Arithmetic exception in Java
Exception Handling is one of the most potent ways of handling runtime faults and preserving the application's normal flow. In Java, an exception is an out-of-the-ordinary state, and exceptions are defined in the Java programming language. In this part, we will look at one of Java's most well-known exceptions, the Arithmetic Exception.
An arithmetic exception is an unexpected result or unchecked error in the code that is thrown or raised anytime a faulty mathematical or arithmetic operation emerges in the code during run time.
Exception.java
public class Exception {
public static void main(String[] args) {
int a = 0, b = 10;
int C;
System.out.println("Value of C is : "+ b/a);
}
}
Output:
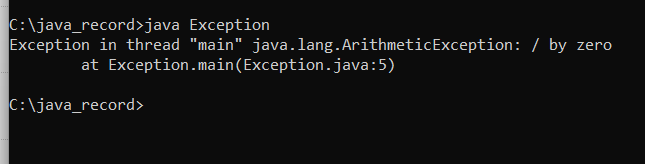
A runtime problem, also known as an exception, occurs when the fraction's denominator is zero, and the JVM cannot determine the result; as a consequence, the program execution is halted, and an exception is reported. It should be noted that the program quits at the point when the exception is triggered. However, the preceding code is performed, and the proper result is printed.
A Java Arithmetic Exception is a type of unchecked error or unexpected result of code that is produced when incorrect arithmetic or mathematical operation occurs in code at run time. When the denominator is integer 0, the JVM is unable to evaluate the result, so the program's execution is halted, and an exception is produced. The program quits at the place where the exception is thrown, but the code preceding that is performed, and the result is shown.
To avoid the ArithmeticException exception in Java, implement arithmetic operations with care and guarantee that they are correct both mathematically and semantically. When found, the Arithmetic Exception error should trigger refactoring of the problematic code, and the exception should be explicitly handled only in rare and warranted circumstances.
Handling Arithmetic Exceptions:
- Try and catch blocks should be used to surround statements that potentially throw ArithmeticException.
- The ArithmeticException can be caught.
- As the execution does not abort, take the essential action for our code.
Exception.java
import java.io.*;
import java.lang.*;
class Exception
{
static int x = 5, y = 0, z;
static int div ()
{
try
{
if (y == 0) // throws an exception
{
throw new ArithmeticException ( " DIVISION BY ZERO "); // throws an exception explicitly
} // if
else
{
return ( x / y );
} // else
} // try
catch (ArithmeticException ae)
{
System.out.println ("ArithmeticException caught at div ( ) ");
throw ae; // rethrows an exception
} // catch
} // div
public static void main (String args [ ] ) throws IOException
{
try
{
int w = div ();
System.out.println (" w = " + w);
try
{
String s1= null;
System.out.println (s1.length ( ) ); // NullPointerException
String s2 = " abc ";
int i = Integer.parseInt (s2); // NumberFormatException
System.out.println (i);
int a [ ]=new int [5];
a [10] = 50; // ArrayIndexOutOfBoundsException
} // inner-try
catch (NullPointerException npe)
{
System.out.println (" NullPointerException at main () = " + npe);
} // catch
catch (NumberFormatException nfe)
{
System.out.println (" NumberFormatException at main () = " + nfe);
}// catch
catch (ArrayIndexOutOfBoundsException aie)
{
System.out.println ("ArrayIndexOutOfBoundsException at main ( ) = " + aie);
} // catch
} // outer-try
catch (ArithmeticException ae)
{
System.out.println (" Exception Recaught at main ( ) = " + ae);
} // catch
finally
{
System.out.println (" CLOSING THE Exception Handling PROGRAM ");
} // finally
} // main
} // ExceptionHandling
Output:
