Collections in Java
Collection framework is one of Java's most powerful subsystems. It is a set of classes and interfaces that is all-the-rage technology for superintending objects and a group of objects. In simple terms; "A collection framework is a container which allows grouping of a number of elements together". It is symbiotic in sorting data and processing it efficiently. Collection Frameworks work as libraries. Collection framework has:
- Interfaces
- Classes to implement the interface.
- Algorithms.
How is Collection Framework different from arrays?
Unlike array;- The size of the collection need not to be declared at the time of instantiation. They can resize themselves with respect to objects automatically.
- Collections cannot hold basic data types.
Operations that can be performed on collection
A list of operations that can be performed on any collection:- Addition of new object.
- Deletion of an existing object.
- Traversing the objects.
- Retrieving desired objects.
- Deleting desired objects.
- Searching a particular object.
Collection-Interface Hierarchy
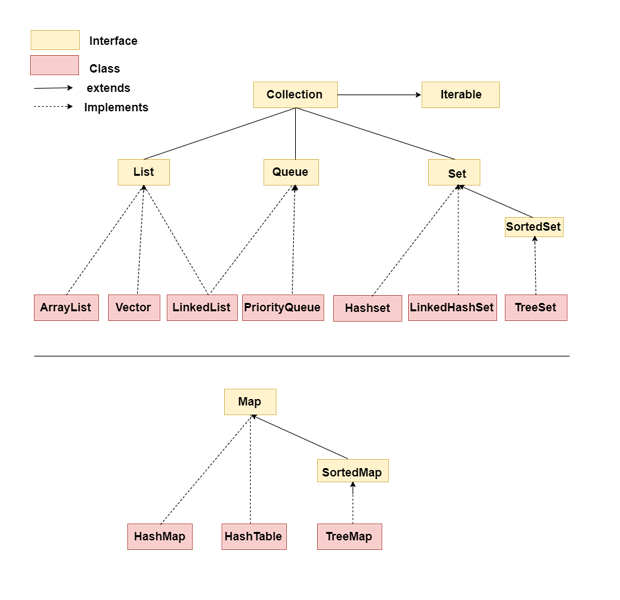
Interfaces in Collection Framework
Collection interfaces determine the fundamental nature of collection classes. There are various interfaces in the collection framework. They are listed below. 1. Collection? It is a generic interface at the top of the collection hierarchy. Every class needs to implement this interface. It enables the users to work with a group of objects. Collection implements the Iterable interface. The general declaration is: interface CollectionE specifies the type of objects that the collection will hold. 2. Queue ? It extends the Collection interface and declares the behavior of a queue. Queue is a data structure that follows the first in first out (FIFO) principle. It is a generic interface. The declaration of queue is: interface QueueE specifies the type of objects that the queue will hold. 3. Dequeue? It extends the Queue interface. Dequeue is a double-ended queue in which the insertion and deletion of elements are possible from both the ends of queue. It is a generic interface with the declaration as interface Dequeue.E specifies the type of objects that dequeue will hold. 4. List ? It extends the collection interface and declares a structure to store a sequence of the element. Elements can be accessed, inserted, deleted by specifying their position in the list. It is a generic interface with declaration as interface List.E specifies the type of objects that the list will hold. 5. Set ? It extends the collection interface and declares a set. The set stores the element such that there is no repetition. It is a generic interface with declaration as interface Set.E specifies the type of objects that the set will hold. 6. SortedSet? It extends the set and declares the behavior stored in a set in ascending order. It is a generic interface with declaration as interface SortedSet.E specifies the type of objects that the set will hold. 7. NavigableSet? It extends the SortedSet interface and declared the behavior of elements such that element with the closest match to a given value is retrieved. It is a generic interface with declaration as interface NavigableSet.E specifies the type of objects that the set will hold.Classes in Collection
There are various classes in the Collection. Some classes provide full implementation while the rest provide a skeletal structure. The various classes are discussed below:- AbstractCollection: This class implements most of the interfaces which are defined in the collection.
- AbstractList: This class inherits the AbstractCollection class and implements most of the interfaces in the List Interface.
- AbstractQueue: This class inherits the AbstractCollection class and implements most of the interfaces in the Queue Interface.
- AbstractSequentialList: This class inherits the AbstractList class for use in the files which uses sequential access. Sequential access is the one in which each word is iterated one by one.
- LinkedList: This class inherits the AbstractSequentialList class and implements linked lists.
- ArrayList: This class inherits the AbstractList class and implements a dynamic array. A dynamic array is the one in which the size is declared at run time.
- ArrayDeque: This class inherits the AbstractCollection class and implements the dequeue interface. It implements a double-ended queue dynamic in nature.
- AbstractSet: This class inherits the AbstractCollection class and implements most of the interfaces in the list interface.
- EnumSet: This class inherits the AbstractSet class and uses it with the enum elements.
- HashSet: This class inherits the AbstractSet class and uses it with the hash table.
- LinkedHashSet: This class inherits the HashSet class and allows iterations in the order of insertion.
- PriorityQueue: This class inherits the AbstractQueue class and uses it in the making of priority queues.
- TreeSet: This class inherits the AbstractSet class and uses to make a sorted set in the tree.
Algorithm in Collection
The collection defines several algorithms that can be used with the collection class.Useful methods of collection interface are as follows:
add(): The add() method inserts a new element into the collection and returns whether it was successful.
The method signature is
boolean add(E element)
The collection uses generics “E for Elements”. It means the generic type that was used to create the collection.
Example :
import java.util.ArrayList; import java.util.HashSet; import java.util.List; import java.util.Set; public class ListExample { public static void main(String[] args) { List<String> countries = new ArrayList<>(); // Creating list of System.out.println(countries.add("INDIA")); // will return true. Set<String> countries1 = new HashSet<>(); System.out.println(countries1.add("INDIA")); // will return true System.out.println(countries1.add("INDIA")); // will return false (set does not allows duplicate) } }
Output:
true true false
remove(): The remove() method removes a single matching value in the collection and returns whether it was successful.
The method signature is
boolean remove(Object object)
Example :
import java.util.ArrayList; import java.util.List; public class RemoveExample { public static void main(String[] args) { List<String> countries = new ArrayList<>(); // creating List of countries countries.add("INDIA"); // adding INDIA will return true // [INDIA] countries.add("INDIA"); // adding INDIA again will return true //[INDIA,INDIA] System.out.println(countries .remove("CHINA")); // removing CHINA and will return false System.out.println( countries .remove("INDIA")); // removing INDIA and will return true System.out.println(countries); // will print list of countries ([INDIA]) } }
Output:
false true [INDIA]
isEmpty() : Checks if the collection have elements:
signature: boolean isEmpty()
Example:
import java.util.ArrayList; import java.util.List; public class IsEmptyExample { public static void main(String[] args) { List<String> countries = new ArrayList<>(); System.out.println(countries.isEmpty()); // returns false countries.add("INDIA"); System.out.println(countries.isEmpty()); // returns true } }
Output:
true false
size(): looks how many elements are in the Collection.
Signature is : int size()
Example:
import java.util.ArrayList; import java.util.List; public class SizeExample { public static void main(String[] args) { List<String> countries = new ArrayList<>(); System.out.println(countries.size()); // returns 0 countries.add("INDIA"); System.out.println(countries.size()); // returns 1 } }
Output:
0 1
clear(): The clear() method provides an easy way to discard all elements of the Collection. The method signature is
void clear()
Example:
import java.util.ArrayList; import java.util.List; public class ClearExample { public static void main(String[] args) { List<String> countries = new ArrayList<>(); countries.add("INDIA"); countries.add("CHINA"); System.out.println(countries.size()); // returns 2 countries.clear(); // [] System.out.println(countries.size()); // return 0 } }
Output:
2 0
contains(): The contains() method checks if a certain value is in the Collection.
The method signature is
boolean contains(Object object)
Example:
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<String> countries = new ArrayList<>(); countries.add("INDIA"); System.out.println(countries.contains("INDIA")); // returns true System.out.println(countries.contains("CHINA"));// returns false } }
Output:
true false