Conditional operator in Java
In Java, there are around eight operators, and among them, three operators are used to evaluate the condition and decide the Result based on the Result of the evaluated condition.
Below are the three conditional operators described.
- Conditional AND
- Conditional OR
- Ternary operator
Conditional AND— symbol (&&)
By using AND, the evaluation is done by comparing the two Boolean expressions specified before and after “&&”. The Result will be true only if the return value of both expressions is true. Else false will be returned.
Let's see the truth table of how && will be compared.
True && false = false
False && false = false
False && true= false
True && true = true.
And example syntax:
The explanation for each of the above four conditions:
int a=5, b=5, c=2;
if (a==b && b>c)
{
//logic to be executed
}
In the above example, a==b is true because a=5 and b=5, and b>c is true because 5>2 is true.
So, a combination of true && true will return true, and then the code inside the block will be executed.
int a=5, b=4, c=2;
if (a==b && b>c)
{
//logic to be executed
}
In the above example, a==b is false because a=5 and b=4, and b>c is true because 4>2 is true.
So, a combination of false && true will return false and exits from the block without going into the block of code.
Conditional OR — symbol (||).
The OR is quite the opposite of the AND. Unlike AND, if the result of one among the two expressions is true, then the Result will be true. Only if both the results are false then OR will return false.
Let's see the truth table of how || will be compared.
True && false = true
False && false = false
False && true= true
True && true = true.
OR example syntax:
The explanation for each of the above four conditions:
int a=5, b=5, c=2;
if (a==b && b<c)
{
//logic to be executed
}
In the above example, a==b is true because a=5 and b=5, and b<c is false because 5<2 is false.
So, a combination of true || false will return true, and then the code inside the block will be executed.
False condition example
int a=5, b=4, c=2;
if (a==b && b<c)
{
//logic to be executed
}
In the above example, a==b is false because a=5 and b=4, and b<c is false because 4<2 is false.
So, a combination of false || false will return false and exits from the block without going into the block of code.
Ternary Operator:
The ternary operator is a type of conditional operator. This operator is known as a ternary operator because it employs three operands: a Boolean expression that can be either true or false; the Result when the Boolean expression is true; and the Result when it is false.
The syntax for the ternary operator:
Result_Value = Conditional _ Statement? value1: value2;
Result_value = this variable gets the Value assigned after computational work.
Conditional_ Statement = this part of the ternary operator performs the execution condition and returns Boolean values.
Value1 = this can be an output statement or Value assigned to Result _Value that gets executed when the conditional_ Statement returns actual Boolean Value.
Value2 = this can be another output statement or Value to be assigned to Result _Value that gets executed when the conditional_ Statement returns a false Boolean value.
For Example:
String resvalue = (20 > 12) ? “ True ” : “ False ”;
Benefits of Ternary Operator:
An if-then-else statement can be expressed using the ternary operator as a shortcut. The code is easier to read as a result.
Let's have a look at the use of the subsequent sample programmes.
Example1: ternary operator with a single condition
TernaryDemo.java
import java.io.*;
import java.util.*;
public class TernaryDemo
{
public static void main (String [] args)
{
Scanner scan = new Scanner (System.in);
System.out.println (" enter the value of x ");
int x = scan.nextInt ();
System.out.println(" enter the value of y ");
int y = scan.nextInt ();
String resultValue = (x >= y)?" x is greater than or equal to y ":" x is less than y ";
System.out.println (resultValue);
// it finds outs the greatest of two numbers
}
}
Output:
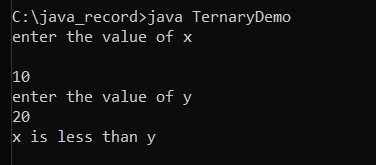
Example2: ternary operator with multiple conditions
TernaryDemo1.java
import java.io.*;
import java.util.*;
public class TernaryDemo
{
public static void main (String [] args)
{
Scanner scan = new Scanner (System.in);
System.out.println (" enter the marks in x ");
int x = scan.nextInt ();
System.out.println (" enter the marks in y ");
int y = scan.nextInt ();
String resultValue = (x >= 80 && y>= 80)?" A grade ":(x >= 60 && y >= 60)?" B Grade ":" Not Eligible ");
System.out.println (resultValue);
// it finds outs the greatest of two numbers
}
}
Output:
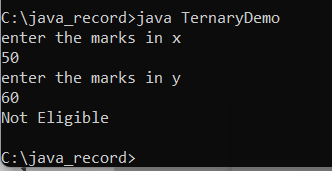