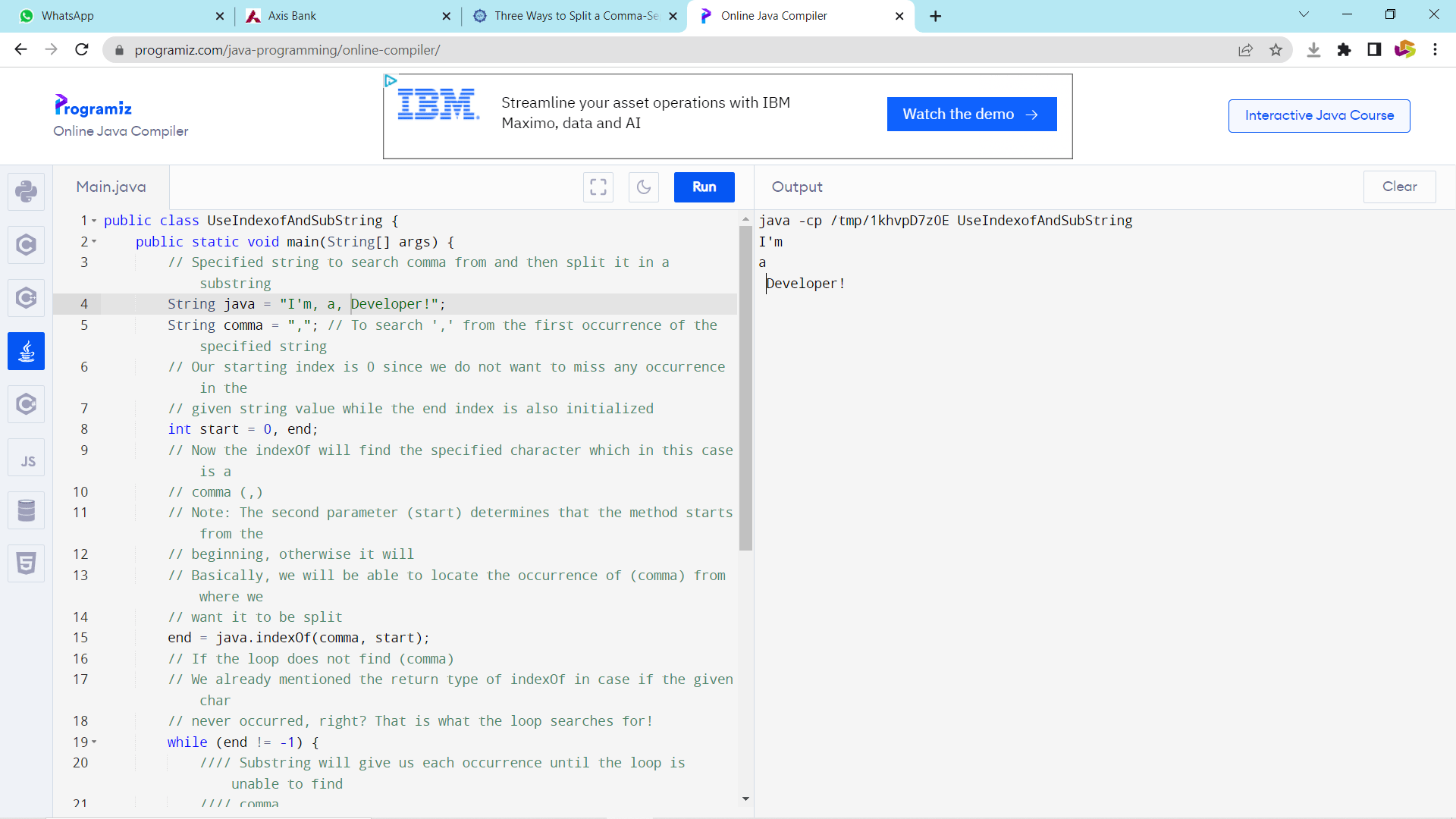
2) String List and Split Method to Split a Comma-Separated String in Java
At the point when we examined the significance of rudimentary level comprehension, we discussed clusters. The accompanying system is simple. You don't have to track down beginning and finishing positions, nor do you want to run some time circle and look at whether the return esteem is - 1. Java's string and array classes summarize everything in four lines of clean code. In the meantime, you really want to grasp two required strategies that will help you to route.
Arrays.aslist()) Function in Java
Syntax: List<String> ArrayString = Arrays.asList(IwillBeSplit.split(","));
Parameters:
- The class of the exhibit components' qualities.
- The client indicated an array of utilizations to store the rundown.
- It gives you a rundown perspective on the predetermined exhibit you characterized.
Split() Function in Java
It involves the two boundaries in the split technique that we, as clients determine as an ordinary articulation and a breaking point boundary of 0. Accordingly, it does exclude the followed void strings in the result set.
Parameter: Regular articulation that fills in as a separator.
Returns: A variety of strings created by isolating this line of the regex articulation.
Example
import java.util.Arrays;
import java.util.List;
public class UseStingListAndSplitMthd {
public static void main(String[] args) {
String str = "There,are,three,seasons,!&#^*,in,a,year";
// List is an interface in Java
List<String> ArraySting = Arrays.asList(str.split(","));
// Using for each loop
ArraySting.forEach(System.out::println);
}
}
Output
There
are
three
seasons
!&#^*
in
a
year
3) Array List and Split Method to Comma Separate a String Value in Java:
The accompanying code block shouldn't confound you. Since, you are now acquainted with the Arrays.asList()); and the split(). In any case, the one thing different here is the way to deal with the arrangement, which is utilizing ArrayList<String> rather than just List<String>. It isn't so difficult. The rundown is a connection point, while an ArrayList is a Java assortment class. The previous gives a static exhibit, while the last option produces a unique cluster to store the components.
Example
import java.util.ArrayList;
import java.util.Arrays;
public class ArrayListSplitMethod {
public static void main(String[] args) {
String str="So,many,ways,to,reach,delhi,from,hyderabad";
ArrayList<String> Split=new ArrayList<>(Arrays.asList(str.split(",")));
Split.forEach(System.out::println);
}
}
Output
So
Many
Ways
to
reach
Delhi
from
Hyderabad