Packages in Java
Packages in Java can be defined as an assortment for grouping various classes and interfaces based on their performance. It is a catalog for holding various java files. They provide a very efficient mechanism for alignment of various files. The files which are related to each other can be stored in the same package, and whenever there is a need to access the related files, we just have to go through one package. A unique namespace is provided by the package. A package can contain the following types of file in java:
- Class
- Interface
- Enumerated types
- Annotations
Advantages of packages
- Two classes in two different packages can have the same name so, name collision issue is not a problem.
- Classes related to each other are stored in the same package so, accessing them is easy.
- Access protection is provided by packages so, no need to worry about the security concerns. The files that are crucial can be hidden successfully.
- Programmer can make their own packages as per their convenience. It is programmer friendly.
- Code reusability is provided.
Types of packages in Java
Built-in packages: They are the ones which are predefined by the java compiler. They can be imported to use by the user. User-defined packages: They are the ones which are made by the programmer to group related classes, enumerations, annotations and interfaces as required by him.Built-in packages in java.
There are some built-in packages in java that can be used as it is by the programmer. They are listed below.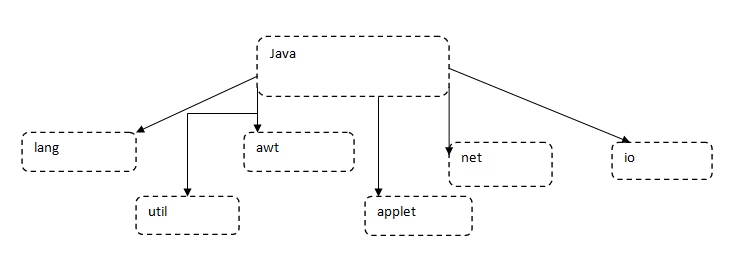
- java.lang: This package contains language support classes. They are by default imported in the java programs.
- java.util: This package contains language utility classes such as vectors, lists, hash tables, etc.
- java.awt: This package contains a set of classes for implementing the graphic user interface.
- java.applet: This package contains a set of classes for using applets in java program.
- java.net: This package contains a set of network classes.
- java.io: This package contains classes for input and output support.
User-defined packages
Having seen the predefined packages, now it's time to see how the packages can be created, imported and used by the users. Creating Packages- Select a suitable name for the package.
- Declare the name of the package with the 'package' keyword in the first line of a program. E.g. package firstpack.
- Define a public class inside that package.
- Create a subdirectory in the main directory to store the class. Save it as classname.java.
- Compile the file.
package packagename; public class classname; { ................. }Importing Packages After the creation of packages, it can be imported using the 'import' keyword. Packages can be imported in two ways.
- By explicitly declaring the class name to be imported: E.g. import firstpackage.secondpackge.classname;
- By importing all the classes in a package. E.g. import packagename.*;
package pkg1; //creating package public class Calculator { int x; int y; public int add(int a,int b) { x=a; y=b; return x+y; } public int sub(int c,int d) { x=c; y=d; return x-y; } public int mul(int e,int f) { x=e; y=f; return x*y; } public int div(int g,int h) { x=g; y=h; return x/y; } }pack1 is the user-defined package, and 'Calculator' is the name of the class. The program is saved with the name Calculator.java. Once defined, let's see how the package is used.
import pkg1.Calculator; //importing package class Operate { public static void main(String args[]) { Calculator obj1 = new Calculator(); obj1.add(5,6); Calculator obj2 = new Calculator(); obj2.sub(8,6); Calculator obj3 = new Calculator(); obj3.mul(5,6); } }
Program to import two packages simultaneously.
package pkg1; public class Calculator { int v=45; public void show() { System.out.println("Hy!! value of v is"+v); } }Now, we will make another class which will import both the packages.
import pkg1.Calculator; import pkg2.ClassA; class Test { public static void main(String args[]) { Calculator obj1 = new Calculator(); obj1.add(5,6); ClassB obj2 = new ClassB(); obj1.show(); } }
This class uses both the packages simultaneously. We can import as many packages as we desire.
How to add a new class to an existing package?
There is an example given below to add a new class to an existing package.package p1; public class One { .............. }This is the package in which we wish to add a new class, and it can be done as:
- Define the class and use a public modifier.
- Place the package statement at the top of the program.
- Compile the class as done earlier.
- The class is now added to the package.
package p1 public class Two { ................ }